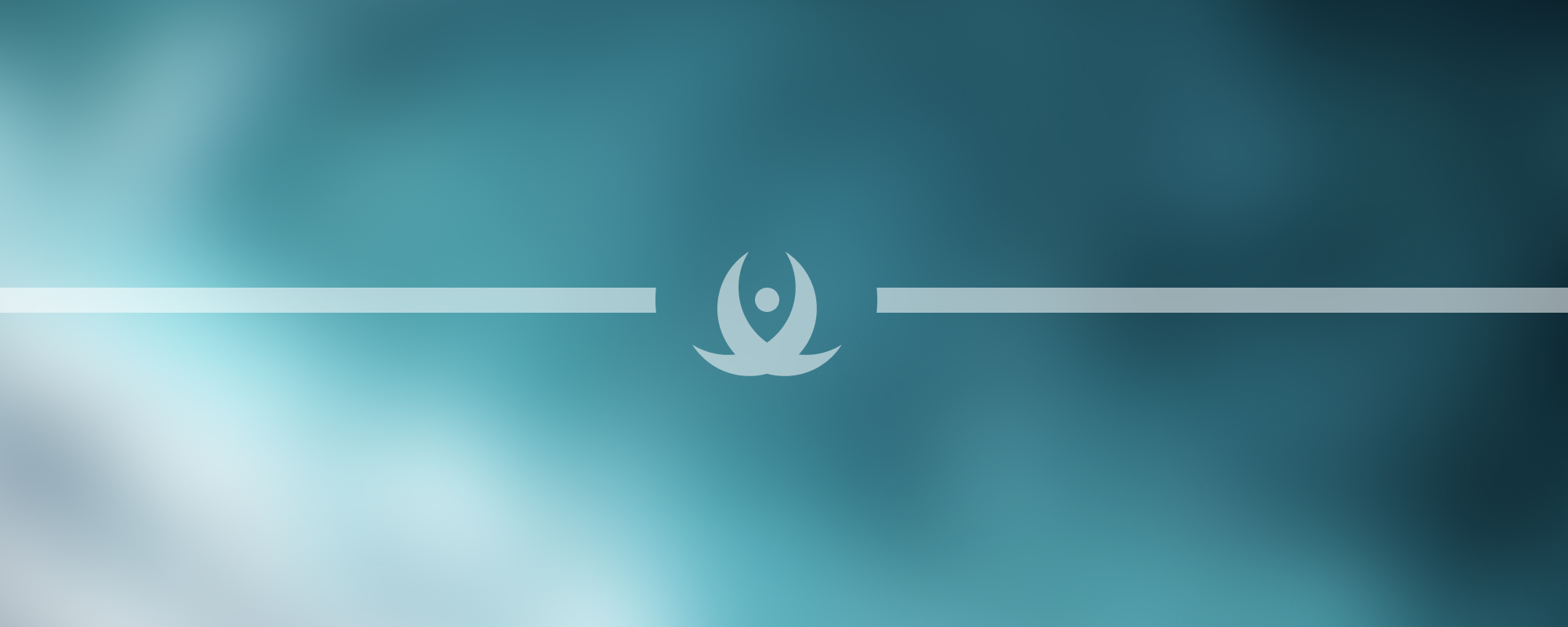
8. Account Module
Account Module in ASP.NET Core MVC
Introduction
The Account Module in ASP.NET Core MVC involves several key components such as IdentityUser
, IdentityRole
, IdentityDbContext
, UserManager
, RoleManager
, and SignInManager
. This note will delve into these components, their custom versions, and how they interact to create a robust account management system in ASP.NET Core MVC.
IdentityUser
Definition
In ASP.NET Core, IdentityUser
is a basic class that represents the user of the application. It includes properties such as UserName
, PasswordHash
, Email
, and PhoneNumber
.
Example
public class ApplicationUser : IdentityUser
{
}
IdentityRole
Definition
IdentityRole
represents the roles that users can have within the application. Each role is associated with a set of permissions.
Example
public class ApplicationRole : IdentityRole
{
}
IdentityDbContext
Definition
IdentityDbContext
is the Entity Framework database context used to manage and interact with the Identity-related tables in the database.
Example
public class ApplicationDbContext : IdentityDbContext<ApplicationUser>
{
public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options)
: base(options)
{
}
}
Custom Classes: CustomIdentityUser, CustomIdentityRole, CustomIdentityDbContext
You can extend the built-in classes (IdentityUser
, IdentityRole
, IdentityDbContext
) to create custom classes that fit your specific requirements.
Example
public class CustomIdentityUser : IdentityUser
{
public string CustomProperty { get; set; }
}
public class CustomIdentityRole : IdentityRole
{
public string CustomProperty { get; set; }
}
public class CustomIdentityDbContext : IdentityDbContext<CustomIdentityUser>
{
public CustomIdentityDbContext(DbContextOptions<CustomIdentityDbContext> options)
: base(options)
{
}
}
UserManager and RoleManager
UserManager
and RoleManager
are services provided by ASP.NET Core Identity to manage users and roles respectively. These services include methods to create, delete, update, and retrieve users and roles.
Example
public class AccountController : Controller
{
private readonly UserManager<CustomIdentityUser> _userManager;
private readonly RoleManager<CustomIdentityRole> _roleManager;
public AccountController(UserManager<CustomIdentityUser> userManager, RoleManager<CustomIdentityRole> roleManager)
{
_userManager = userManager;
_roleManager = roleManager;
}
}
SignInManager
SignInManager
is a service provided by ASP.NET Core Identity to manage user sign-ins.
Example
public class AccountController : Controller
{
private readonly SignInManager<CustomIdentityUser> _signInManager;
public AccountController(SignInManager<CustomIdentityUser> signInManager)
{
_signInManager = signInManager;
}
}
Conclusion
Understanding these components and their interactions is crucial to working effectively with the Account Module in ASP.NET Core MVC. For a more in-depth understanding, check out the sample code on GitHub and watch YouTube videos about ASP.NET Core MVC Role Management System.
References
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version