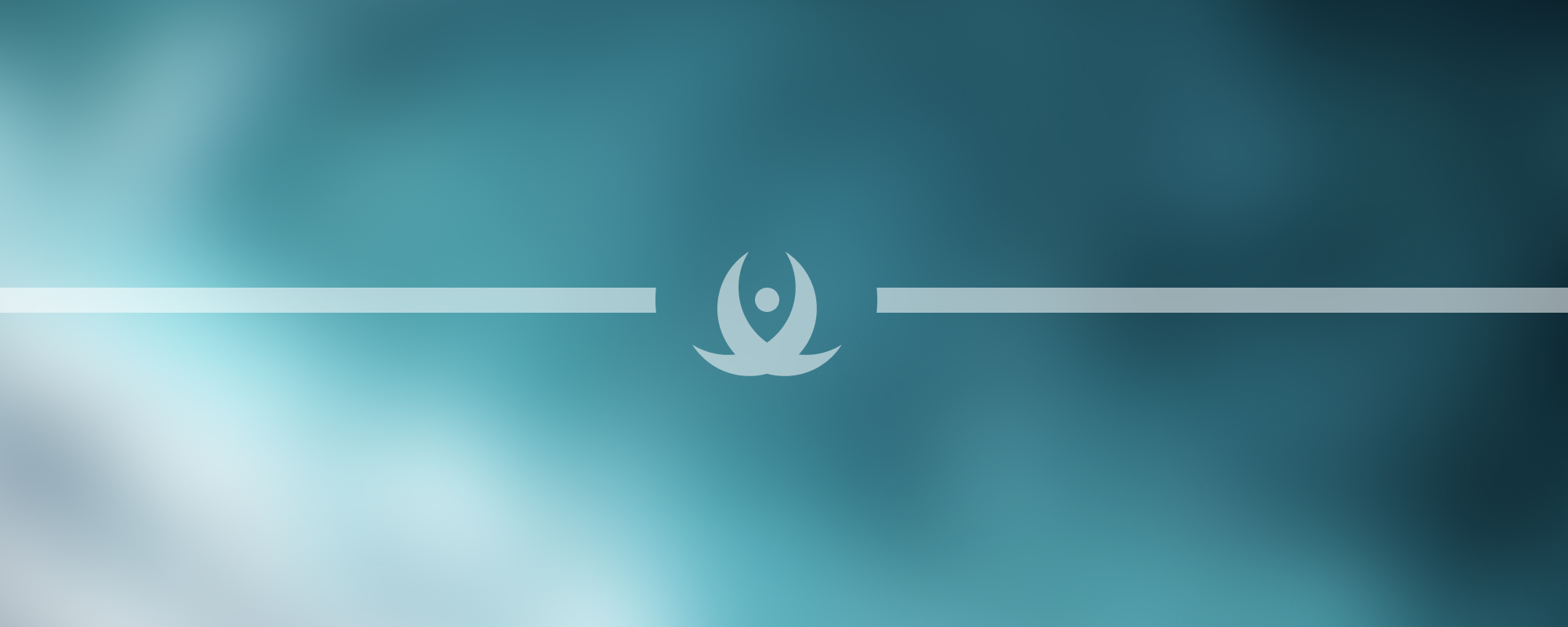
5. ECommerce Monolith Structure
ECommerce Monolith Structure in ASP.NET
Introduction to ASP.NET Session
The session in ASP.NET is a state management technique that is used to store and retrieve user data. It helps to identify requests from the same browser during a specific time period, referred to as a 'session'.
Key Features of ASP.NET Session
- Session State: Used to store and retrieve user data temporarily.
- Identifies User Requests: Helps in identifying requests from the same browser during a specific time period.
- Time-Bound: The data in a session is stored for a particular time period.
Example of using Session in ASP.NET:
Session["UserName"] = "JohnDoe"; // Store data in session
string userName = Session["UserName"].ToString(); // Retrieve data from session
Monolithic Architecture
A monolithic architecture is a software design pattern where the application is developed as one cohesive unit. The application's codebase is interconnected and interdependent, rather than segregated into microservices.
Pros and Cons of Monolithic Architecture
Pros
- Simplicity: Developing and deploying a monolithic application can be simpler since it involves managing a single codebase.
- Consistency: A monolithic architecture allows for shared-memory access and can be beneficial for tasks that require intense communication between components.
Cons
- Scalability: Monolithic applications can be challenging to scale when different modules have conflicting resource requirements.
- Reliability: If one module of a monolithic application fails, it can lead to the failure of the entire system.
Example of Monolithic Architecture:
In an eCommerce platform, components like User Interface, Product Catalog, Shopping Cart, etc., are interdependent and exist as a single unit.
public class ECommercePlatform
{
public UserInterface UI { get; set; }
public ProductCatalog Catalog { get; set; }
public ShoppingCart Cart { get; set; }
// ... Other components
}
When to Use Monolithic Architecture
Despite its drawbacks, a monolithic architecture is suitable in the following scenarios:
- Small Applications: For small-scale applications with limited modules, a monolithic architecture can be beneficial due to its simplicity.
- Well-Planned Architecture: If the application's growth and scale can be accurately predicted, a monolithic architecture can be suitable.
Conversely, for large-scale applications with unpredictable growth, a microservices architecture might be more suitable.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version