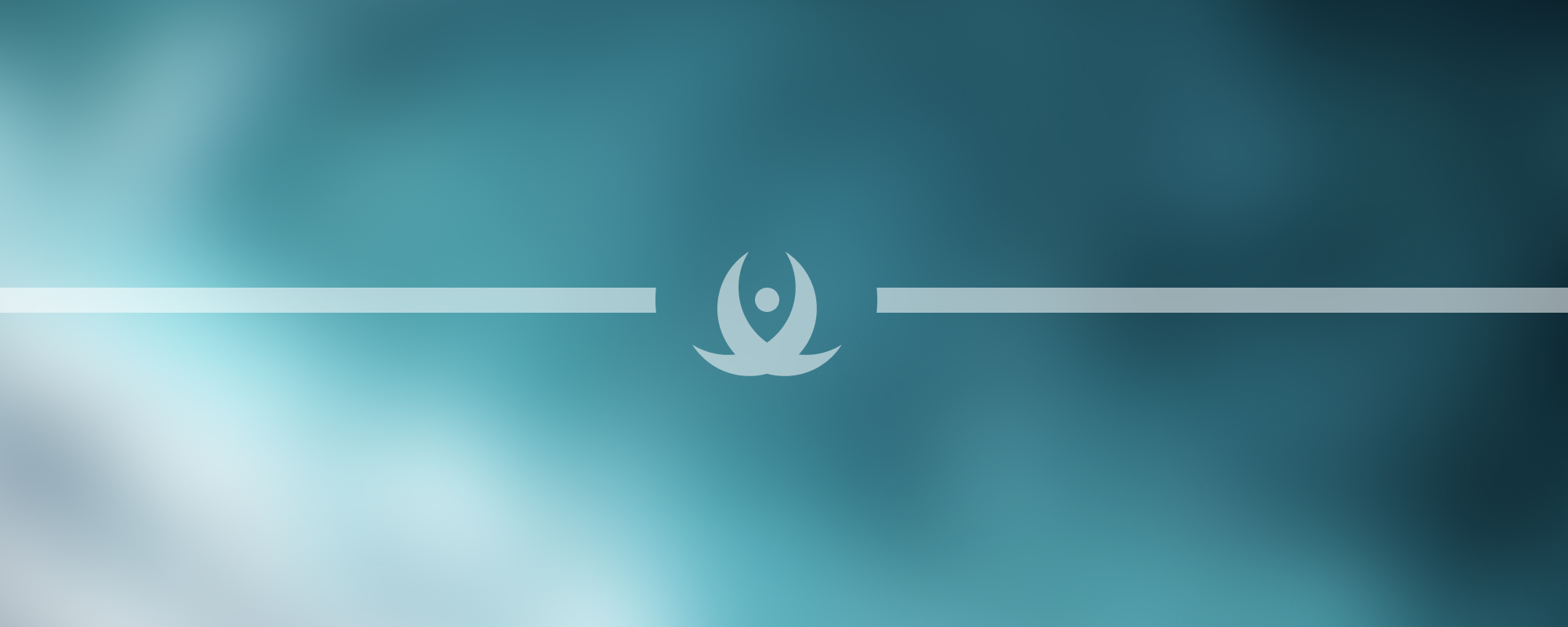
12. Custom Middleware
Custom Middleware in Node.js
What is Middleware?
In the context of Node.js, middleware are functions that have access to the request object (req), the response object (res), and the next function in the application’s request-response cycle. The next
function is a function in the Node.js server's stack that will receive the request and response objects after the current middleware has completed executing.
Middleware functions can perform the following tasks:
- Execute any code.
- Make changes to the request and the response objects.
- End the request-response cycle.
- Call the next middleware in the stack.
Middleware functions are called in the order they are defined. The order is crucial for security, performance, and functionality.
Key Points:
- Middleware can inspect the request and response objects and modify them as needed.
- Middleware can end a request-response cycle by sending a response to the client.
- Middleware can call the next middleware in the stack or skip to error-handling middleware.
Custom Middleware
Custom middleware is a middleware function that we write ourselves to handle specific tasks in our application. We can use it for various purposes, including error handling, logging, body parsing, and much more.
Example:
const myMiddleware = function (req, res, next) {
console.log('Middleware executed!');
next();
};
app.use(myMiddleware);
Middleware Execution During Response
Sometimes, it's necessary to perform certain checks when a response is being prepared. For instance, the validity of a token might expire, or an account password could change. To handle such cases, we can execute middleware when a response is being prepared.
Example:
const tokenCheckMiddleware = function (req, res, next) {
// Perform token validation checks here
if (tokenIsInvalid) {
res.status(401).send('Invalid token');
} else {
next();
}
};
app.use(tokenCheckMiddleware);
In the example above, the tokenCheckMiddleware
function checks the validity of the token in each request. If the token is invalid, it sends a 401 Unauthorized
status and an 'Invalid token' message. If the token is valid, it calls the next
function, allowing the request-response cycle to continue.
Remember to call next()
at the end of your middleware function if the request-response cycle should continue. Otherwise, the request will be left hanging, and the client will not receive any response.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version