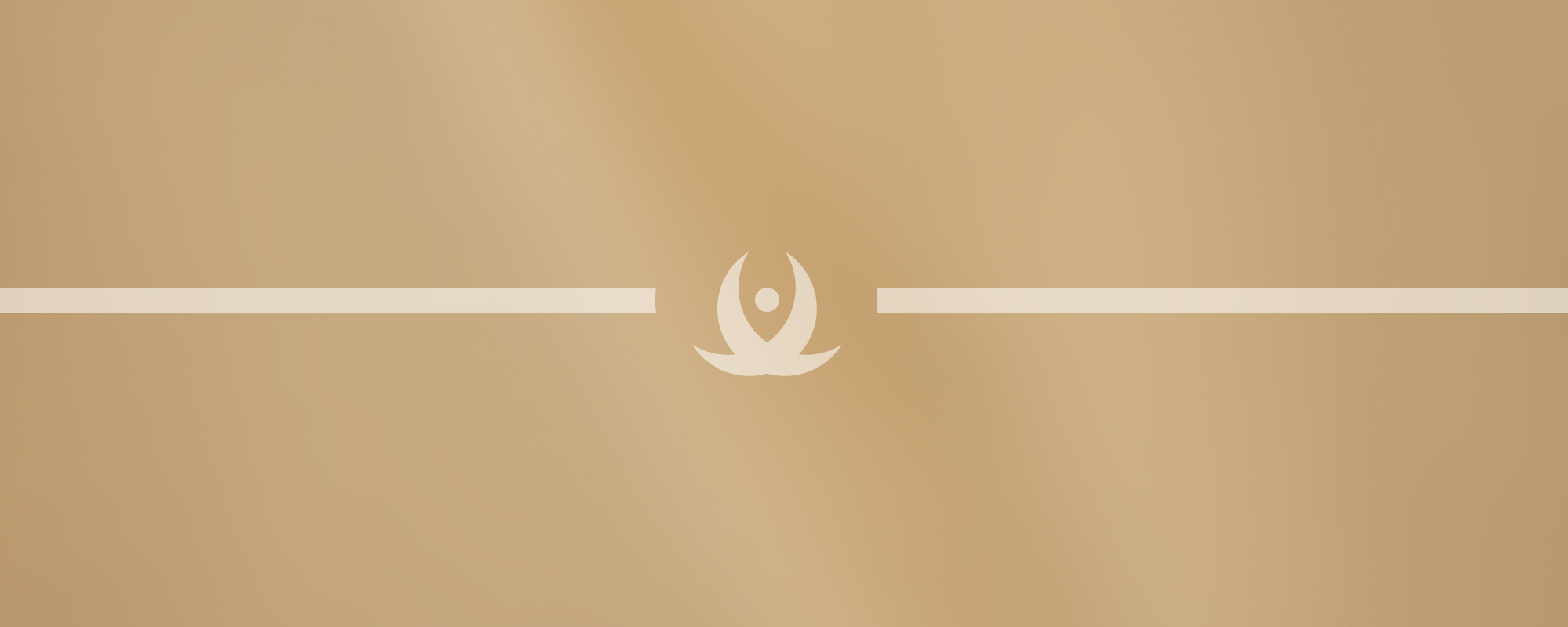
9. FluentApi, IUnitOfWork
FluentApi and IUnitOfWork in Entity Framework
Entity Framework is a powerful Object-Relational Mapping (ORM) tool for .NET applications. It offers several approaches to model and map your application's data structure to a relational database. This lesson will focus on the FluentApi and IUnitOfWork patterns in the Entity Framework.
IUnitOfWork Pattern
The IUnitOfWork is a design pattern that enables you to encapsulate all changes to the objects in a single transaction. This promotes the idea of "committing a unit of work," where you can make changes to your database and then commit all these changes at once or rollback if any error occurs.
Key Points:
- IUnitOfWork is often used in combination with the Repository Pattern.
- It promotes consistency and completeness by ensuring that a transaction is only completed if every operation within it succeeds.
- The IUnitOfWork typically provides a
Save()
orCommit()
method to persist all changes to the database.
FluentApi
FluentApi is a configuration option provided by Entity Framework that allows you to configure your model using C# code, rather than data annotations. FluentApi provides a full set of configuration options, making it a flexible and powerful tool for model configuration.
Key Points:
- FluentApi configurations are specified in the
OnModelCreating()
method in your context class.
- It offers more configuration options compared to data annotations.
- FluentApi configurations have higher precedence over data annotations.
FluentApi Code Example
Here's a code example of how FluentApi can be used in configuring model relationships:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Student>()
.HasOne(s => s.Address)
.WithOne(ad => ad.Student)
.HasForeignKey<StudentAddress>(ad => ad.AddressOfStudentId);
}
In this example, we are configuring a one-to-one relationship between the Student
and StudentAddress
entities.
DbContext and Entity Framework
DbContext is a class provided by Entity Framework that establishes a session with the database and allows querying and saving data. It's a bridge between your domain or entity classes and the database.
Key Points:
- DbContext is used to manage entity objects during runtime, which includes populating objects with data from a database, change tracking, and persisting data to the database.
- DbContext is responsible for the
OnModelCreating()
method where you can override default configurations using FluentApi.
DbContext Code Example
Here's a code example of how DbContext can be used in Entity Framework:
public class SchoolContext : DbContext
{
public SchoolContext(DbContextOptions<SchoolContext> options)
: base(options)
{ }
public DbSet<Student> Students { get; set; }
public DbSet<StudentAddress> StudentAddresses { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Student>()
.HasOne(s => s.Address)
.WithOne(ad => ad.Student)
.HasForeignKey<StudentAddress>(ad => ad.AddressOfStudentId);
}
}
In this DbContext example, we have two DbSets representing Student
and StudentAddress
entities. We also have an OnModelCreating
method where we define the relationship between these entities using FluentApi.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version