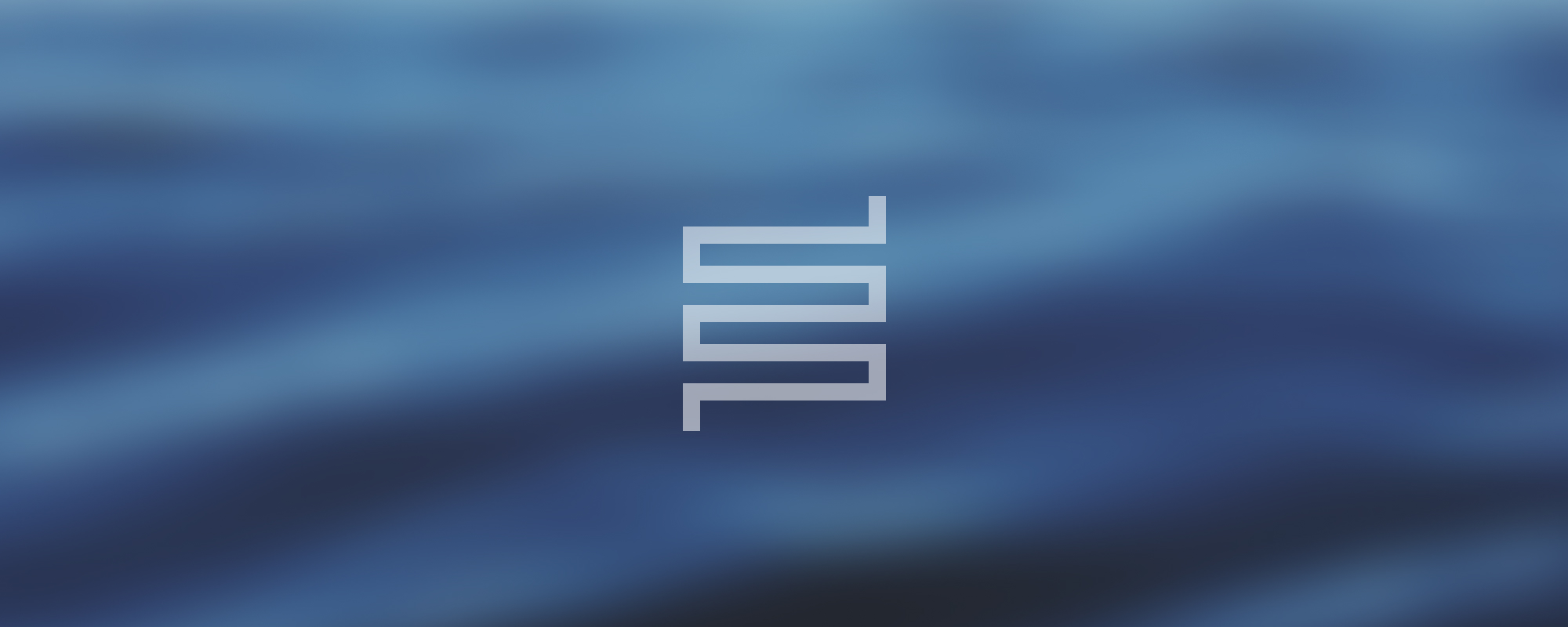
14. Object Relationships, Nested Classes, and Inheritance
Object Relationships in C++
Object-oriented programming (OOP) in C++ often involves relationships between objects. There are four primary types of object relationships:
- Composition
- Aggregation
- Association
- Dependency
Composition
Composition is a "has-a" relationship between classes. In a composition, an object is made up of other objects. If the composite object ceases to exist, so do its component objects. For example, a car is made up of several parts (engine, wheels, etc.). If the car is destroyed, its parts are also destroyed.
Aggregation
Aggregation is a less strict type of composition, where an object "has-a" relationship with other objects, but the component objects can exist independently. For example, a bank can have customers, but not all people (potential customers) have a bank account, and customers can exist independently of the bank.
Association
Association is a "uses-a" relationship between two classes. The objects are related, but they don't necessarily depend on each other to exist. For example, a doctor has a relationship with a patient, but both can exist independently of each other.
Dependency
Dependency is a "uses-a" relationship where one class depends on another if it uses it at some point in time. One class depends on another if it uses it, and changes to the class being used can affect the dependent class.
Nested (Embedded) Classes in C++
Nested classes, also known as inner classes, are a feature of C++ that allows you to define a class within another class. The nested class is only in scope within the outer class, aiding in encapsulation and improving readability.
Example of nested classes:
class List
{
public:
// ...
private:
class Node
{
public:
int data;
Node* next;
Node* prev;
};
private:
Node* head;
Node* tail;
};
void main()
{
List myList;
// ...
}
In this example, Node
is a nested class within List
, and it is only accessible within List
.
Inheritance in C++
Inheritance is a fundamental concept in OOP that allows one class to inherit properties and methods from another class. This leads to a hierarchical classification.
When a class is derived from another class, it inherits all the base class's public and protected members. This promotes code reusability and is a way to add additional features to an existing class without modifying it.
The class that is inherited from is known as the base class, superclass, or parent class, and the class that inherits is known as the derived class, subclass, or child class.
Inheritance in C++ can be implemented as follows:
class Base
{
// Base class code
};
class Derived : public Base
{
// Derived class code
};
In this example, Derived
is the subclass that inherits from the Base
superclass. Note that inheritance should only be used where there is a clear, logical "is-a" relationship between the classes. For instance, a Dog
class could logically inherit from an Animal
class, as a dog is an animal.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version