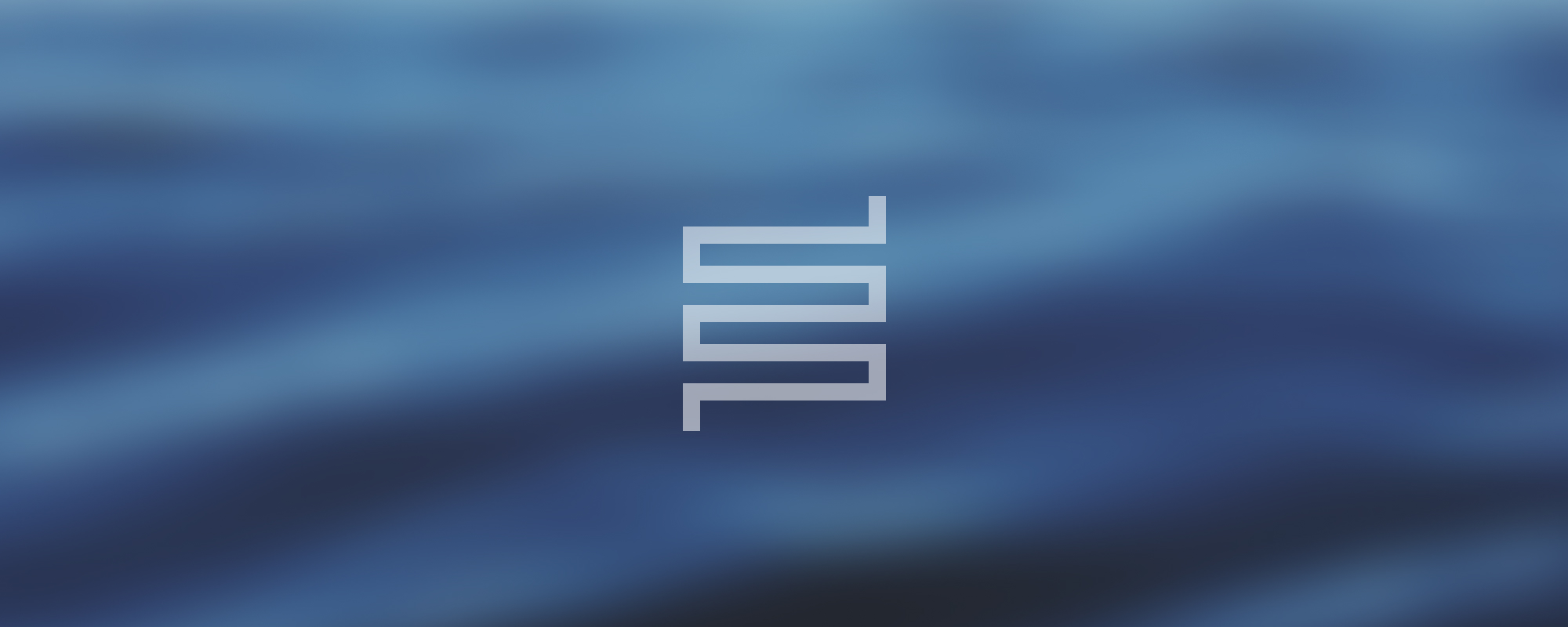
18. Introduction to Exception Handling
Introduction to Exception Handling in C++
Exception handling in C++ is a process to handle errors and it prevents the normal flow of the program from being disrupted. It is a powerful mechanism to handle runtime errors. This can aid in preventing the program from crashing.
Purpose of Exception Handling
When we have a function that cannot find what it is looking for, it typically returns -1. However, this isn't very detailed. Throwing an exception allows us to return more detailed information, including the time of the exception, the line of code where it occurred, and the file in which it is located.
You can throw exceptions of any data type: class, string, int, enum etc.
Throwing and Catching Exceptions
Exceptions can be thrown and handled within a function using the try
and catch
blocks.
The try
block surrounds the set of instructions that might throw an exception while the catch
block is used to handle the exception that has been thrown by the try
block.
Here is a simple example:
try
{
doSomething(-10); // Function that might throw an exception
}
catch (const char* ex) // Catches exceptions of type const char*
{
cout << "Error : " << ex << endl;
}
catch (int ex) // Catches exceptions of type int
{
cout << "Error : " << ex << endl;
}
The code after an exception is thrown does not get executed. Control is directly transferred to the catch
block.
If it cannot find a catch
block to handle the exception, the program will terminate with an error.
Catching All Exceptions
If we want to catch all type of exceptions regardless of their type, we can use an ellipsis ...
as the parameter for the catch
block:
void main()
{
try
{
doSomething(2); // Function that might throw an exception
}
catch (int)
{
cout << "Int Error" << endl;
}
catch (const char*)
{
cout << "Const char error" << endl;
}
catch (...)
{
cout << "General Error" << endl;
}
}
The downside of this approach is that we will not be able to identify the type of the exception, thus limiting our ability to respond to the error appropriately.
Conclusion
Exception handling is a crucial aspect of coding in C++. Proper use of try
and catch
blocks can greatly improve the robustness of your code and enhance the user experience by providing more informative error messages.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version