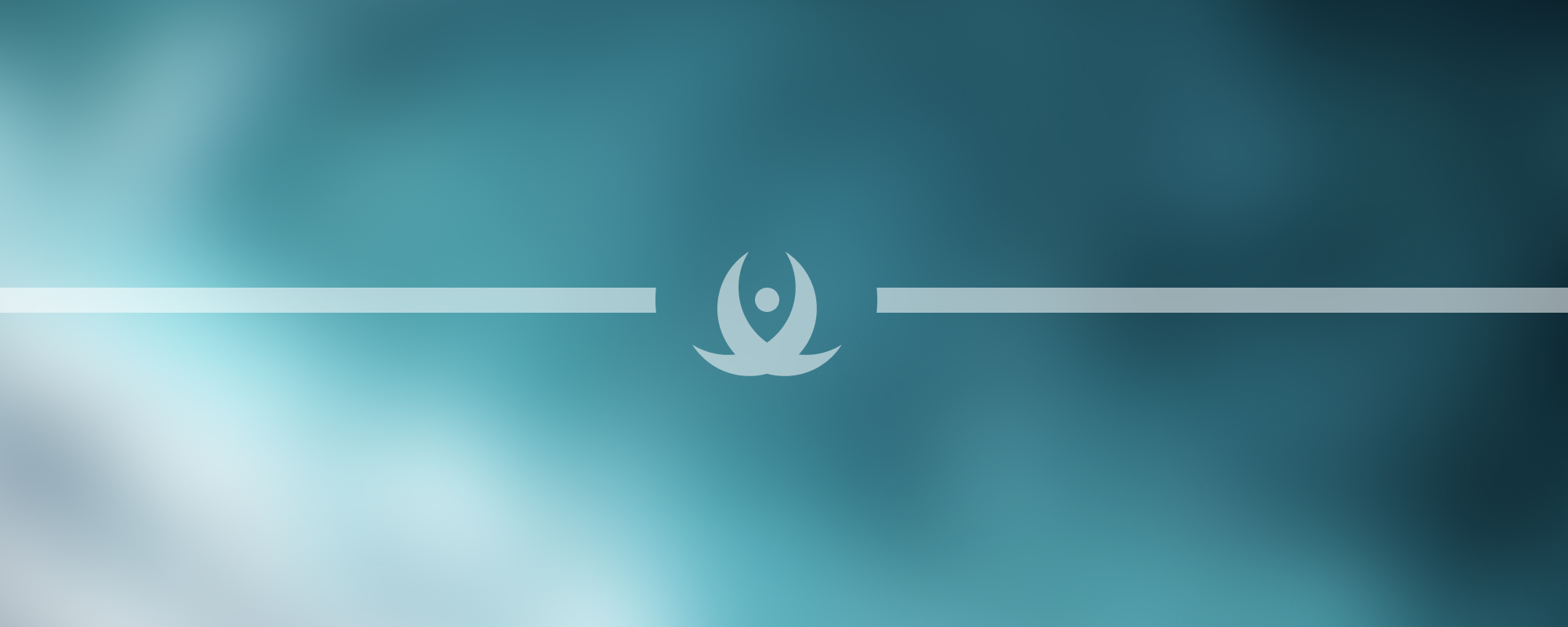
14. City Manager API (II)
City Manager API
The City Manager API lesson notes focus on creating an authentication API for a city management system. The API uses the Repository Pattern, Entity Framework Core, JWT authentication, and .NET Core Identity for user management and authentication.
IAuthRepository
The IAuthRepository
interface defines the contract for user authentication operations. It includes methods for registering a new user, logging in an existing user, and checking if a user exists.
using CityManagerApi.Models;
namespace CityManagerApi.Data
{
public interface IAuthRepository
{
Task<User> Register(User user, string password);
Task<User> Login(string username, string password);
Task<bool> UserExists(string username);
}
}
AuthRepository
The AuthRepository
class implements the IAuthRepository
interface. It uses Entity Framework Core to interact with the database, and .NET Core Identity to manage users and securely hash passwords.
using CityManagerApi.Models;
using Microsoft.EntityFrameworkCore;
namespace CityManagerApi.Data
{
public class AuthRepository : IAuthRepository
{
private DataContext _context;
...
}
}
Login Method
The Login
method attempts to find a user by username. If the user is not found or the password does not match, it returns null
. If the user exists and the password matches, it returns the user.
public async Task<User> Login(string username, string password)
{
...
}
Register Method
The Register
method creates a new user, hashes their password, and saves them to the database.
public async Task<User> Register(User user, string password)
{
...
}
UserExists Method
The UserExists
method checks if a given username exists in the database.
public async Task<bool> UserExists(string username)
{
...
}
JWT Authentication
JWT Authentication is configured in Startup.cs
. The API uses JWT Bearer tokens for authentication.
var key = Encoding.ASCII.GetBytes(builder.Configuration.GetSection("AppSettings:Token").Value);
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters()
{
ValidateIssuerSigningKey = true,
IssuerSigningKey = new SymmetricSecurityKey(key),
ValidateIssuer = false,
ValidateAudience = false
};
});
app.UseAuthentication();
app.UseAuthorization();
Login with JWT Token
The Login
action in the AuthController
handles user login. It checks the provided credentials, creates a JWT token for the user if the credentials are valid, and returns the token.
[HttpPost]
public async Task<IActionResult> Login([FromBody] UserForLoginDto dto)
{
...
}
This token is then used for authenticating subsequent requests by the user. The token includes claims about the user like the user ID and username, and is signed using a secret key.
The City Manager API notes provide a starting point for building a secure API with user registration and login functionality. They demonstrate how to use JWT authentication in a .NET Core API, and how to implement user management with Entity Framework Core and .NET Core Identity.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version