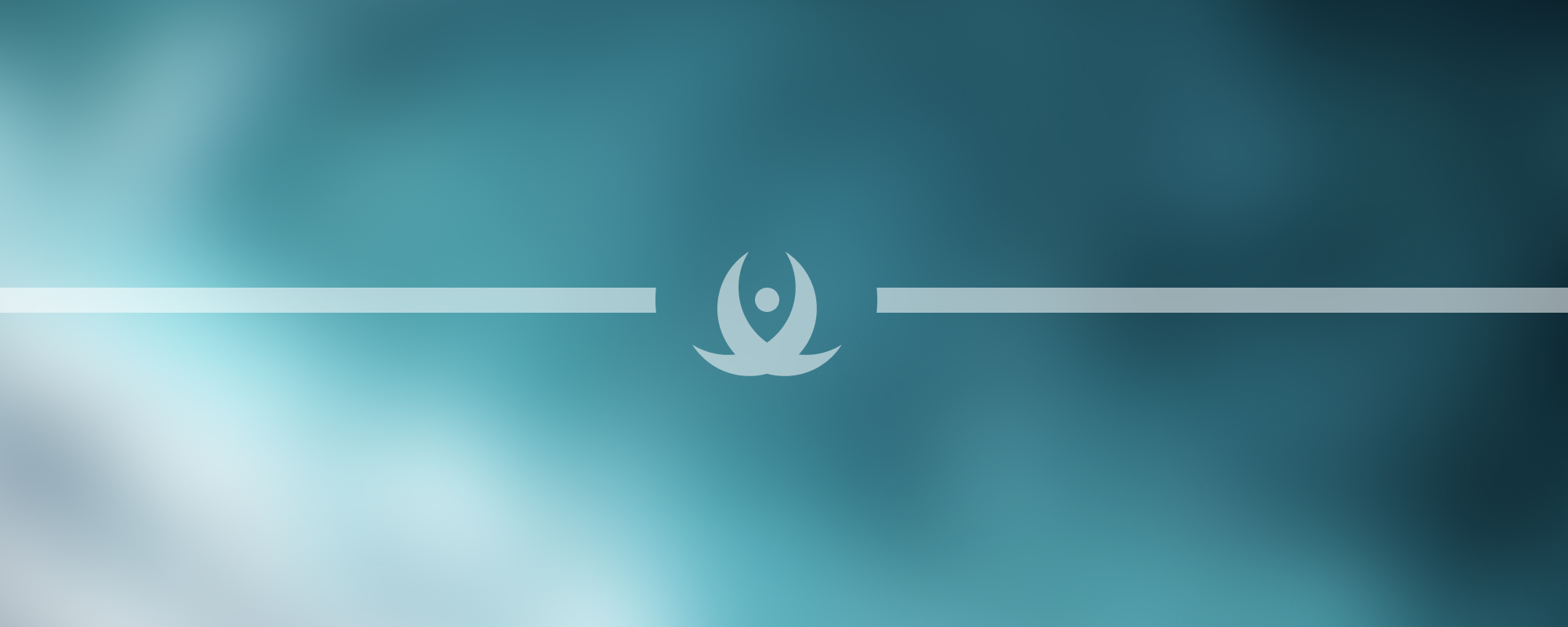
13. City Manager API (I)
City Manager API Using AutoMapper in C#
Introduction
The City Manager API is designed to manage city data. In this lesson, we will be focusing on how to leverage the AutoMapper
library in .NET Core to simplify object-to-object mapping. This is especially useful when properties are numerous and creating DTOs (Data Transfer Objects) manually can become error-prone and cumbersome.
What is AutoMapper?
AutoMapper is a simple, little library built to solve a deceptively complex problem - getting rid of code that mapped one object to another. This type of code is rather tedious and mundane to write, so why not invent a tool to do it for us?
Key Points:
- AutoMapper uses a convention-based matching algorithm to match up source to destination values.
- AutoMapper is designed to provide a way to map your objects, managing the complexity of the mappings so you don't have to.
Installing AutoMapper
To install AutoMapper, we can use NuGet package manager in Visual Studio or run the following command in the Package Manager Console:
Install-Package AutoMapper.Extensions.Microsoft.DependencyInjection
Registering AutoMapper
In order to use AutoMapper, we first need to register it in our Startup.cs file.
builder.Services.AddAutoMapper(typeof(Program).Assembly);
Creating AutoMapper Profiles
An AutoMapper profile is a class where you can define mappings. It extends from the Profile
class in the AutoMapper namespace. Here is an example of an AutoMapper profile for our City Manager API:
using AutoMapper;
using CityManagerApi.Dtos;
using CityManagerApi.Models;
namespace CityManagerApi.Helpers
{
public class AutoMapperProfiles : Profile
{
public AutoMapperProfiles()
{
CreateMap<City, CityForListDto>()
.ForMember(dest => dest.PhotoUrl, option =>
{
option.MapFrom(src => src.Photos.First(p => p.IsMain).Url);
});
CreateMap<City, CityForListDto>();
}
}
}
In the AutoMapperProfiles
constructor, we define two mappings from City
to CityForListDto
. In the first mapping, we also specify how to map the PhotoUrl
property.
Using AutoMapper in Controllers
To use AutoMapper in a controller, we inject it via the constructor. We can then use the _mapper.Map<>
method to map our source object to our target DTO. Here's how we can use AutoMapper in our CitiesController
:
private IMapper _mapper;
public CitiesController(IMapper mapper)
{
_mapper = mapper;
}
[HttpGet]
public IActionResult GetCities()
{
var cities = _appRepository.GetCities(1);
var citiesToReturn = _mapper.Map<List<CityForListDto>>(cities);
return Ok(citiesToReturn);
}
In the GetCities
method, we get a list of cities from the repository. We then use AutoMapper to map the list of City
objects to a list of CityForListDto
objects. Finally, we return the mapped list of DTOs.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version