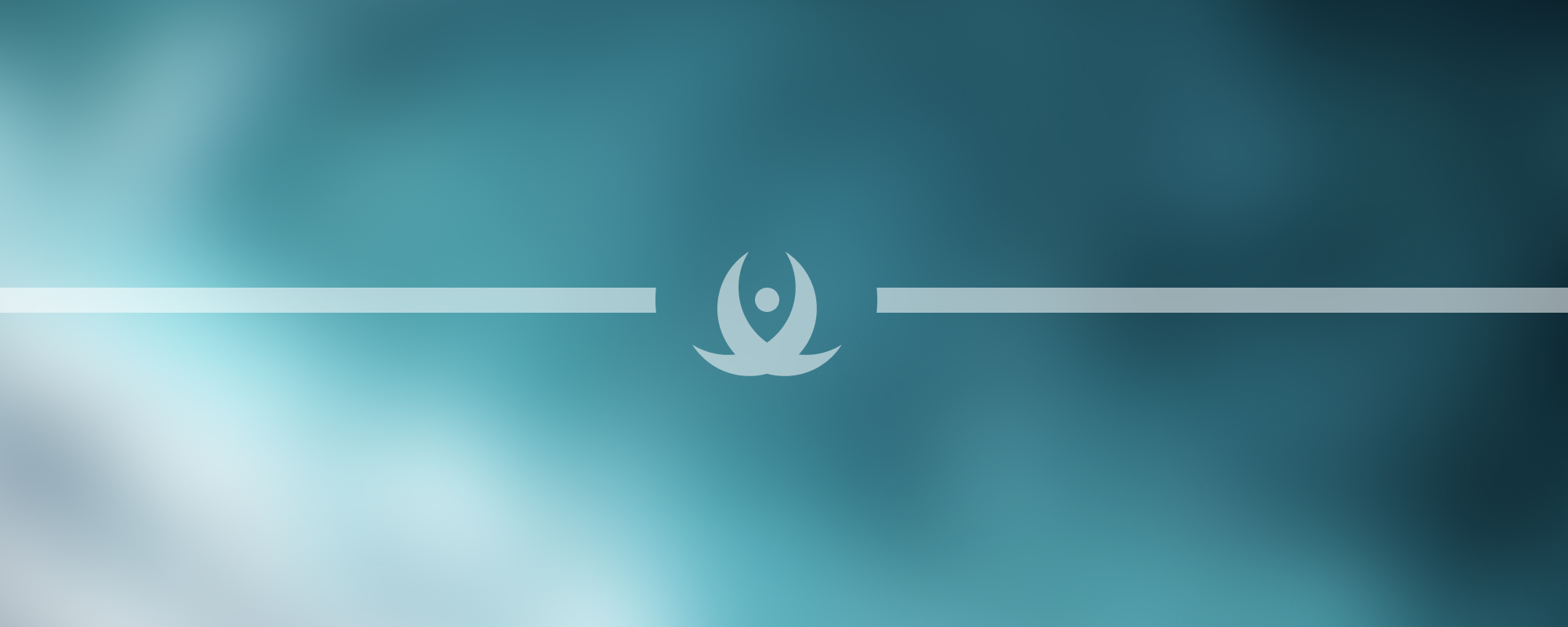
28. Microservices, Monolith Structure (IV)
Microservices and Monolithic Architecture
In this note, we will cover two critical architectural styles used in software development: Microservices and Monolithic structure. We will also delve into different approaches for communication between services in a Microservices architecture, including Https Request, GRPC integration, Stream, and Message Broker.
Microservices Architecture
Microservices architecture is a design pattern where an application is created as a collection of small autonomous services, each running in its own process and communicating with lightweight protocols.
Communication between Services in Microservices
The components in a Microservices architecture interact with each other through various mechanisms. Here are a few common approaches:
- Https Request
- GRPC Integration
- Stream
- Message Broker
Https Request
In an Https request, services communicate with each other using the HTTP protocol over a secure SSL connection. This method is straightforward and widely used. The services send requests to each other and wait for responses.
Here's a basic example of an HTTP request using Python's requests library:
import requests
response = requests.get('<https://api.github.com>')
print(response.status_code)
print(response.json())
GRPC Integration
GRPC is a high-performance, open-source universal RPC framework. It uses Protocol Buffers (protobuf) as its interface definition language, allowing you to define services and message types in .proto files. This integration allows for more efficient communication between services.
Here's an example of a simple service defined in a .proto file:
syntax = "proto3";
service MyService {
rpc MyMethod (MyRequest) returns (MyResponse);
}
message MyRequest {
string request_string = 1;
}
message MyResponse {
string response_string = 1;
}
Stream
Stream communication is an older variant used in Microservices. Services send and receive data as continuous streams.
Node.js example of reading from a stream:
const fs = require('fs');
let readableStream = fs.createReadStream('file.txt');
let data = '';
readableStream.on('data', function(chunk) {
data+=chunk;
});
readableStream.on('end', function() {
console.log(data);
});
Message Broker
A Message Broker is an architectural pattern for message validation, transformation, and routing. It mediates communication among applications, minimizing the mutual awareness that applications should have of each other to exchange messages.
Example of publishing a message to RabbitMQ message broker in Python:
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='hello')
channel.basic_publish(exchange='', routing_key='hello', body='Hello World!')
print(" [x] Sent 'Hello World!'")
connection.close()
Monolithic Architecture
A monolithic architecture is a software development pattern where an application is built as a single, autonomous unit. This unit has one codebase, which is divided into different functional components, such as the database, user interface, server-side application, etc. Monolithic architecture is simple to develop, test, and deploy, making it a good choice for small-scale applications.
In the next note, we will explore the advantages and disadvantages of both Microservices and Monolithic architectures, and when to use each.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version