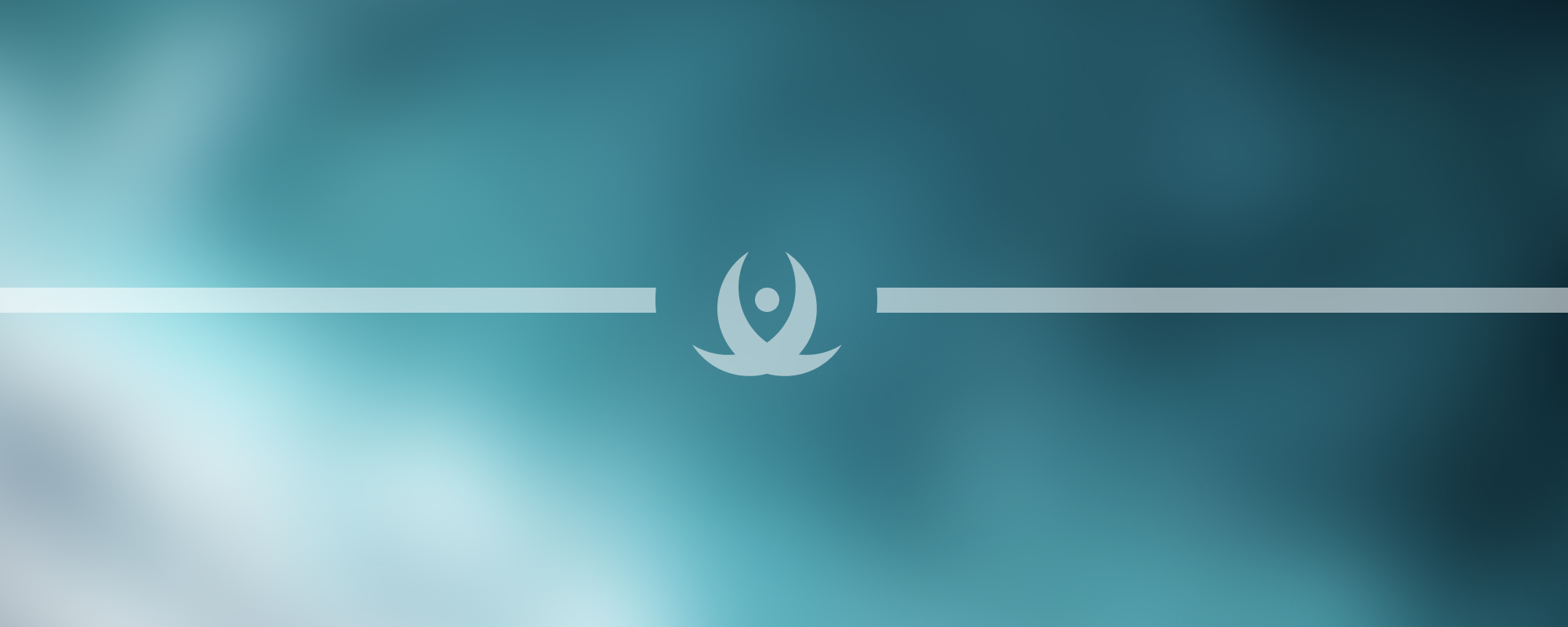
3. View Components (I)
View Components in C#
View components are similar to partial views in ASP.NET Core, but they're much more powerful. View components do not use model binding, and only depend on the data provided when calling into them.
Dependency Injection in View Components
Before discussing the details of View Components, let's understand what Dependency Injection is as it's a fundamental part of View Components.
Dependency Injection (DI) is a technique in which an object receives other objects that it depends on. These other objects are called dependencies. In the field of software engineering, DI is a design pattern that implements inversion of control for resolving dependencies.
In the context of View Components, DI comes into play in providing the services needed by the components.
For example:
public class ExampleViewComponent : ViewComponent
{
private readonly IProductRepository _productRepository;
public ExampleViewComponent(IProductRepository productRepository)
{
_productRepository = productRepository;
}
public IViewComponentResult Invoke()
{
return View(_productRepository.Products);
}
}
In the above example, IProductRepository
is a service that's injected into ExampleViewComponent
through its constructor.
AddSingleton
The AddSingleton
method is one of the methods provided by the .NET Core framework to add services to the DI container. When you register a service using AddSingleton
, the same instance of the service is used every time it's requested.
Here's an example of how AddSingleton
can be used:
services.AddSingleton<IProductRepository, EFProductRepository>();
In the above example, IProductRepository
is an interface and EFProductRepository
is a class that implements this interface. AddSingleton
creates a single instance of EFProductRepository
and uses that every time an IProductRepository
is needed.
AddScoped
The AddScoped
method creates a new instance of the service for each request. This is useful in scenarios where you want to ensure that the service does not maintain any state between requests.
Here's an example of how AddScoped
can be used:
services.AddScoped<IProductRepository, EFProductRepository>();
In the above example, a new EFProductRepository
is created for each request.
AddTransient
The AddTransient
method creates a new instance of the service every time it's requested. This is even more granular than AddScoped
and ensures that the service does not maintain any state even within a single request.
Here's an example of how AddTransient
can be used:
services.AddTransient<IProductRepository, EFProductRepository>();
In the above example, a new EFProductRepository
is created every time an IProductRepository
is requested, even if it's within the same request.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version