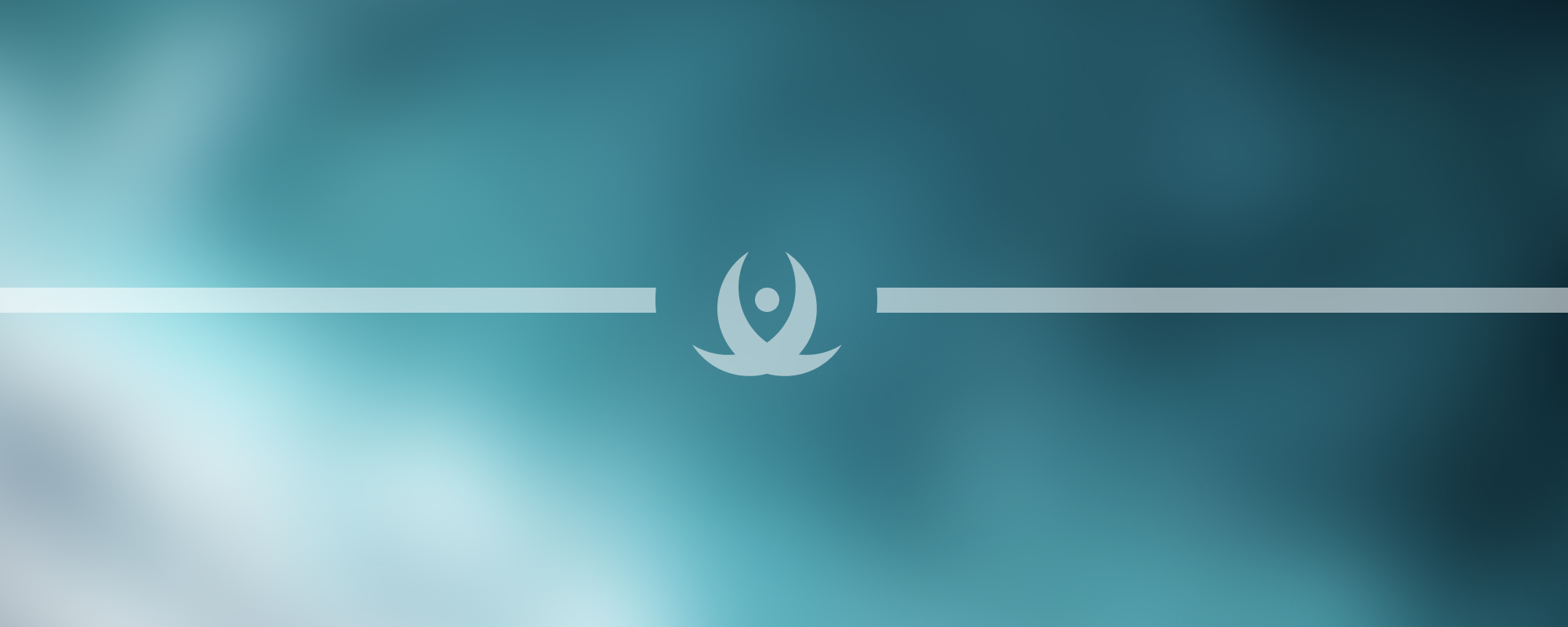
9. ASP ECommerce Project, Identity
ASP ECommerce Project: Identity
Introduction
ASP.NET Core Identity is a membership system that adds login functionality to ASP.NET Core apps. It includes features to manage user creation, email confirmation, password resets, and more. This system is crucial in ecommerce projects where user data and security is a primary concern.
Understanding ASP.NET Core Identity
ASP.NET Core Identity is an API that supports user interface (UI) login functionality. It allows users to create an account and login with their credentials, which is necessary for ecommerce websites to provide personalized experience and to manage transactions.
Key Points:
- It manages user creation, login, email confirmation, password reset, and more.
- ASP.NET Core Identity uses a default schema for the database that can be customized as required.
- It supports external logins where users can log in using their social media accounts such as Facebook, Google, etc.
Implementation of ASP.NET Core Identity in ECommerce Project
In an ecommerce project, ASP.NET Core Identity can be implemented to manage user identities. This section will provide a simple code example of how to incorporate ASP.NET Core Identity into an ecommerce project.
Setting up Identity
First, create a new ASP.NET Core MVC project. Then, install the necessary Identity package.
dotnet new mvc -n ECommerce
cd ECommerce
dotnet add package Microsoft.AspNetCore.Identity.EntityFrameworkCore
Creating User Class
Create a new class ApplicationUser
which will inherit from IdentityUser
.
public class ApplicationUser : IdentityUser
{
}
Configuring Identity in Startup.cs
In the Startup.cs
file, add the following code in the ConfigureServices
method to set up Identity.
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(
Configuration.GetConnectionString("DefaultConnection")));
services.AddDefaultIdentity<ApplicationUser>(options => options.SignIn.RequireConfirmedAccount = true)
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddControllersWithViews();
services.AddRazorPages();
}
Creating a Login View
Create a new Razor view for login under Views/Account
named Login.cshtml
.
@{
ViewData["Title"] = "Login";
}
<h2>@ViewData["Title"]</h2>
<div class="row">
<div class="col-md-8">
<section>
<form method="post" id="account">
<h4>Use a local account to log in.</h4>
<hr />
<div asp-validation-summary="All" class="text-danger"></div>
<div class="form-group">
<label asp-for="Input.Email"></label>
<input asp-for="Input.Email" class="form-control" />
<span asp-validation-for="Input.Email" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Input.Password"></label>
<input asp-for="Input.Password" class="form-control" />
<span asp-validation-for="Input.Password" class="text-danger"></span>
</div>
<button type="submit" class="btn btn-primary">Log in</button>
</form>
</section>
</div>
</div>
@section Scripts {
@await Html.PartialAsync("_ValidationScriptsPartial")
}
This is a basic implementation of ASP.NET Core Identity in an ecommerce project. To expand it further, we can add registration, password reset, email confirmation, and external login functionalities.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version