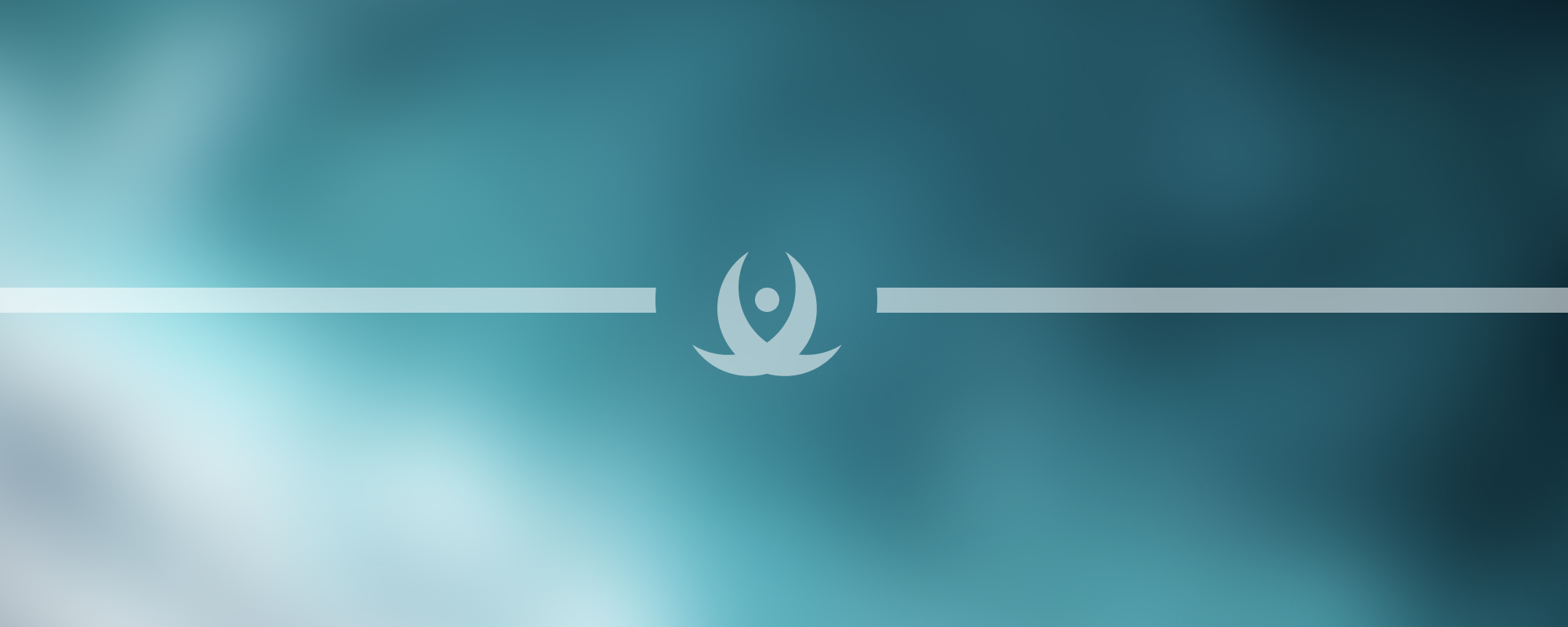
16. SignalR
SignalR in .NET
Introduction to SignalR
SignalR is a software library developed by Microsoft for .NET developers that simplifies the process of adding real-time web functionality to applications. Real-time web functionality is the ability to have server-side code push content to the connected clients as it happens, in real-time.
Key Points:
- SignalR provides a simple API for creating server-to-client remote procedure calls (RPC) that call JavaScript functions in client browsers from server-side .NET code.
- SignalR also includes API for connection management (for instance, connect and disconnect events), grouping connections, and authorization.
SignalR Hubs
- A Hub is a high-level pipeline that allows a client and server to call methods on each other directly.
- SignalR handles the dispatching across machine and process boundaries automatically, allowing clients to call methods on the server as easily as server to client.
Implementing SignalR Hubs
Here is a simple example of a SignalR Hub:
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
}
In this example, the ChatHub
class inherits from the Microsoft.AspNetCore.SignalR.Hub
class. The SendMessage
method can be called from a client to send a message to all clients.
SignalR Transports
SignalR uses the WebSocket transport where available, and falls back to older transports where necessary. While this process is generally transparent, understanding transports can be helpful if you encounter problems or need advanced functionality.
Key Points:
- SignalR will use WebSockets where it is available, and fall back to other techniques and technologies when it isn't.
- Older transports might be less efficient and more resource-intensive, especially on the server.
- SignalR will automatically select the best transport based on the client and server's capabilities.
Client-Side Usage of SignalR
On the client side, you can use the signalr
package provided by Microsoft. Below is an example of how to establish a connection to a SignalR hub and call server-side methods using JavaScript.
const connection = new signalR.HubConnectionBuilder()
.withUrl("/chatHub")
.build();
connection.on("ReceiveMessage", (user, message) => {
console.log(`${user} says ${message}`);
});
connection.start().catch(err => console.error(err.toString()));
In this example, the HubConnectionBuilder
is used to create a connection to the /chatHub
endpoint, and the start
method is called to establish the connection. The on
method is used to define a callback that the server can call.
Conclusion
SignalR is a powerful library that simplifies the process of adding real-time web functionality to your applications. It allows for easy RPCs between client and server, handles connection management, and gracefully falls back to older technologies when necessary. By understanding the basic concepts and capabilities of SignalR, you can start adding real-time features to your own applications.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version