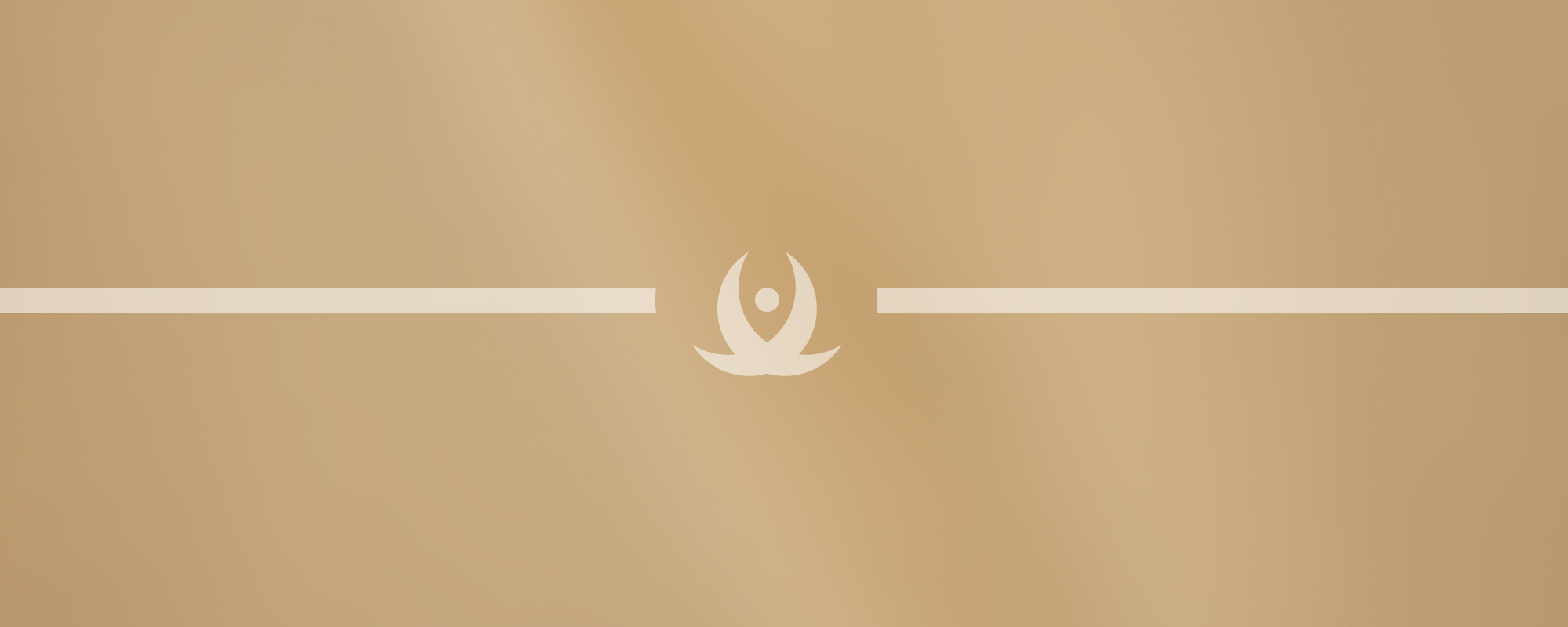
8. Many To Many Relationships
Many To Many Relationships in C#
Overview
In database design, a Many-To-Many relationship refers to a relationship between tables in a database when a parent row in one table contains several child rows in the second table, and vice versa. Many-to-many relationships are often tricky to handle in database schemas.
Understanding Lazy Loading
In C#, the term virtual
in front of properties is used to enable lazy loading. Lazy loading is a concept where we delay the loading of related data until we absolutely need it. This means that the data from the database can be fetched at runtime.
Example:
public class Customer
{
public virtual ICollection<Order> Orders { get; set; }
}
Fluent API & The Unity API
The Fluent API provides a full set of configuration options available in Code-First. This API allows you to configure your model using a fluent API. The Fluent API provides more functionality for configuration than Data Annotations.
The Unity API is a dependency injection container. It is used to create instances of dependencies and inject them across your app.
Mapping
Mapping is a way to define how our entities and their relationships are mapped to a database schema. It provides a more flexible way to handle our database design by writing less code and not messing up the neatness of our codebase.
Example:
public class CustomerMap : EntityTypeConfiguration<Customer>
{
}
EntityTypeConfiguration<Customer>
implies that when a Customer
is created, it should be created according to the values(data annotations) given by this class.
Index Cluster Creation Syntax
The creation of an index cluster involves defining the columns that will make up the index. This is done using the HasColumnAnnotation
method.
Example:
this.Property(t => t.ContactName)
.HasColumnAnnotation(
IndexAnnotation.AnnotationName,
new IndexAnnotation(new IndexAttribute("IX_ContactName", 2) { IsUnique = true }));
In this example, an index cluster is created on the ContactName
column of the table, with a unique constraint indicating that no two rows can have the same value for ContactName
.
Summary
Many-to-Many relationships in C# can be handled using various tools such as Fluent API, Unity API, and Lazy Loading. Understanding these concepts and tools will help you design and implement efficient and flexible database schemas.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version