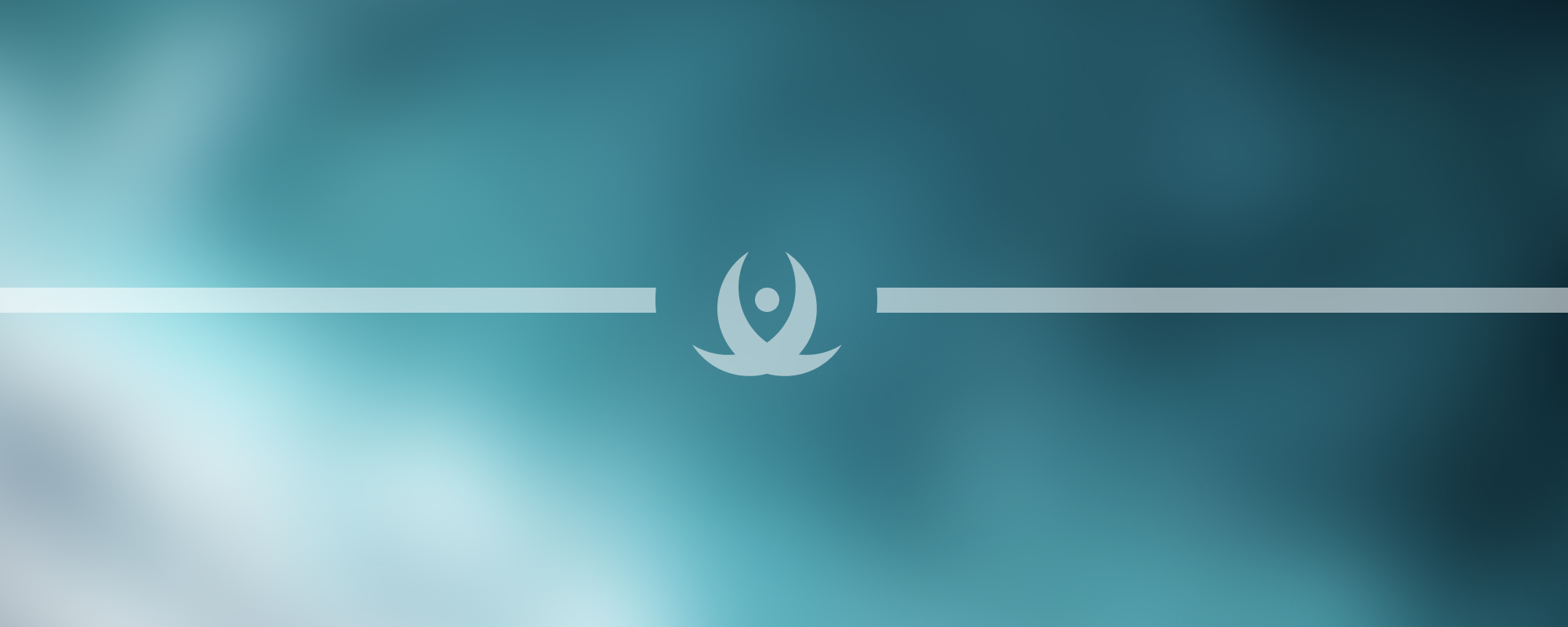
1. Introduction to MVC
Introduction to MVC and ASP
Overview of ASP
ASP, or "Active Server Pages," is a technology developed by Microsoft for building dynamic web pages and web applications. It allows web developers to embed server-side scripting code in HTML pages, which can be executed by the web server before sending the page to the client's web browser. This enables the creation of dynamic content, database-driven websites, and web applications.
<!-- Example of a simple ASP code snippet -->
<%
Dim name
name = "John Doe"
Response.Write("Hello " & name)
%>
ASP operates on a pipeline structure, which includes various middleware components. Each request passes through this middleware, which can provide various functionalities such as authentication.
Overview of MVC
MVC, or "Model-View-Controller," is a design pattern used in software engineering to separate the concerns of an application into three distinct components: the Model, the View, and the Controller.
- The Model represents the application's data and business logic.
- The View represents the user interface.
- The Controller handles user input and manages the interaction between the Model and the View.
In the context of web development, the MVC pattern is often used with frameworks like ASP.NET MVC, Ruby on Rails, and Django to build scalable, maintainable web applications.
For example, in an ASP.NET MVC application, a request like home/GetAllUsers
is processed by the "Home" controller, and the "GetAllUsers" action method is invoked. The Controller then interacts with the Model, obtains the necessary data, and sends it to the View for display.
// Example of a simple Controller in ASP.NET MVC
public class HomeController : Controller
{
public IActionResult GetAllUsers()
{
// Fetch all users from the Model
var users = UserModel.GetAllUsers();
// Pass the data to the View
return View(users);
}
}
Passing Parameters in ASP
In ASP, there are three main ways to pass parameters:
- Route Parameters: Parameters that are part of the URL. For example, in the URL
home/employees/1
, 1 is a route parameter.
- Query Parameters: Parameters that are appended to the URL after a question mark. For example, in the URL
home/employees?key=apple&id=5
,key
andid
are query parameters.
- Form Parameters: When a form is submitted, the values of its input fields are sent to the server as form parameters. In the ASP script, these values can be retrieved using the
Request.Form
collection.
// Example of accessing Form parameters in ASP.NET MVC
[HttpPost]
public ActionResult SubmitForm()
{
string firstName = Request.Form["firstName"];
string lastName = Request.Form["lastName"];
// Process the form data...
return View();
}
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version