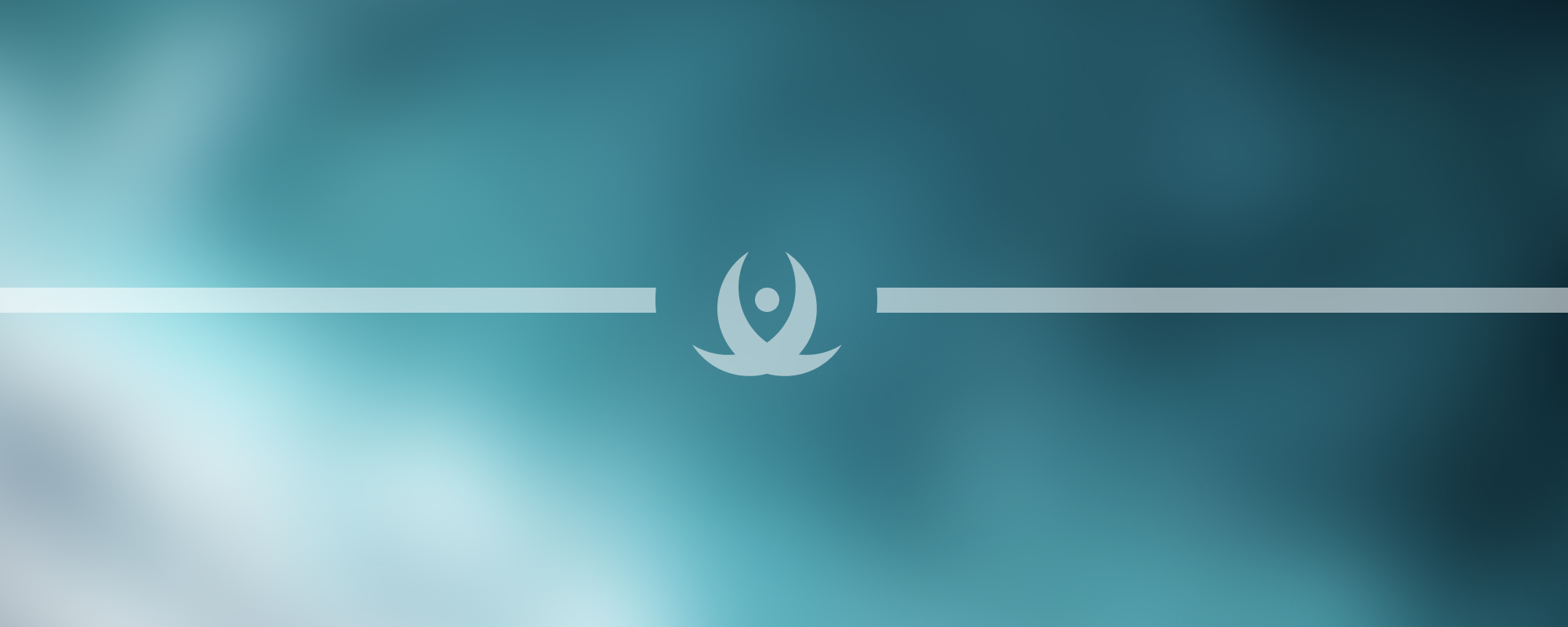
10. Web API
Web API
Introduction
A Web API acts as a gateway or an access card. It is not tied to any specific platform and can receive requests from web, desktop, mobile, etc. It receives requests and finds the action method in the MVC controller (it can fetch data from the database, perform service operations, etc). It then returns the data, which can be in JSON, XML, or any custom format.
Advantages of Web API
One of the key advantages of using a Web API is its portability compared to MVC. This is because it can receive requests from any platform. Web API operates over the HTTP protocol. When an HTTP request arrives from any platform, it sends back an HTTP Response. Majority of the time, the return type is in JSON format (approx. 90%).
Types of Web Services: RESTful and SOAP
Web APIs typically use either RESTful or SOAP web service types for communication between different software applications.
RESTful API
RESTful API (Representational State Transfer) is an architectural style for creating web services. It uses HTTP protocols such as GET, POST, PUT, and DELETE to communicate between client and server. RESTful APIs rely on resources, which are identified by URIs (Uniform Resource Identifiers) and can be manipulated using standard HTTP methods. The data sent and received by RESTful APIs is usually in JSON or XML format. RESTful APIs are widely used for building scalable and lightweight applications and are often used in mobile and web applications.
# Example of RESTful API in Python using Flask
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api', methods=['GET'])
def get_data():
data = {"name": "John", "age": 30}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
SOAP API
SOAP API (Simple Object Access Protocol) is a protocol for exchanging structured information between applications over the internet. SOAP uses XML to encode the messages sent and received and typically uses HTTP or HTTPS for communication. SOAP APIs are typically more complex than RESTful APIs, but offer more features, such as built-in error handling, transaction support, and standardized security protocols. SOAP APIs are commonly used in enterprise applications where reliability and security are important.
// Example of SOAP API in Java
import javax.jws.WebService;
import javax.jws.WebMethod;
@WebService
public class HelloWorld {
private String message = "Hello, ";
@WebMethod
public String sayHello(String name) {
return message + name + ".";
}
}
In summary, RESTful APIs are lightweight, scalable, and flexible, while SOAP APIs are more complex and offer more features, but can be slower and less flexible. The choice between RESTful and SOAP API depends on the specific needs of the application and the level of complexity required for the data exchange.
Data Transfer Objects (DTO)
When returning data in an API, sending unnecessary properties can cause delays in query returns due to the volume of data. Therefore, it is necessary to use Data Transfer Objects (DTOs). DTOs only include necessary properties, which aligns with the principles of Encapsulation.
// Example of DTO in C#
public class UserDTO
{
public string UserName { get; set; }
public string Email { get; set; }
}
In the above example, the UserDTO
class includes only the necessary properties UserName
and Email
. Other properties that are not necessary for the client to know can be excluded from the DTO. This can reduce the amount of data transferred over the network, improving the performance of the API.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version