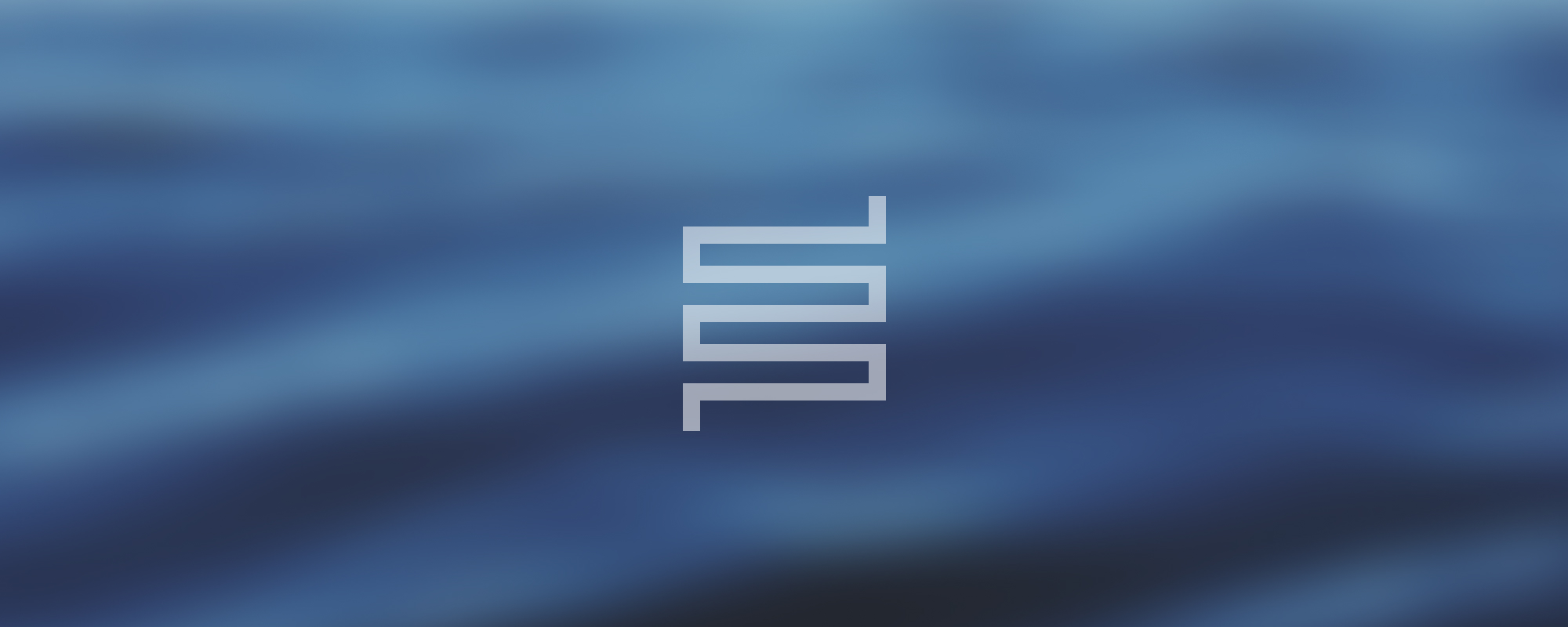
22. Working with Files in C++
Working with Files in C++
In C++, we often need to read data from or write data to external files. The C++ standard library provides us with the tools to perform these tasks.
To work with files in C++, we must include the <fstream>
library, which provides us with filestream classes.
#include <fstream>
File Operations in C++
The primary operations we can perform on files are:
- Writing to a file (output operation)
- Reading from a file (input operation)
- Appending data to a file (appending operation)
Text File Modes
1. Writing to Text Files
To write to a file, we use the ofstream
(output file stream) class. We use the ios::out
mode to open the file for writing.
std::ofstream outFile("filename", std::ios::out);
2. Reading from Text Files
To read from a file, we use the ifstream
(input file stream) class. We use the ios::in
mode to open the file for reading.
std::ifstream inFile("filename", std::ios::in);
3. Appending to Text Files
To append data to a file, we again use the ofstream
class. We use the ios::app
mode to open the file for appending.
std::ofstream appendFile("filename", std::ios::app);
Binary File Modes
Binary files are files that contain data in a binary format which is only readable by computers. Unlike text files, these files are not human-readable.
1. Writing to Binary Files
To write to a binary file, we use the ofstream
class in combination with the ios_base::binary
and ios_base::out
modes.
std::ofstream binaryOutFile("filename", std::ios_base::binary | std::ios_base::out);
2. Reading from Binary Files
To read from a binary file, we use the ifstream
class with the ios_base::binary
mode.
std::ifstream binaryInFile("filename", std::ios_base::binary);
3. Appending to Binary Files
To append data to a binary file, we use the ofstream
class with the ios_base::binary
and ios_base::app
modes.
std::ofstream binaryAppendFile("filename", std::ios_base::binary | std::ios_base::app);
In conclusion, C++ provides a powerful and flexible set of tools for working with both text and binary files. Using the fstream
library, we can easily read from, write to, and append to files in a variety of modes.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version