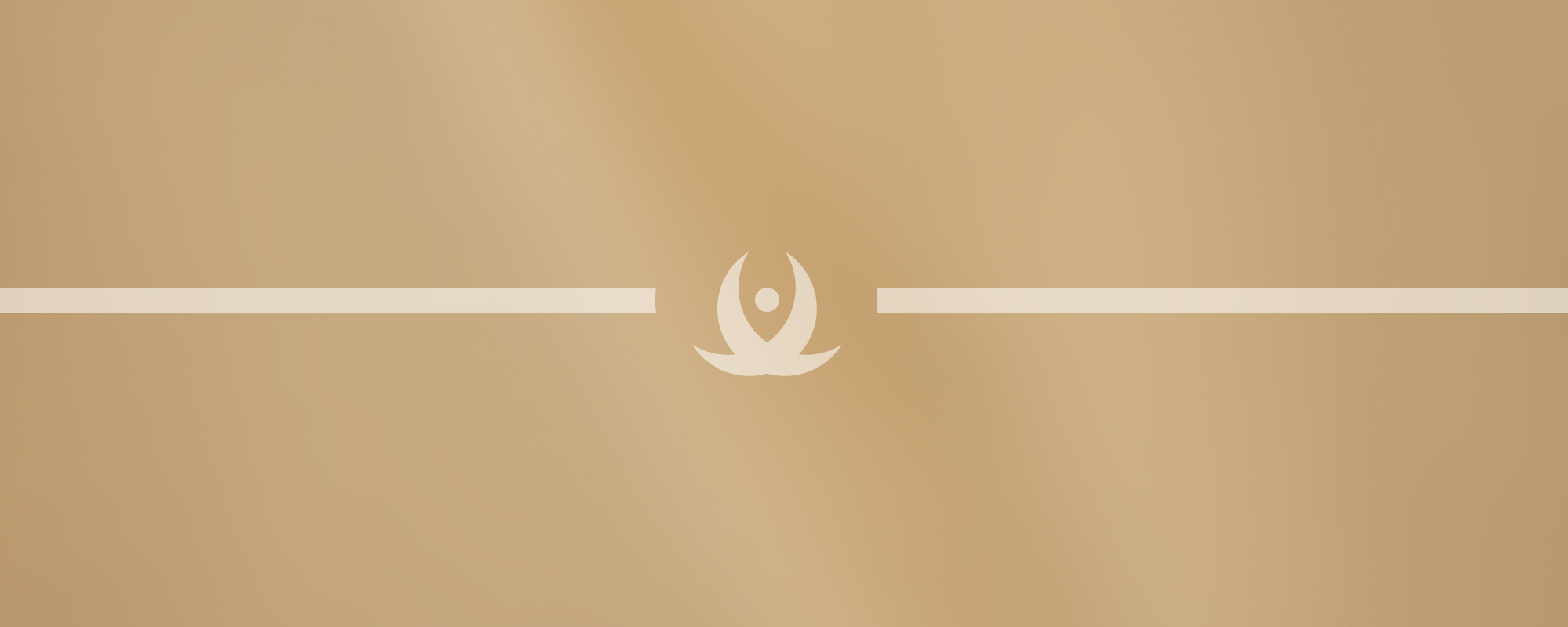
7. Code First Model With Entity Framework
Code First Model With Entity Framework
Introduction
The Code First Model with Entity Framework is a methodology where you write the Classes (Entities) first, and Entity Framework (EF) generates the database from these classes.
Entity Framework and ORM
Entity Framework (EF) is an Object-Relational Mapping (ORM) framework for .NET. It automates database-related activities and enables you to interact with the database using .NET objects.
In EF, Entities are the class equivalent of database tables. They are CLR (Common Language Runtime) classes that EF can track changes to.
Data Annotations
Data Annotations are attributes you can place on a class or property to specify the EF's behavior.
Examples of data annotations include:
[Key]
: This attribute specifies that a property is the primary key of an entity. EF can infer this from property names likeId
or[ClassName]Id
.
[Key]
public int Id { get; set; }
[Required]
: This attribute signifies that the property cannot be null in the database.
[Required]
public string Name { get; set; }
[ForeignKey(Book)]
: This attribute indicates that a property holds a foreign key.
[ForeignKey("Book")]
public int BookId { get; set; }
[Range(10,20)]
: This attribute sets an integer range.
[Range(10,20)]
public int Score { get; set; }
[MaxLength(30, ErrorMessage = "Category name must be 30 or less")]
: This attribute sets a length limit for a string.
[MaxLength(30, ErrorMessage = "Category name must be 30 or less")]
public string CategoryName { get; set; }
int?
: This signifies that an integer property can be null.
public int? OptionalId { get; set; }
Package Manager Console
You can use the Package Manager Console in Visual Studio to execute Entity Framework commands to manage your database schema. These commands include enabling migrations, adding migrations, and updating the database.
Enable-Migrations
: Enables Code First Migrations in a project.
Enable-Migrations
add migration MigrationName
: Adds a new migration with the specified name.
add migration InitialCreate
update-database
: Applies any pending migrations to the database.
update-database
Loading Data
EF provides several ways to load related data.
ToList()
: This method creates a copy of the data in memory.
var books = dbContext.Books.ToList();
LazyLoading
: With Lazy Loading, EF only loads the related data when you access the navigation property. You can control this by setting theLazyLoadingEnabled
property tofalse
ortrue
.
dbContext.Configuration.LazyLoadingEnabled = false;
EagerLoading
: With Eager Loading, you load related data upfront using theInclude
method.
var booksWithAuthors = dbContext.Books.Include(b => b.Author).ToList();
Asynchronous Operations
To perform an operation asynchronously, you can return a Task
. This will run the operation on a background thread.
public async Task<List<Book>> GetBooksAsync()
{
return await dbContext.Books.ToListAsync();
}
Deleting Data
By default, the onDelete
action is set to CASCADE
. This means that deleting a record will also delete its related records in a relationship.
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Book>()
.HasOne(b => b.Author)
.WithMany(a => a.Books)
.OnDelete(DeleteBehavior.Cascade);
}
In conclusion, the Code First approach with Entity Framework provides a powerful way to manage your database schema using .NET classes. It provides several features and tools, including Data Annotations, Package Manager Console Commands, and different ways to load data.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version