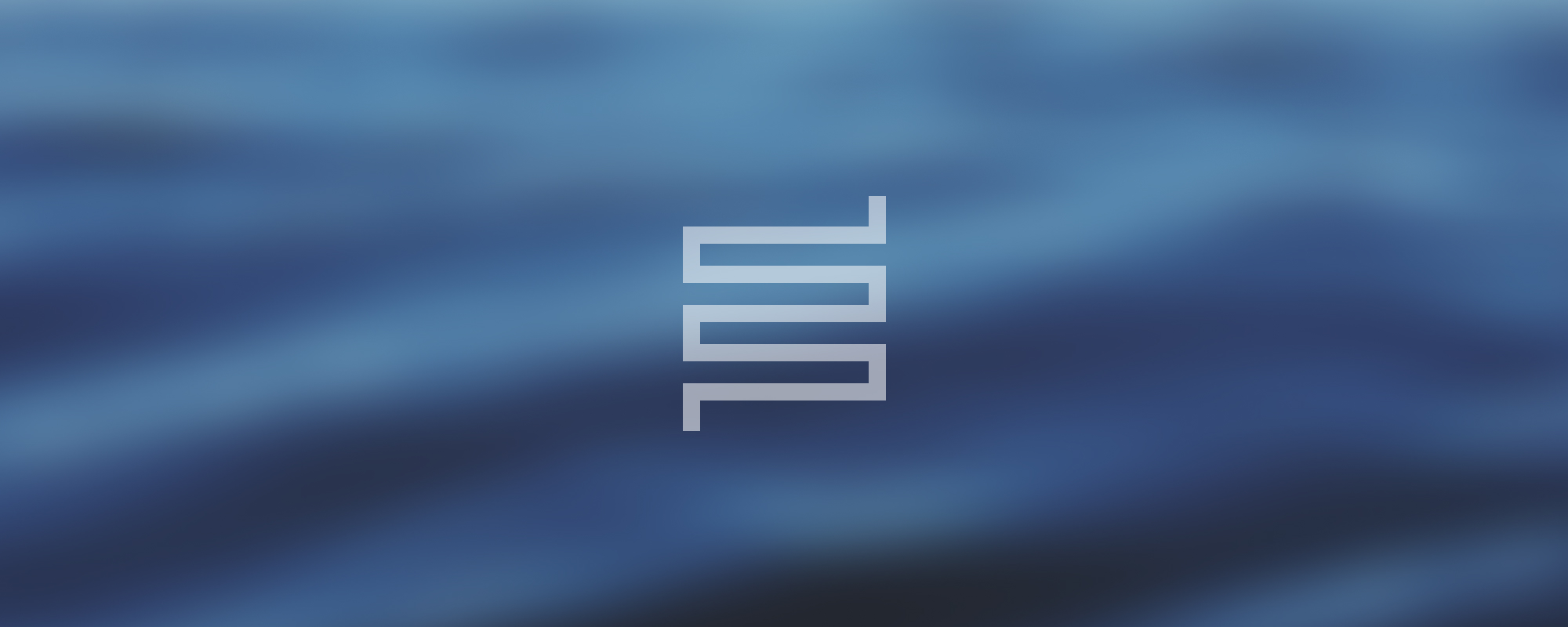
15. Inheritance in C++
What is Inheritance?
Inheritance is a fundamental concept in object-oriented programming (OOP). It allows for the creation of hierarchical relationships between classes, where one class can inherit properties and methods from another class. This is a powerful tool for reducing code redundancy and increasing the modularity of your code.
Types of Inheritance:
- Simple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Multiple Inheritance
1) Simple Inheritance
Simple Inheritance is when a class (derived/child class) inherits properties and methods from a single class (base/parent class).
Example:
class Animal {
public:
void eat() {
cout << "I can eat!" << endl;
}
};
class Dog : public Animal {
};
int main() {
Dog dog;
dog.eat(); // Output: "I can eat!"
return 0;
}
2) Multilevel Inheritance
Multilevel Inheritance is when a derived class is based on another derived class.
Example:
class Animal {
public:
void eat() {
cout << "I can eat!" << endl;
}
};
class Dog : public Animal {
public:
void bark() {
cout << "I can bark!" << endl;
}
};
class BabyDog : public Dog {
public:
void weep() {
cout << "I can weep!" << endl;
}
};
int main() {
BabyDog d;
d.weep();
d.bark();
d.eat();
return 0;
}
3) Hierarchical Inheritance
Hierarchical Inheritance is when multiple classes inherit from a single base class, creating a tree-like structure.
Example:
class Animal {
public:
void eat() {
cout << "I can eat!" << endl;
}
};
class Cat : public Animal {
public:
void meow() {
cout << "I can meow!" << endl;
}
};
class Dog : public Animal {
public:
void bark() {
cout << "I can bark!" << endl;
}
};
int main() {
Cat cat;
cat.meow();
cat.eat();
Dog dog;
dog.bark();
dog.eat();
return 0;
}
4) Multiple Inheritance
Multiple Inheritance is when a class inherits from more than one base class.
Example:
class Teacher {
public:
void teach() {
cout << "I can teach!" << endl;
}
};
class Student {
public:
void study() {
cout << "I can study!" << endl;
}
};
// SuperStudent inherits from both Teacher and Student
class SuperStudent : public Teacher, public Student {
};
int main() {
SuperStudent s;
s.teach();
s.study();
return 0;
}
Dealing with multiple inheritance conflicts
When using multiple inheritance, there can be conflicts such as the Diamond Problem, where a class inherits from two classes that have a common base class. This can lead to ambiguities and can be resolved with the use of the "virtual" keyword.
Example:
class A {
public:
A() {
cout << "Constructor of A" << endl;
}
};
// B and C classes inherit from A
class B : virtual public A {
};
class C : virtual public A {
};
// D inherits from both B and C
class D : public B, public C {
};
int main() {
D d; // Output: "Constructor of A"
return 0;
}
Additional Notes:
- To create an object of a derived class, an object of the base class must also be created.
- Private fields of the base class are always inaccessible (private) in the derived class.
- When a method is overridden in the derived class (a method in the base class is written with the same name in the derived class), the overridden method in the derived class is called instead of the base class method. For overriding, the "virtual" keyword must be used and there should be a pointer to the base class.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version