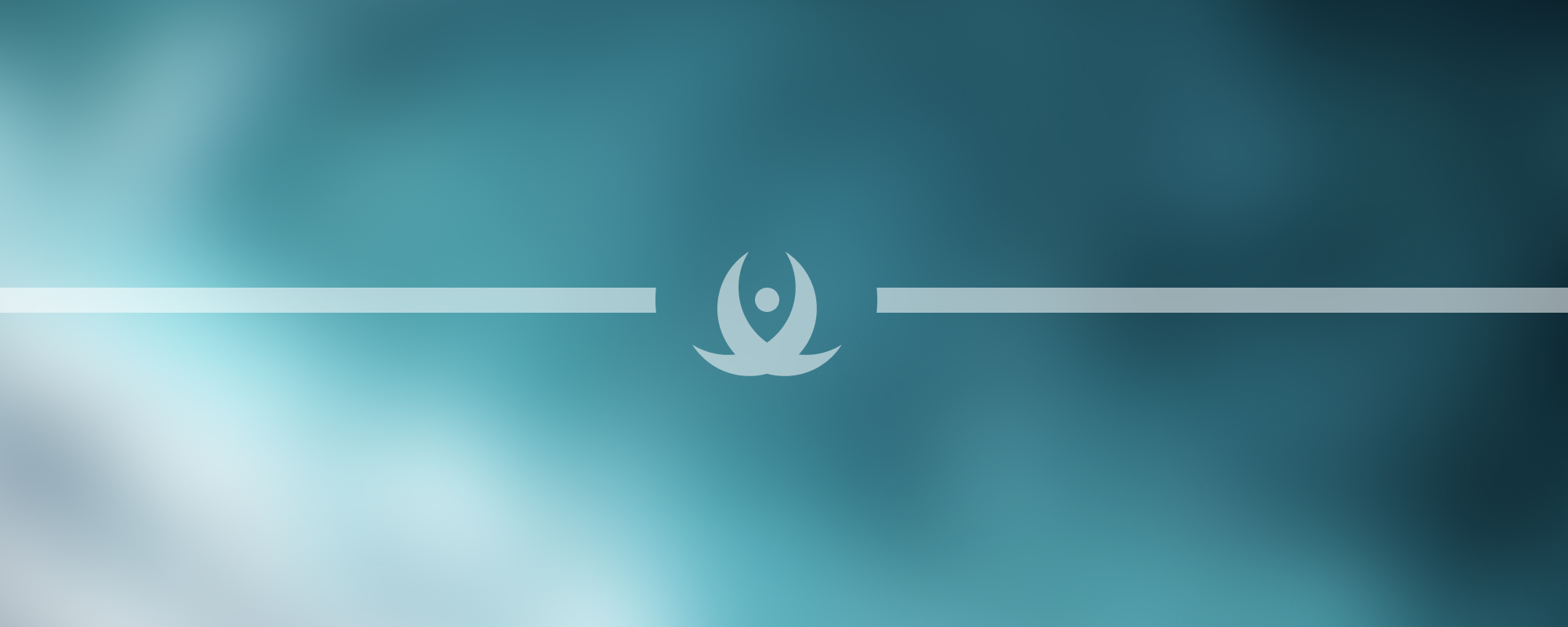
2. Layout, HTTP GET, POST, ASP Taghelpers
Layout, HTTP GET, POST, and ASP Tag Helpers
HTTP GET and POST Methods
Basics of HTTP Methods
HTTP (Hypertext Transfer Protocol) is a protocol that enables communication between clients and servers. In this context, HTTP methods such as GET and POST are used to interact with the server using requests.
HTTP GET Method
The HTTP GET method is utilized to retrieve or read data from the server. The request data is appended to the URL, and thus, it is not suitable for sensitive information.
Example of HTTP GET Usage in ASP.NET:
[HttpGet]
public IActionResult Index()
{
return View();
}
HTTP POST Method
The HTTP POST method is used to send data to the server. Unlike the GET method, the data is sent within the body of the HTTP request, making it more secure and suitable for transmitting sensitive information such as passwords.
Example of HTTP POST Usage in ASP.NET:
[HttpPost]
public IActionResult Create(Employee employee)
{
if (ModelState.IsValid)
{
_db.Add(employee);
_db.SaveChanges();
return RedirectToAction("Index");
}
return View(employee);
}
ASP Tag Helpers
What are Tag Helpers?
Tag Helpers in ASP.NET Core MVC allow server-side code to participate in creating and rendering HTML elements in Razor files. They can be used for various tasks, such as conditionally rendering HTML, creating forms, etc.
Binding with Tag Helpers
To bind a model's property to a form field, you can use Tag Helpers. This allows the value of the form field to be automatically populated with the corresponding model property value and vice versa.
Example of Tag Helper Usage in ASP.NET:
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
<section>
<label asp-for="Employee.Id"></label>
<input asp-for="Employee.Id"/>
</section>
In the example above, the asp-for
attribute specifies the model property to bind the form field to. When the form is submitted, the value entered in the input field is automatically assigned to the Employee.Id
property.
Summary
In this lesson, we covered the basics of HTTP GET and POST methods used in ASP.NET. We also discussed ASP Tag Helpers and how they can be used for model binding in forms. Both these concepts are fundamental in creating interactive web applications using ASP.NET Core MVC.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version