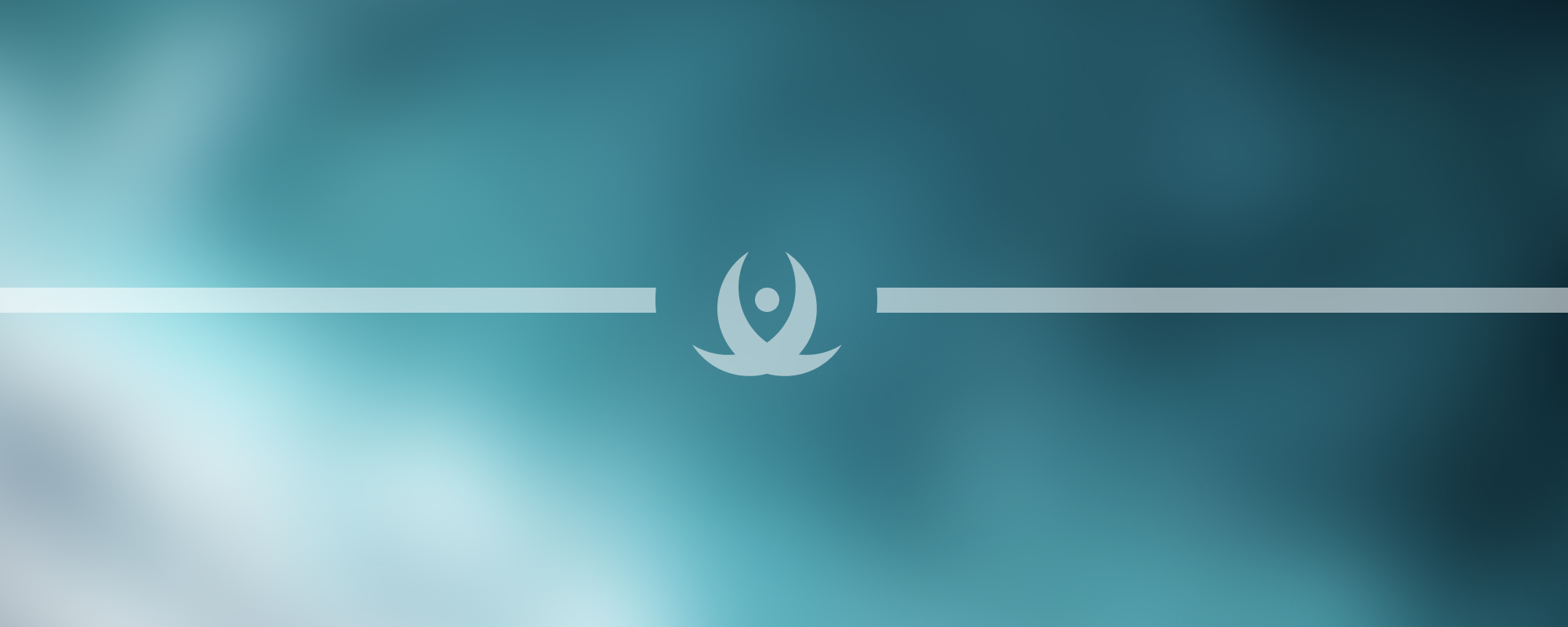
4. View Components (II)
Working with View Components in ASP.NET
Introduction
ASP.NET Core's View Components are an excellent tool for separating parts of your web application. They are dynamic, meaning they can change and adapt to different situations. This makes them more powerful than partial views, which are static.
Necessary Packages for CodeFirst and DatabaseFirst Models
When working with CodeFirst and DatabaseFirst models in ASP.NET, there are specific packages that you need to install.
For both models, you will need the following packages:
- Microsoft.EntityFrameworkCore
- Microsoft.EntityFrameworkCore.Design
- Microsoft.EntityFrameworkCore.SqlServer
- Microsoft.EntityFrameworkCore.Tools
Key Point:
- The versions of the packages must match the version of your project.
Understanding ASP.NET Core View Components
View Components in ASP.NET Core are similar to partial views but they are much more powerful. They are used to create reusable chunks of user interface (UI) that can contain both static and business logic. A View Component consists of two parts, the class (derived from ViewComponent) and the razor view.
Key Points:
- View Components are dynamic, meaning they can change based on the conditions of the application.
- They are not dependent on controllers and can be invoked from anywhere in the application.
Data Binding in View Components
ASP.NET Core provides multiple ways to pass data from controller to view and vice versa. These include ViewData
, ViewBag
, and TempData
.
[BindProperty]
is an attribute that can be applied to properties in a Razor Page model, and it enables model binding.
TempData
is a dictionary object that is used to store data temporarily between two consecutive requests.
ViewData
is a dictionary object that stays for the duration of the HTTP Request. It can be used to maintain data between controller and views.
ViewBag
is a dynamic object, which means it can dynamically add properties to the object.
Razor Pages
Razor Pages are a new aspect of ASP.NET Core MVC that allows for page-based programming model. A Razor Page is a combination of a Razor view with a specific page model class, where you can put your handler methods.
Code Examples
In the following example, a View Component is defined and invoked in a Razor page:
public class PriorityList: ViewComponent
{
private readonly ApplicationDbContext db;
public PriorityList(ApplicationDbContext context)
{
db = context;
}
public async Task<IViewComponentResult> InvokeAsync(
int maxPriority, bool isDone)
{
var items = await GetItemsAsync(maxPriority, isDone);
return View(items);
}
private Task<List<TodoItem>> GetItemsAsync(int maxPriority, bool isDone)
{
return db.ToDo.Where(x => x.IsDone == isDone &&
x.Priority <= maxPriority).ToListAsync();
}
}
To invoke the View Component, we use the Component.InvokeAsync
method:
@await Component.InvokeAsync("PriorityList", new { maxPriority = 2, isDone = false })
In this example, PriorityList
is the name of the View Component and maxPriority
and isDone
are parameters.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version