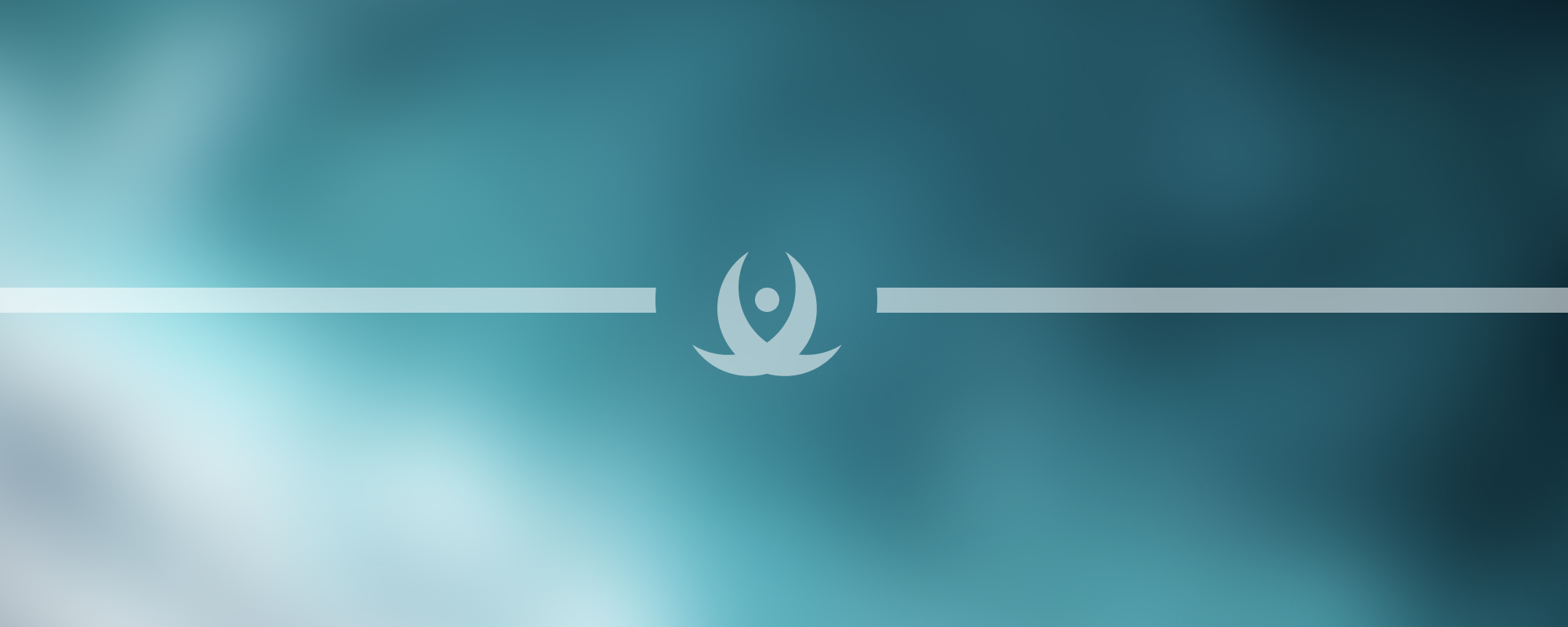
7. ECommerce MVC Project Development (II)
ECommerce MVC Project Development
In this lesson, we delve into the development of an eCommerce project using the MVC (Model-View-Controller) architectural pattern. The MVC pattern separates the application into three interconnected components enabling efficient project management, easier application scaling, and improved control over the application.
Introduction to MVC
The MVC architecture is a design pattern used in software engineering that separates an application into three interconnected parts: Model, View, and Controller.
- Model: This handles the data and business logic of the application. It is responsible for retrieving and storing data, as well as any transformations or operations that the data may require.
- View: This is the user interface — it represents the data from the Model in a suitable format for the user to interact with.
- Controller: This is the link between the Model and the View. It processes user input and interactions, and updates the Model and View accordingly.
Building an ECommerce Project with MVC
When building an eCommerce project using the MVC pattern, the application could be structured as follows:
Model
The Model could comprise various classes representing different entities in the eCommerce platform, such as Product
, User
, Order
, Cart
, and so on. Each class will have fields representing attributes of the entity and methods for data manipulation. For instance, the Product
class could have fields like productId
, productName
, productDescription
, productPrice
, and so on.
Example:
public class Product {
public int productId { get; set; }
public string productName { get; set; }
public string productDescription { get; set; }
public decimal productPrice { get; set; }
}
View
The View could be made up of various pages like ProductList
, ProductDetails
, Cart
, Checkout
, and so on. Each of these pages will display data from the Model to the users and collect user inputs.
Controller
The Controller will have methods to handle user requests, retrieve data from the Model, and update the View. For instance, the ProductController
class could have methods like GetProductList
, GetProductDetails
, AddToCart
, etc.
Example:
public class ProductController {
public List<Product> GetProductList() {
// Retrieving product list from the Model
}
public Product GetProductDetails(int id) {
// Retrieving product details from the Model
}
public void AddToCart(int id) {
// Adding product to cart
}
}
Conclusion
Building an eCommerce project using the MVC pattern has several benefits, including improved maintainability, scalability, and control over the application. However, careful planning is needed to ensure a clear separation of concerns between the Model, View, and Controller.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version