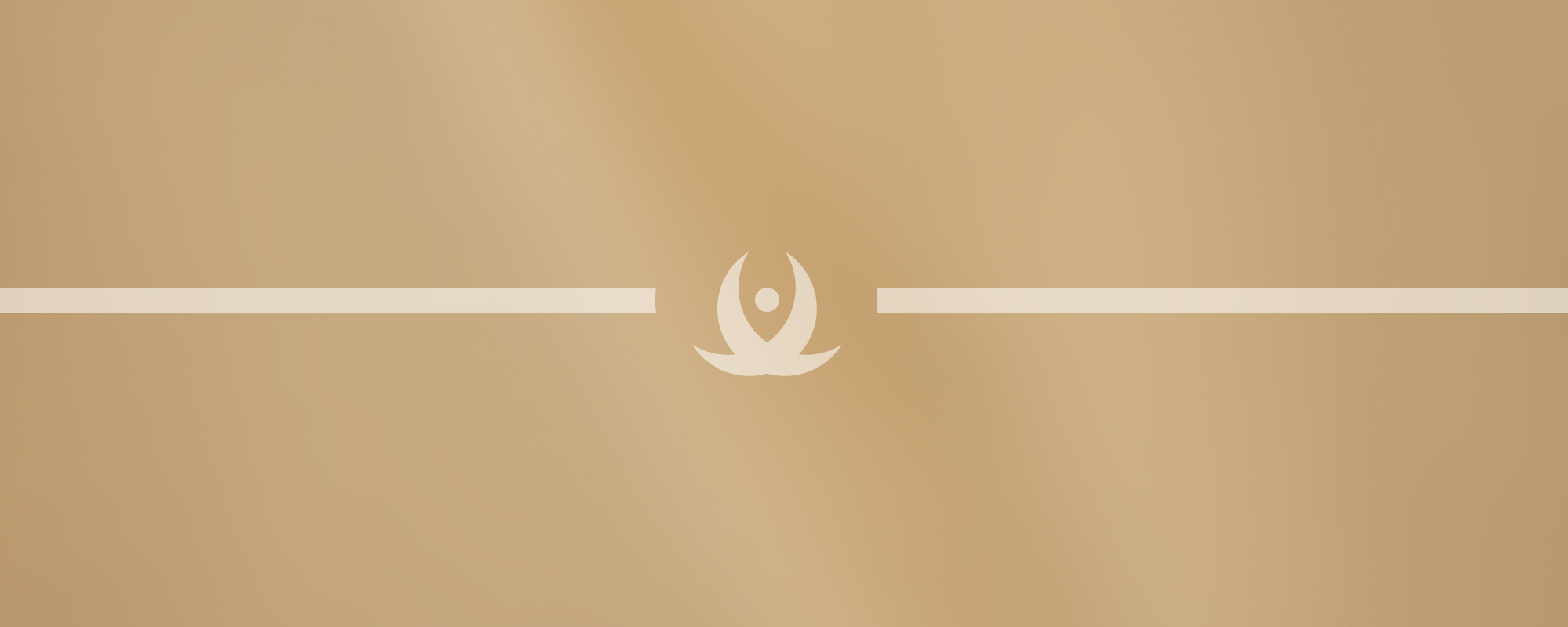
5. LINQ
Language Integrated Query (LINQ) in C#
Language Integrated Query (LINQ) is a powerful feature in C# that allows us to work with data in a more intuitive and readable manner.
Introduction to LINQ
Language Integrated Query or LINQ is a data querying method built directly into C# that allows you to query data from different data sources like collections, databases, XML documents, etc. It provides a standard, easy-to-understand syntax for querying data, and it's integrated directly into C#, which means you can use it anywhere you can write C# code.
Key Points:
- LINQ can be used with a variety of data sources.
- It provides a unified syntax for querying data, regardless of the data source.
- LINQ is built into C#, providing strong typing, IntelliSense support, and other features of the language.
LINQ Query Syntax
Here is an example of a basic LINQ query in C#:
List<int> numbers = new List<int>{ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
var evenNumbers = from num in numbers
where num % 2 == 0
select num;
In this example, evenNumbers
will contain all even numbers from the numbers
list. As you can see, the syntax is similar to SQL and easy to understand.
LINQ Method Syntax
Apart from the query syntax, LINQ also provides a method syntax that is more flexible and powerful. Here is how you can rewrite the above query using method syntax:
List<int> numbers = new List<int>{ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
var evenNumbers = numbers.Where(num => num % 2 == 0);
In this example, evenNumbers
will again contain all even numbers from the numbers
list.
LINQ Operations
There are different types of operations you can perform with LINQ, including:
- Filtering: Selects elements from a collection based on a condition.
- Ordering: Arranges elements of a collection in a particular order.
- Grouping: Groups elements of a collection based on a specified key value.
- Joining: Combines elements from two collections based on a common key value.
Here are some examples of these operations:
Filtering:
var evenNumbers = numbers.Where(num => num % 2 == 0);
Ordering:
var orderedNumbers = numbers.OrderBy(num => num);
Grouping:
var groupedNumbers = numbers.GroupBy(num => num % 2 == 0);
Joining:
var joinedLists = list1.Join(list2, num1 => num1, num2 => num2, (num1, num2) => num1);
Practice with LINQ
It's always a good idea to practice with LINQ queries to get a good grasp of how they work. Try to write queries for different scenarios and experiment with different LINQ methods and operations to see how they work. This will not only help you understand LINQ better, but also make you more comfortable with using it in your code.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version