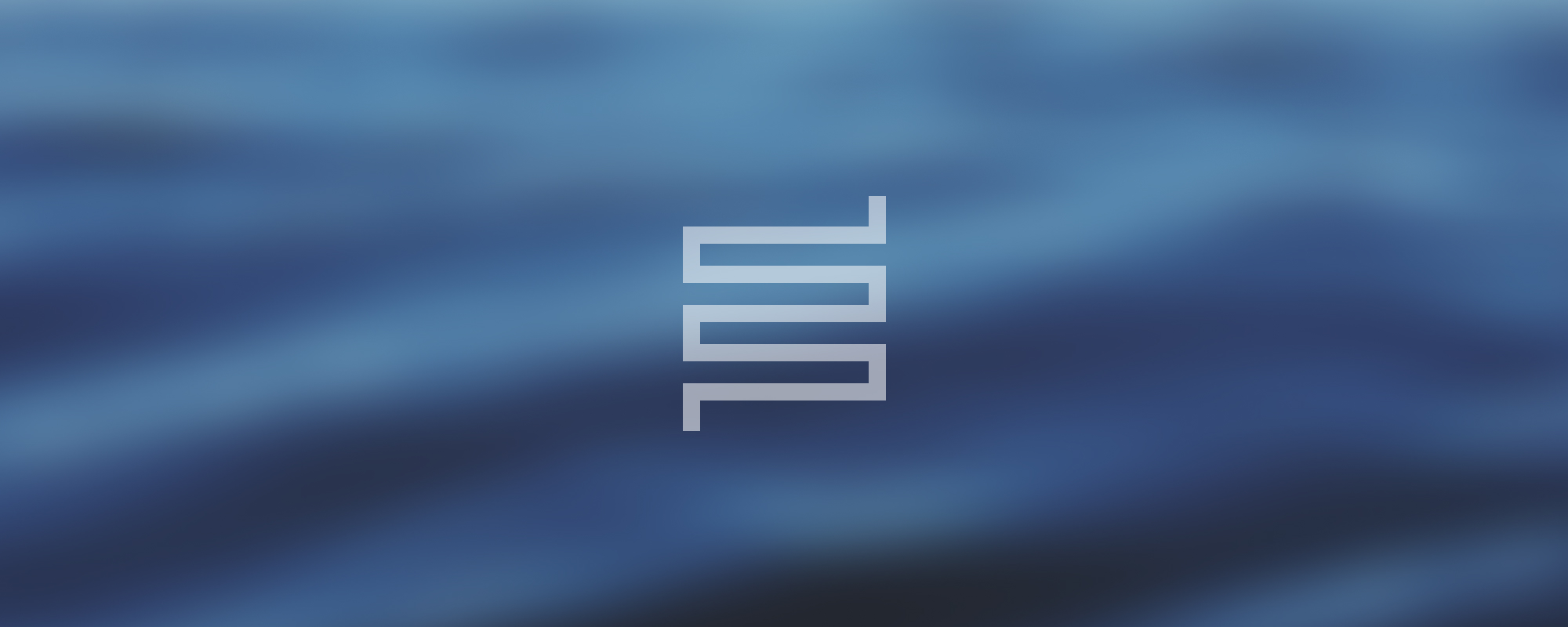
16. Polymorphism in C++
What is Polymorphism?
In object-oriented programming, polymorphism refers to the ability of an object to take on many forms. It's a principle that allows objects to behave differently in different contexts. In C++, polymorphism is primarily achieved through inheritance and virtual functions.
Key Points:
- Polymorphism occurs at runtime.
- It allows using a base class pointer to hold derived class objects.
- Polymorphism promotes code reusability and simplicity.
What is Inheritance?
Inheritance is a fundamental concept in C++ that allows creating a new class using properties and methods of an existing class. The new class is known as the derived class, and the existing class is referred to as the base class.
Example:
class Animal {
// Animal class code...
};
class Cat : public Animal {
// Cat class code...
};
Using Virtual Functions for Polymorphism
A virtual function is a function in a base class that can be overridden in a derived class. It is used to achieve runtime polymorphism. The virtual
keyword is used to create virtual functions.
Example:
class Base {
public:
virtual string GetName()
{
return "I am base";
}
};
class Derived : public Base {
public:
string GetName() override
{
return "I am derived";
}
};
int main() {
Base* base = new Derived();
cout << base->GetName() << endl; // OUTPUT: I am derived
}
Static and Dynamic Polymorphism
- Static Polymorphism (Early Binding): Here, the function call is resolved at compile time. C++ offers function overloading and templates for static polymorphism.
Example:
void Show() {
cout << 10 << endl;
}
int main() {
Show();
}
- Dynamic Polymorphism (Late Binding): In this, the function call is resolved at runtime. C++ uses virtual functions for dynamic polymorphism.
Example:
void add(int a, int b) {
cout << a + b << endl;
}
int main() {
void(*ptr)(int, int) = add;
ptr(10,20);
}
Do we always need Inheritance for Polymorphism?
No, we can achieve late binding or dynamic polymorphism using function pointers, without the need for inheritance.
Overriding in Derived Classes
When overriding a method in a derived class, we can use the override
keyword to ensure that the method is correctly overridden. This keyword also improves readability.
Inheritance and Final Keyword
The final
keyword can be used to prevent further overriding of a virtual function. Once a virtual function is declared as final
, it cannot be overridden in any further derived classes.
Example:
class A {
public:
virtual void Speak() {}
};
class B : public A {
public:
void Speak() override final {}
};
class C : public B {
// Cannot override Speak() here because it's declared as final in B
};
In the above example, the Speak()
function cannot be overridden in class C
because it was declared as final
in class B
.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version