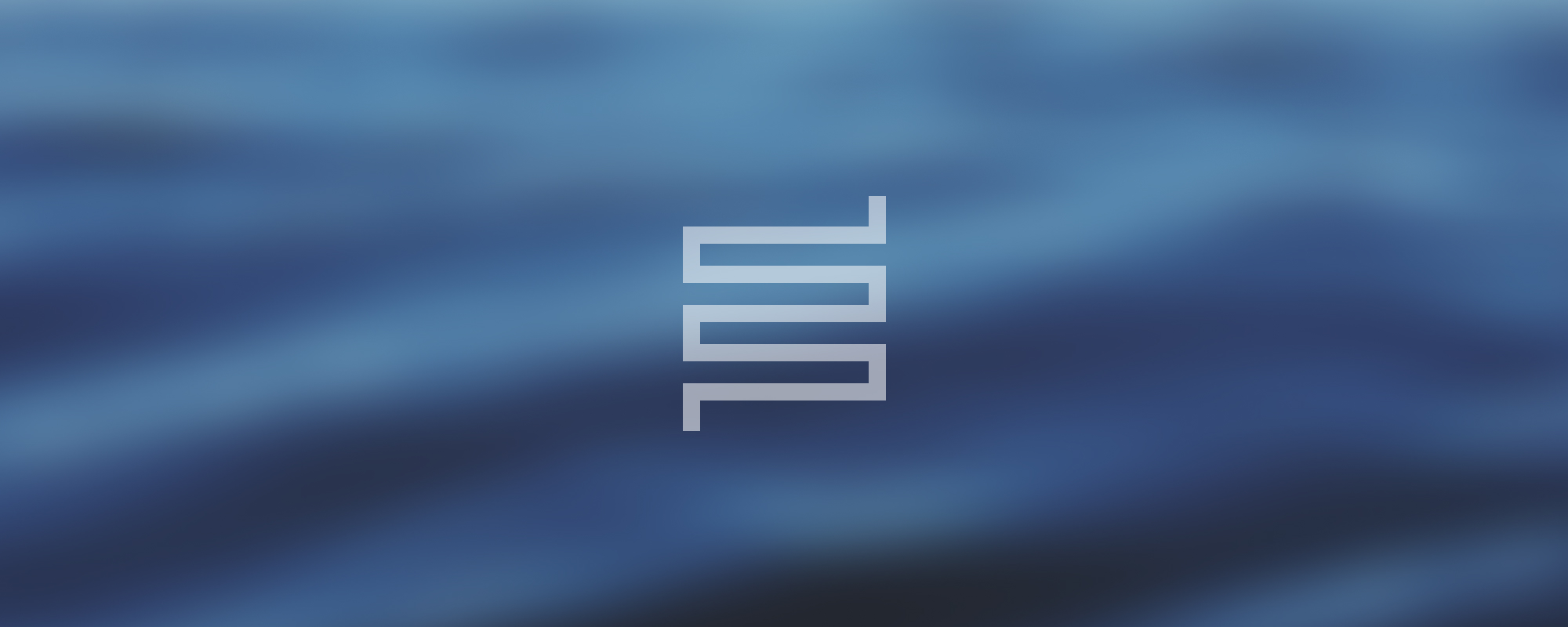
21. Streams and String Streams
Overview
In C++, streams and string streams are important concepts, especially in handling inputs and outputs. This lesson will cover these topics in detail, providing formal definitions, examples, and clarifying common misunderstandings.
Streams in C++
A stream in C++ is an abstraction that represents a device on which input and output operations are performed. A stream can basically be represented as a source or destination of characters of indefinite length. In other words, from a stream, you can either derive data/input (get) or provide data/output (put).
All streams are derived from the ios_base
class.
Example:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
Console Stream Types
cout
(console out) : Used for output, to display data on the console.
cin
(console in) : Used for input, to take data from the user.
Example:
cout << "Hello, World!" << endl; // Output "Hello, World!" to the console
int number;
cin >> number; // Take an integer input from the user
Formatted Output
The showpos
manipulator can be used to indicate the sign of a number, and boolalpha
to print boolean values as "true" or "false".
Example:
int num = 10;
cout << showpos << num << endl; // OUTPUT: +10
bool result = true;
cout << boolalpha << result << endl; // OUTPUT: true
Alignment
The setw()
function sets the field width, while left
and right
manipulators set the alignment.
Example:
cout << "|" << setw(5) << left << 1 << "|" << endl; // OUTPUT: |1 |
cout << "|" << setw(5) << right << 1 << "|" << endl; // OUTPUT: | 1|
String Streams
A string stream is a stream that can be used to operate on strings. It is used to convert strings to numerical values and vice versa. The sstream
library provides us with the string stream classes.
Unlike other streams, data cannot be stored in string streams. Once you've read from a string stream, you won't be able to read it again. This makes string streams more memory efficient.
Example:
#include <iostream>
#include <sstream>
using namespace std;
int main() {
stringstream os;
os << "Hello World and Programmers";
string data = "";
os >> data;
cout << data << " "; // OUTPUT: Hello
os >> data;
cout << data << " "; // OUTPUT: World
os >> data;
cout << data << " "; // OUTPUT: and
os >> data;
cout << data << endl; // OUTPUT: Programmers
return 0;
}
Reading from a String Stream
getline()
can be used to read a line from a string stream.
Example:
#include <iostream>
#include <sstream>
using namespace std;
int main() {
stringstream os;
os << "Hello World and Programmers \\n Today is an unhappy day" << endl;
string data;
getline(os, data);
cout << data << endl; // OUTPUT: Hello World and Programmers
getline(os, data);
cout << data << endl; // OUTPUT: Today is an unhappy day
return 0;
}
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version