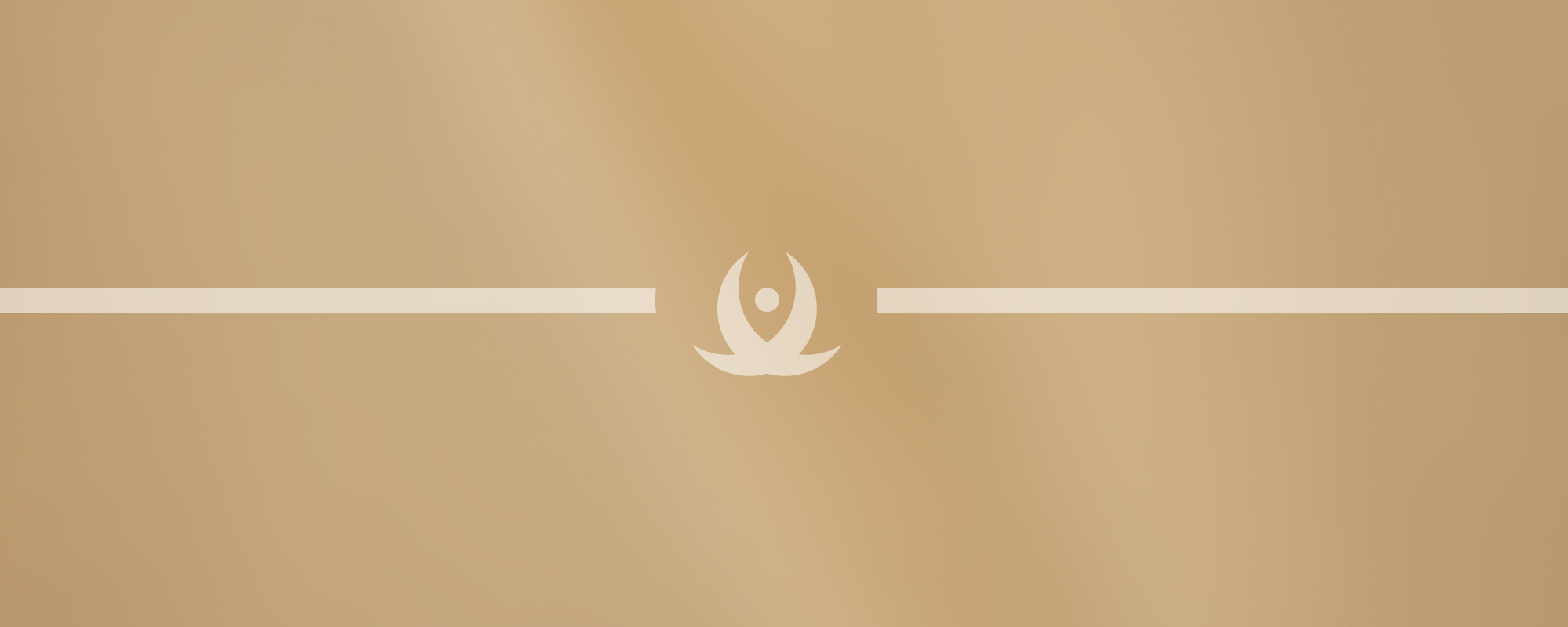
10. Dapper
Dapper Object-Relational Mapping (ORM)
Overview
Dapper is a simple and effective Object-Relational Mapping (ORM) library for .NET. It is among the fastest ORMs due to its minimalistic approach and direct use of SQL queries. Dapper's strength lies in its automatic execution of SQL queries written by the programmer. It is as fast as ADO.NET yet provides a more comfortable programming experience.
Key Features of Dapper
- Dapper is a micro-ORM, meaning it provides minimal functionality while staying lightweight.
- It extends the IDbConnection interface, making it easy to integrate with existing .NET projects.
- Dapper works with any database that has a .NET provider. It can handle both static and dynamic parameters.
Querying with Dapper
Dapper provides a variety of methods to fetch data from a database.
Fetching Multiple Elements
To retrieve multiple elements, we use the Query
method. This method executes a query and maps the result to a strongly typed list.
Example:
using (var connection = new SqlConnection(connectionString))
{
var employees = connection.Query<Employee>("SELECT * FROM Employee").ToList();
}
In this example, Query
executes the SQL query "SELECT * FROM Employee" and maps the result to a list of Employee
objects.
Fetching a Single Element
When fetching a single element (for instance, where id == user_id
), we use the QueryFirstOrDefault
method. This method executes a query and maps the first result to a strongly typed object. If no elements are found, it returns the default value for the type.
Example:
using (var connection = new SqlConnection(connectionString))
{
var employee = connection.QueryFirstOrDefault<Employee>("SELECT * FROM Employee WHERE Id = @Id", new { Id = employeeId });
}
In this example, QueryFirstOrDefault
executes the SQL query "SELECT * FROM Employee WHERE Id = @Id" and maps the first result to an Employee
object. If no results are found, it returns null
.
Conclusion
Dapper is a fast and efficient micro-ORM that is ideal for developers who value the control and simplicity of writing SQL, while also benefiting from the comfort and convenience of an ORM. It's a great choice for applications where performance is critical, and the database schema is already well-defined.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version