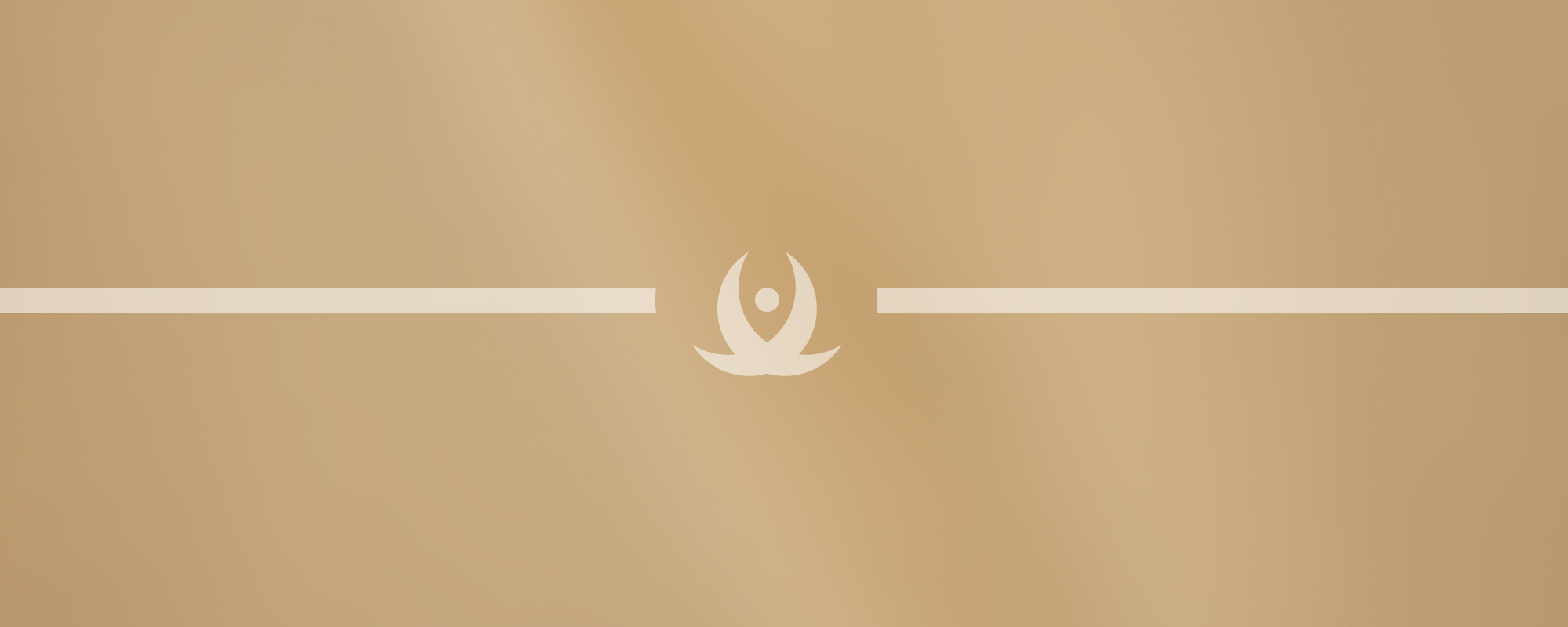
3. Transactions
Transactions in Programming
Overview
A transaction in the context of programming is a sequence of operations performed as a single logical unit of work. A logical unit of work must exhibit four properties, referred to as the atomicity, consistency, isolation, and durability (ACID) properties, to qualify as a transaction.
Callback Function
A callback function is a function that is passed as an argument to another function, to be "called back" at a later time. Callback functions enhance the flexibility and extensibility of our code and are especially critical in handling asynchronous operations such as transactions.
Key Points:
- Callback functions are executed at the end of the current operation.
- They can be used to ensure the sequence of operations (e.g., in a transaction).
Example:
function performTransaction(data, callback) {
// transaction logic here
...
callback(result);
}
performTransaction(data, function(result) {
console.log("Transaction completed. Result: ", result);
});
Wait Handle
A Wait Handle is a programming concept used to block a thread until a particular condition is met. It is an efficient means for threads to wait for a specific event to occur.
Key Points:
- Wait handles pause the execution of the program until a signal is received.
- You can set a limit on how long the program should wait for the signal before proceeding.
Example:
public class Transaction
{
private static AutoResetEvent waitHandle = new AutoResetEvent(false);
public void PerformTransaction()
{
// transaction logic here
...
waitHandle.WaitOne(TimeSpan.FromSeconds(5));
}
public void SignalCompletion()
{
waitHandle.Set();
}
}
In the above example, the PerfomTransaction
method waits for up to 5 seconds for the transaction to complete. The SignalCompletion
method signals that the transaction has completed. If the signal is not received within 5 seconds, the PerformTransaction
method will proceed.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version