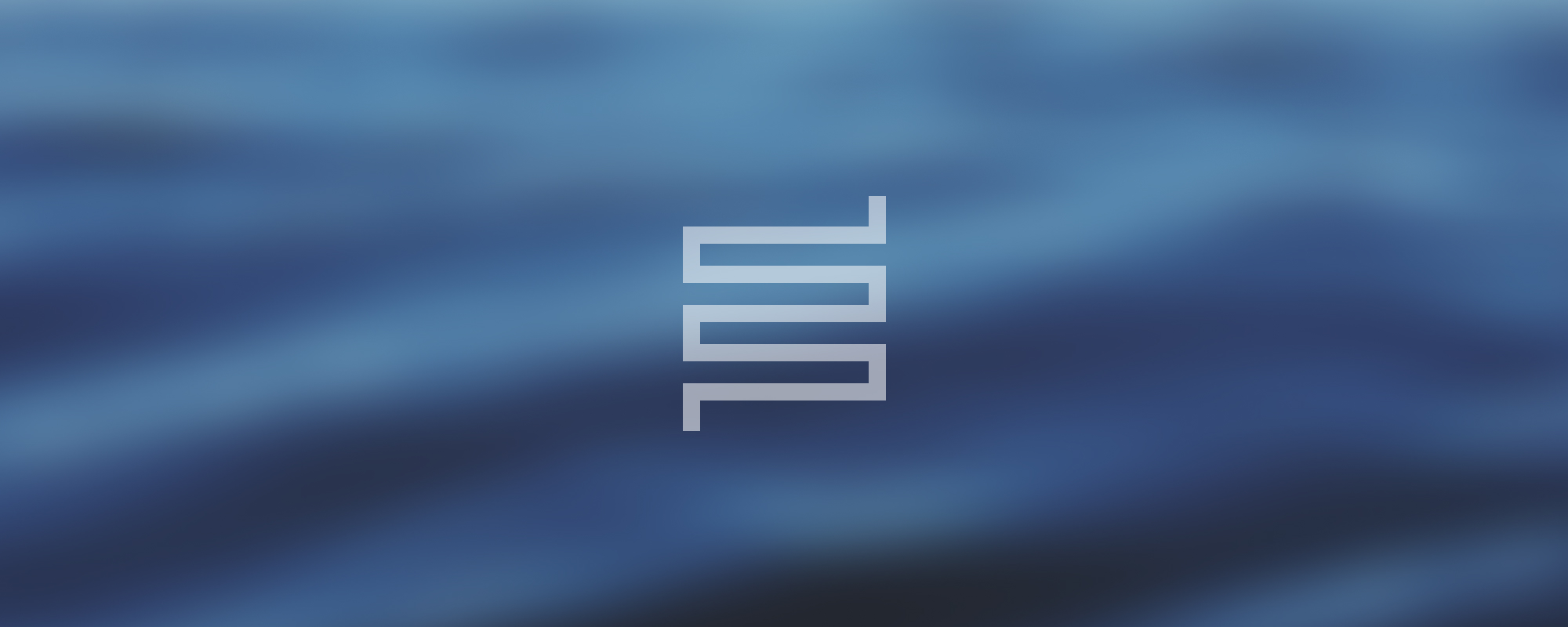
17. Abstraction in C++
Understanding Abstraction
Abstraction is one of the fundamental principles of Object-Oriented Programming. It refers to the process of hiding the complex details of an object and only exposing the relevant or essential features to the outside world. The main goal of abstraction is to handle complexity by hiding unnecessary details from the user.
Abstract Classes in C++
An abstract class in C++ is a class that cannot be instantiated, meaning we cannot create an object of an abstract class. Typically, abstract classes are used as base classes in inheritance hierarchies and they contain at least one pure virtual function.
Key Points:
- An abstract class cannot be instantiated.
- An abstract class can contain data members, methods (including constructors and destructors), and also pure virtual functions.
- A class containing at least one pure virtual function becomes an abstract class.
Example:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0;
};
Pure Virtual Functions
A pure virtual function is a function declared in a base class that has no definition relative to the base. A pure virtual function makes a class abstract and it must be implemented by any non-abstract class that directly or indirectly inherits from the class that the pure virtual function is defined.
Example:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0;
};
class ConcreteClass : public AbstractClass {
public:
void pureVirtualFunction() override {
// Implementation of pure virtual function
}
};
Interfaces in C++
In C++, an Interface is an abstract class with all its methods as pure virtual. It provides a way to ensure that a class adheres to a certain contract or interface.
Example:
class Interface {
public:
virtual void method1() = 0;
virtual void method2() = 0;
};
Pure Virtual Destructors
In C++, a pure virtual destructor is allowed. However, even if destructors are declared as pure virtual, they must provide a definition, because when an object of a derived class is destroyed, it first calls the destructor of the derived class, then it calls the destructor of the base class.
Example:
class AbstractClass {
public:
virtual ~AbstractClass() = 0; // Pure virtual destructor
};
AbstractClass::~AbstractClass() {
// Implementation of pure virtual destructor
}
Derived class destructor overrides the base class destructor:
class ConcreteClass : public AbstractClass {
public:
~ConcreteClass() {
// Implementation of destructor
}
};
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version