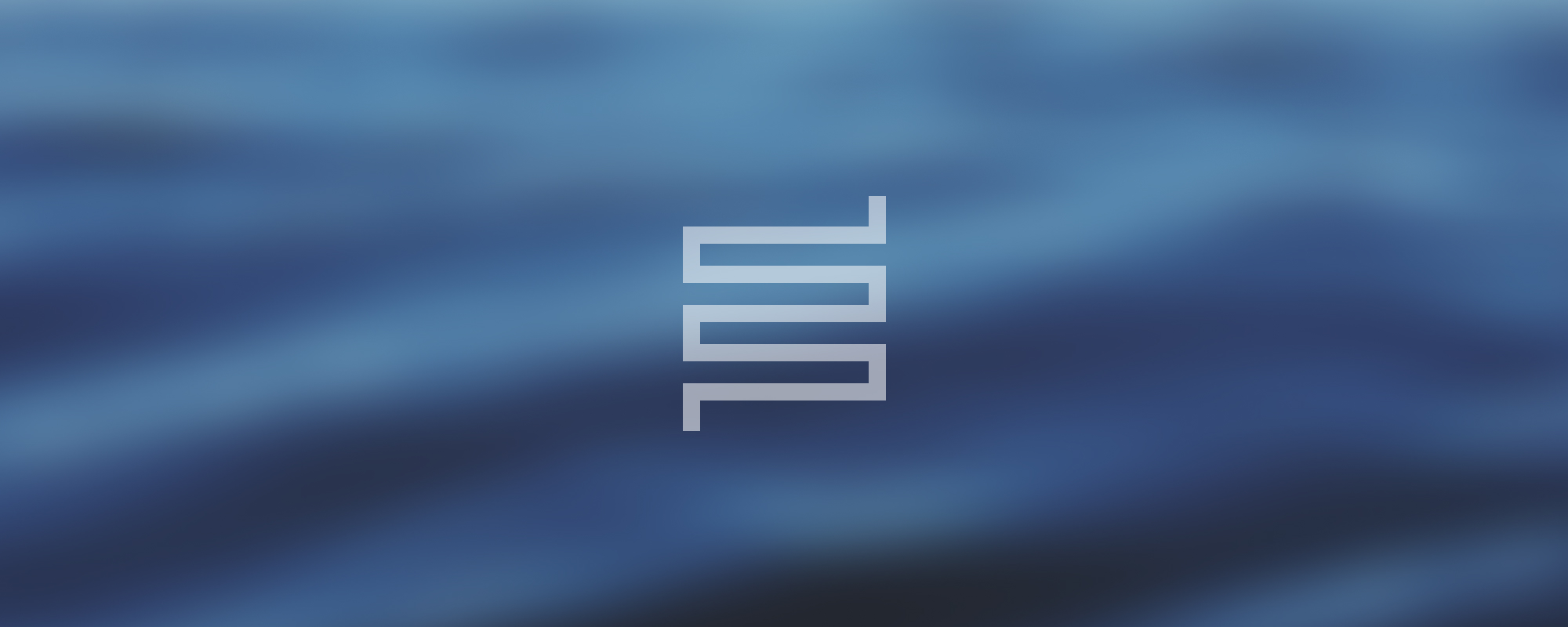
20. Type Identification, Polymorphism, Mutable, and Casting
Introduction
In this lesson, we explore key features of the C++ language, specifically focusing on type identification, polymorphism, the mutable
keyword, and the four types of casting supported by the language.
Understanding Type Identification
C++ provides a typeid
keyword, used to identify the type of an expression. This is particularly useful when dealing with polymorphic classes.
#include <typeinfo>
int i = 5;
float j = 1.5f;
char c = 'a';
const type_info& t1 = typeid(i * j);
const type_info& t2 = typeid(i * c);
const type_info& t3 = typeid(c);
cout << "t1 is of type " << t1.name() << endl;
cout << "t2 is of type " << t2.name() << endl;
cout << "t3 is of type " << t3.name() << endl;
Polymorphism in C++
For a class to be polymorphic in C++, it must contain at least one virtual function. The keyword virtual
is used to allow a function to be overridden in a derived class.
The Mutable Keyword
The mutable
keyword allows a member of an object to be modified even if the object is declared as const
.
class Student
{
int roll;
mutable int roll2;
public:
Student(int r) : roll(r)
{
}
void Fun() const
{
this->roll2 = 200; // okay because roll2 is mutable
}
};
C++ Casting Types
C++ supports four types of casting: static_cast
, dynamic_cast
, const_cast
, and reinterpret_cast
.
Static Cast
static_cast
is used for conversion of types, where the compiler checks the validity of the conversion during compilation. It throws a compile error if the conversion is not possible.
int x = 10;
double y = static_cast<double>(x);
Dynamic Cast
dynamic_cast
is used for converting base-class pointers into derived-class pointers. It returns nullptr
if the casting fails.
class Base { virtual void fun() {} };
class Derived: public Base { int a; };
Base * base = new Derived;
Derived * derived = dynamic_cast<Derived*>(base);
Const Cast
const_cast
is used to remove the const
qualifier from a variable.
const int x = 10;
int* y = const_cast<int*>(&x);
Reinterpret Cast
reinterpret_cast
is used for any type of conversion, preserving the bit pattern in memory. It's typically used for low-level programming tasks.
int* p = new int(65);
char* ch = reinterpret_cast<char*>(p);
Conclusion
In C++, understanding how to work with type identification, polymorphism, the mutable
keyword, and the different casting types is crucial. These features provide the flexibility and control needed to write robust and efficient code.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version