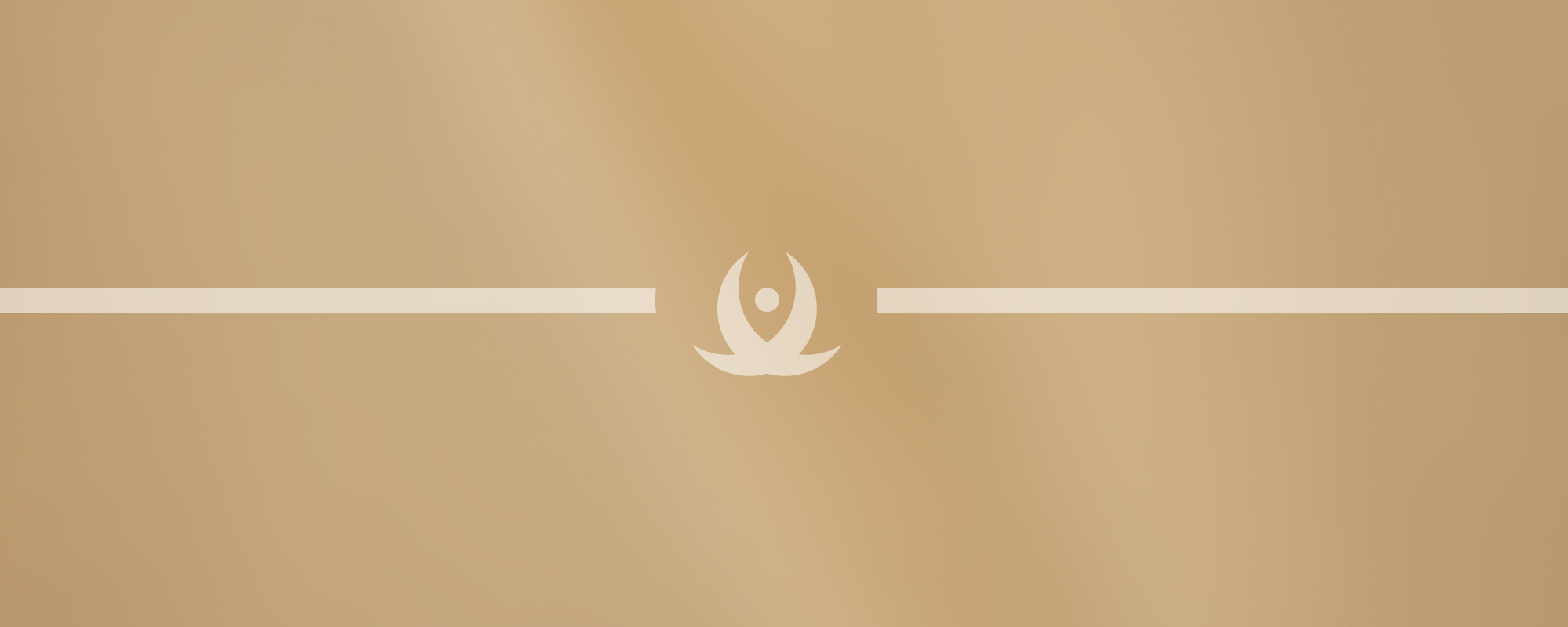
4. Cancellation Token, LINQ to SQL
Cancellation Token, LINQ to SQL
This note provides comprehensive coverage on the topics of Cancellation Tokens and LINQ to SQL, detailing their uses, relevant definitions, and code examples.
Understanding LINQ to SQL
LINQ to SQL is a component of .NET Framework that provides a runtime infrastructure for managing relational data as objects without losing the ability to query. It translates language-integrated queries in the object model into SQL and sends them for execution to the database.
Creating New Threads
Creating new threads with the Begin
method is considered an outdated practice. The modern approach is to use the await
and async
keywords.
public async Task MyMethodAsync() {
// code here
}
Cancellation Token
A CancellationToken in C# is primarily used to send a cancellation message to the operation. Each CancellationToken is 1MB in size and contains a stack.
Thread Execution
Threads within a core operate sequentially. The thread rotation happens every 1/10th over 6 seconds.
Understanding Processes
A running program, such as Google Chrome, is considered a process. For example, when Chrome is open, it is an active process.
DataClasses and LINQ
DataClasses are the heart of LINQ to SQL which automatically generates a object based on our database structure.
INumerable vs IQueryable LINQ
INumerable
fetches all data before filtering, whereas IQueryable
fetches data after applying the filter, making IQueryable
a more efficient method.
IQueryable<YourClass> query = _context.YourClass.Where(x => x.YourProperty == someValue);
DTO - Data to Object
DTO, also known as Data Transfer Object, is a class created to fetch data from a LINQ query. For example, if data about a book is coming and we only need the book name and page number, we create a BookNameDto and fetch data into a BookNameDto object. The result will be IQueryable<BookNameDto>
.
public class BookNameDto
{
public string Name { get; set; }
public int PageCount { get; set; }
}
Navigation Property
In the context of databases, a Navigation Property holds the relational data. For instance, if S_cards holds Student_Id, it will also hold the student object.
InsertOnSubmit and DeleteOnSubmit
These are methods used to insert and delete objects respectively in LINQ to SQL. After modifying the data, we call SubmitChanges()
to save the changes to the database.
// Insert a new object to the database
context.TableName.InsertOnSubmit(obj);
context.SubmitChanges();
// Delete an existing object from the database
context.TableName.DeleteOnSubmit(obj);
context.SubmitChanges();
By understanding these core concepts, one can effectively use LINQ to SQL and Cancellation Tokens within their .NET applications.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version