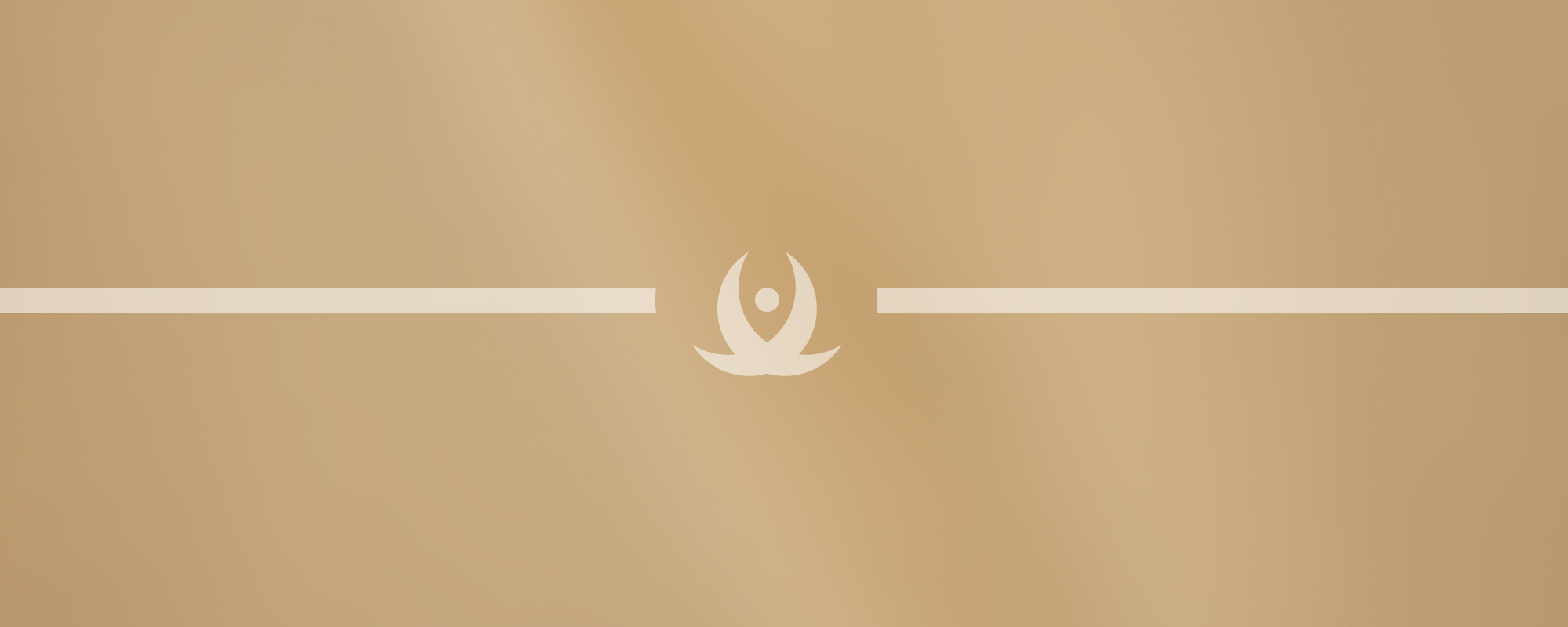
6. Database First Model With Entity Framework
Database First Model with Entity Framework
The Entity Framework is a set of technologies in ADO.NET that support the development of data-oriented software applications. It is an Object/Relational Mapping (ORM) framework that enables developers to work with relational databases in an object-oriented manner.
Introduction to Entity Framework
Entity Framework (EF) is an open-source ORM framework for .NET applications supported by Microsoft. It enables developers to work with data as objects and properties. With EF, you can interact with your database like you would interact with any other .NET objects.
Key Points:
- Entity Framework automatically generates code for the Model (Middle Layer), Data Access Layer, and mapping code, reducing a lot of manual work and resulting in a clean application architecture with less code.
- It provides an abstraction to the relational design pattern and makes the developer's job easier when dealing with the database.
Models in Entity Framework
There are two models in Entity Framework: Database First and Code First.
Database First
Database First is an approach where you first create your database, tables, and relation between them. After that, you create your .NET application that interacts with this database. In this approach, EF creates the context and entities (classes) for the database and its tables that already exist.
Code First
On the other hand, Code First is an approach where you first create your classes in your application. EF then creates the database, tables, and relations based on these classes.
Navigation Properties
Navigation properties are typically defined as virtual so that they can take advantage of certain Entity Framework functionality such as lazy loading. If a navigation property can hold multiple entities, its type must be a list where T is the type of the related entity.
However, sometimes these navigation properties return null. To prevent null values, we can use the include(nameof(Class.NavigationProperty))
to call them.
Here is an example of a navigation property in C#:
public class Blog
{
public int BlogId { get; set; }
public string Name { get; set; }
public virtual List<Post> Posts { get; set; }
}
public class Post
{
public int PostId { get; set; }
public string Title { get; set; }
public string Content { get; set; }
public int BlogId { get; set; }
public virtual Blog Blog { get; set; }
}
In the above example, the Posts
property of a Blog
entity and the Blog
property of a Post
entity are navigation properties. They allow us to navigate from a Blog
to its related Posts
and from a Post
to its related Blog
.
If we want to include the Posts
when querying a Blog
, we can use the Include
method like this:
var blogs = dbContext.Blogs.Include(nameof(Blog.Posts));
This will ensure that the Posts
navigation property is not null when we access it.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version