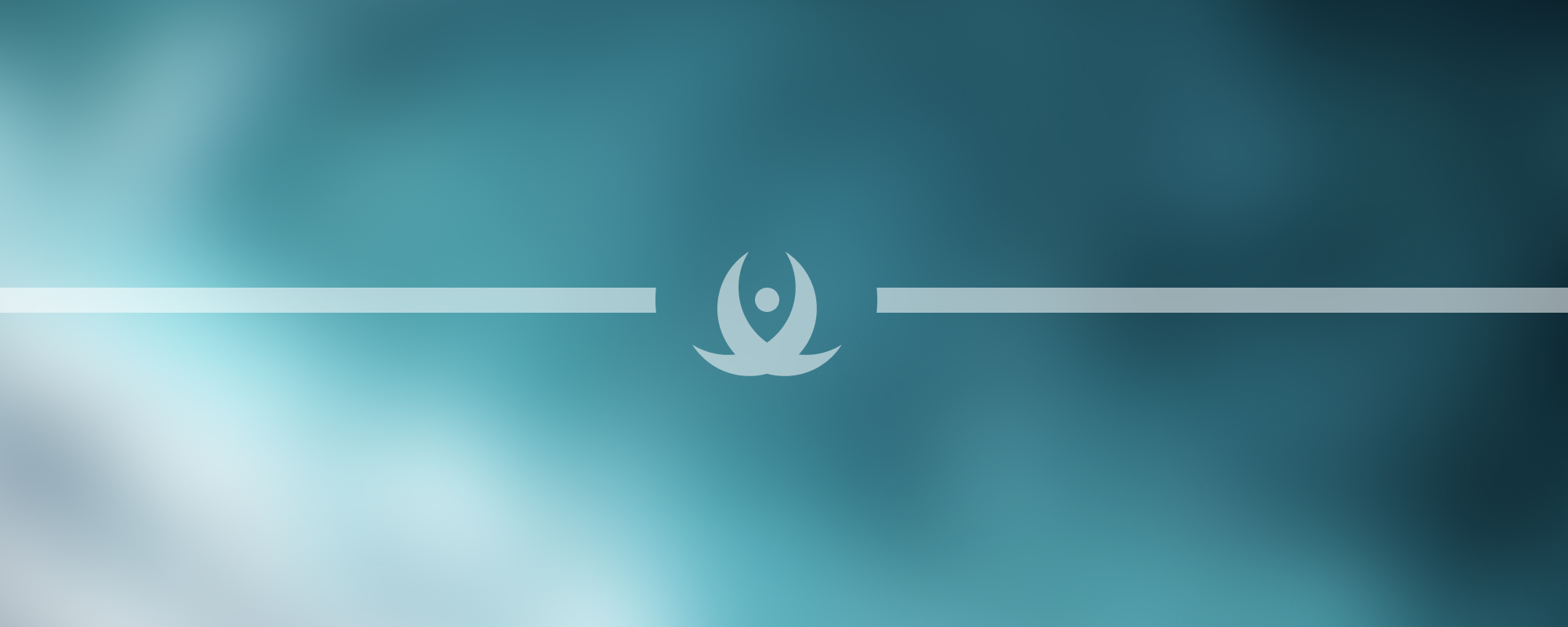
6. ECommerce MVC Project Development (I)
E-Commerce MVC Project Development
Overview
This guide focuses on the development of an E-Commerce project utilizing the Model-View-Controller (MVC) architectural pattern. The MVC pattern is a software design pattern that separates an application into three interconnected components: Model, View, and Controller. This separation provides a more organized and modular way to handle application development, making it a popular choice for web application development, including E-Commerce platforms.
MVC Architecture
Model
The Model represents the data of the application. It is responsible for retrieving, storing, and updating this data. In an E-Commerce platform, models might include User, Product, Order, and more.
Example:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
View
The View is what the user sees and interacts with. It represents the presentation of the data. For an E-Commerce site, views would include product listings, shopping cart, user profiles, and others.
Example:
<div>
<h2>@Model.Name</h2>
<p>Price: @Model.Price</p>
</div>
Controller
The Controller handles the user interaction, works with the model, and ultimately selects a view to render. In E-Commerce, controllers would handle tasks such as adding items to a shopping cart, user registration and login, processing orders, and more.
Example:
public class ShoppingCartController : Controller
{
public ActionResult AddToCart(int id)
{
// Implementation logic goes here
}
}
Application Flow
In an MVC E-Commerce application, the flow of control would typically be as follows:
- The user interacts with the view (e.g., clicks a "Buy Now" button).
- The controller handling the user input retrieves the necessary data from the model (e.g., product details).
- The controller performs the necessary operation (e.g., adds the product to the shopping cart).
- The controller selects an appropriate view (e.g., the shopping cart view) and passes the model data to it.
- The view is rendered to the user, displaying the updated model data (e.g., the updated shopping cart).
Building an E-Commerce Platform with MVC
When building an E-Commerce platform using MVC architecture, consider the following key points:
- User Authentication: Implement a robust user authentication and authorization system. This will help secure user data and allow for personalized experiences.
- Product Management: Create comprehensive product models and views for displaying product details, and controllers for managing product data.
- Shopping Cart: Implement a shopping cart feature that allows users to add products, view their cart, and modify its contents before checkout.
- Order Processing: Develop an efficient order processing system. This includes order models, views for order review and confirmation, and controllers that handle the order transactions.
- Payment Integration: Integrate with a secure payment gateway for handling payments. This involves coordinating with the payment gateway's API and handling responses to ensure orders are properly paid and recorded.
Remember, the key to a successful E-Commerce platform is a seamless user experience, which can be effectively achieved with the organized structure and separation of concerns provided by the MVC architecture.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version