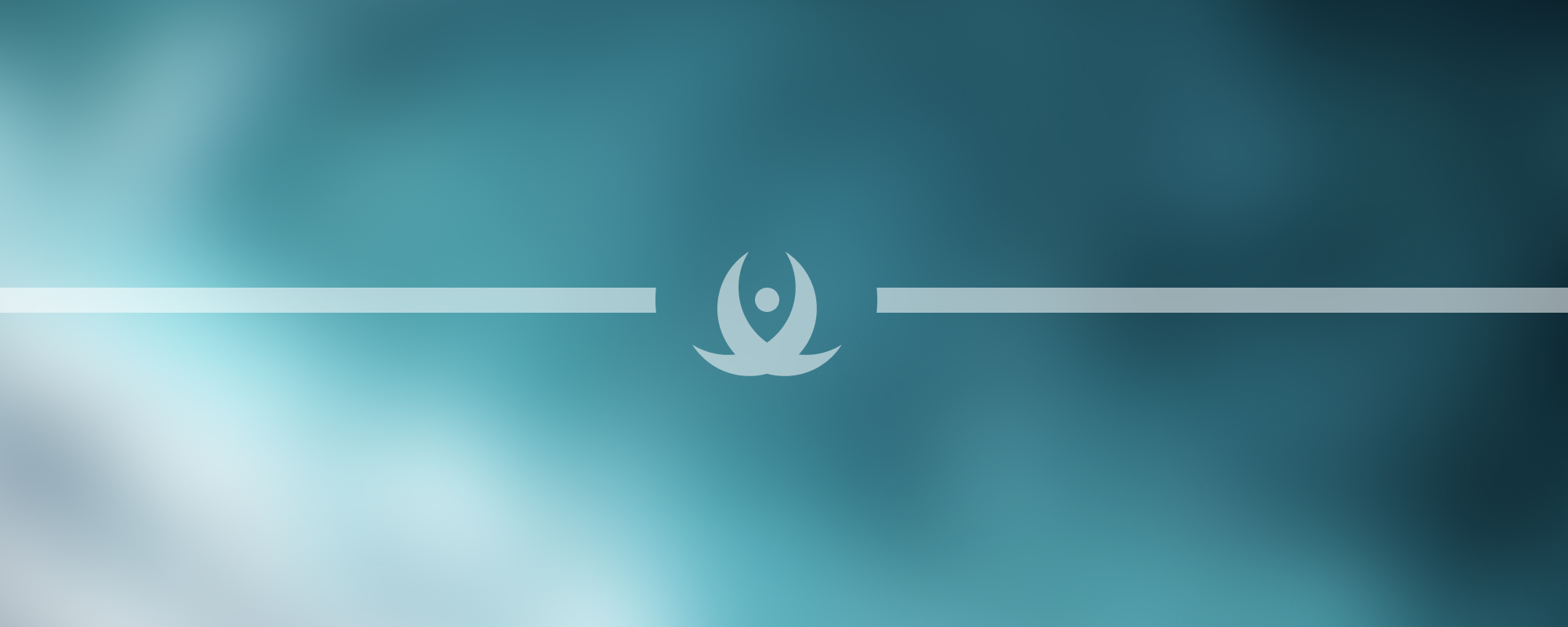
11. Output, Input Formatter
Output and Input Formatters in Software Development
Output and input formatters are pivotal tools used in software development to convert data between diverse formats. These formatters play an integral role in data integration, data exchange, and interoperability between various systems or components.
Definition of Input and Output Formatters
- Input Formatter: An input formatter accepts input data in a specific format and transforms it into a form that the application or system can process. This transformation may involve parsing text, decoding binary data, or extracting relevant information from a complex data structure. The output from the input formatter is usually a standard format that the application can handle.
- Output Formatter: Conversely, an output formatter takes processed data from the application and transforms it into a preferred output format. This transformation could involve formatting the data as plain text, encoding it in a particular binary format, or generating output in a structured format like JSON or XML. The output formatter ensures the data is presented in a manner that is useful and meaningful to the recipient or the subsequent stage in the data processing pipeline.
Role of Input and Output Formatters
Input and output formatters are crucial for compatibility and consistency when working with various data formats. They enable seamless communication and data flow between different software components.
Examples of Input and Output Formatters in C#
Below are some code examples to illustrate the use of input and output formatters in C#.
Input Formatter Example in C#
In this example, we will create a custom JsonInputFormatter
which will handle JSON input with a specific format.
public class CustomJsonInputFormatter : JsonInputFormatter
{
public CustomJsonInputFormatter(ILogger logger, JsonSerializerSettings serializerSettings, ArrayPool<char> charPool, ObjectPoolProvider objectPoolProvider, MvcOptions options, MvcJsonOptions jsonOptions) : base(logger, serializerSettings, charPool, objectPoolProvider, options, jsonOptions)
{
}
public override async Task<InputFormatterResult> ReadRequestBodyAsync(InputFormatterContext context, Encoding encoding)
{
// Custom logic for handling input
}
}
Output Formatter Example in C#
In this example, we will create a custom XmlOutputFormatter
which will format the output data in XML format.
public class CustomXmlOutputFormatter : XmlSerializerOutputFormatter
{
public override void WriteResponseBody(OutputFormatterWriteContext context)
{
var settings = new XmlWriterSettings { Indent = true };
using (var writer = XmlWriter.Create(context.HttpContext.Response.Body, settings))
{
var serializer = new XmlSerializer(context.ObjectType);
serializer.Serialize(writer, context.Object);
}
}
}
In the above examples, the custom input formatter is designed to handle specific JSON input, while the custom output formatter formats the output data in XML format. This showcases how we can transform data to suit our specific requirements in software development.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version