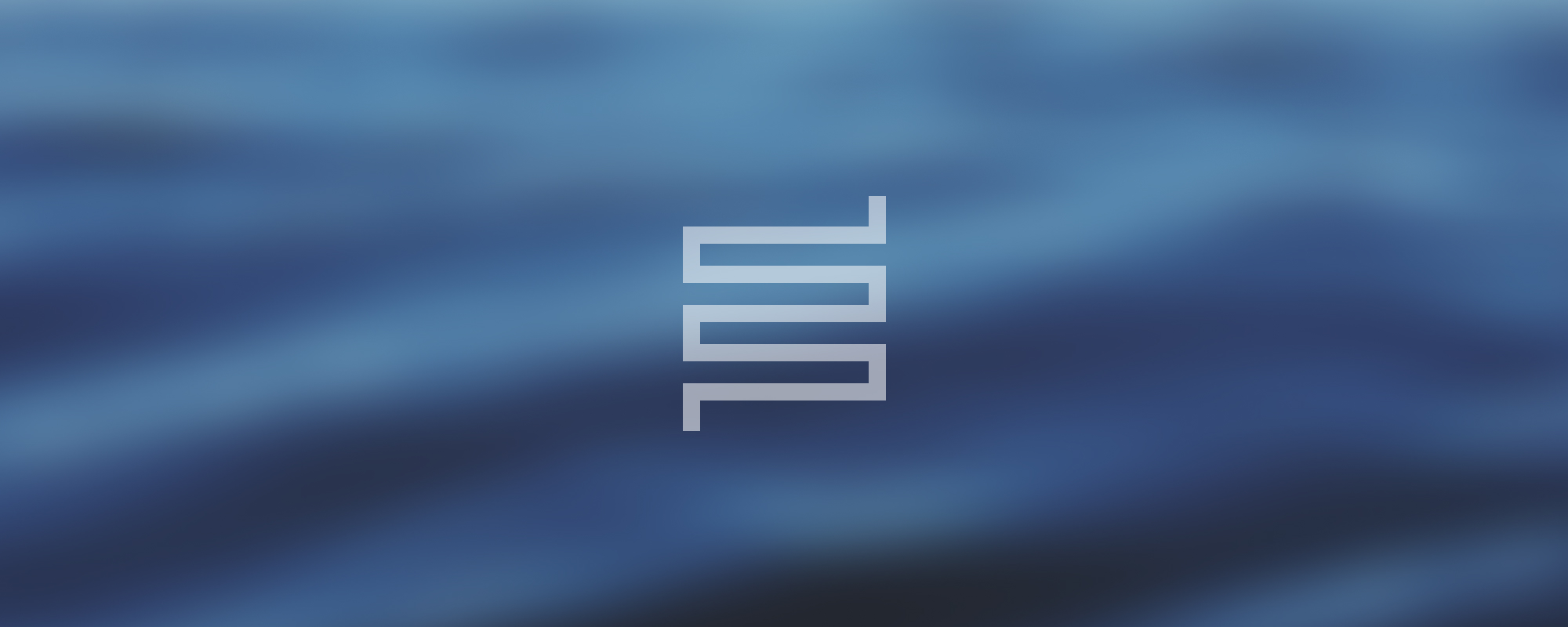
23. Smart Pointers
Introduction to Smart Pointers
In C++, a smart pointer is a template class that uses operator overloading to provide the functionality of a pointer while also providing automatic garbage collection. It automatically deallocates or frees the memory without the programmer needing to write code to free memory.
There are three types of smart pointers in C++:
- Shared Pointers
- Unique Pointers
- Weak Pointers
Unique Pointers
A unique pointer is a smart pointer that owns and manages another object through a pointer and disposes of that object when the unique_ptr goes out of scope. The main characteristic of a unique pointer is that it can only point to each memory address once, preventing multiple ownership.
This is achieved by blocking copy assignment and copy constructors.
Example:
// Creating a unique pointer
std::unique_ptr<int> p1 (new int(100));
// Transferring ownership
std::unique_ptr<int> p2 = move(p1);
Shared Pointers
A shared pointer is a reference-counting smart pointer in C++. It retains shared ownership of an object through a pointer. Several shared_ptr objects may own the same object, where the object is destroyed and its memory deallocated when the last shared_ptr is destroyed.
Shared pointers use a reference count that keeps track of how many pointers are pointing to a resource. Once a shared pointer stops pointing to a resource (either by going out of scope or by being assigned a new resource), the reference count is decreased. When the reference count reaches zero, the memory is automatically deleted.
Example:
std::shared_ptr<int> p1(new int(42));
std::shared_ptr<int> p2 = p1;
Weak Pointers
A weak pointer is a smart pointer that holds a non-owning ("weak") reference to an object that is managed by std::shared_ptr
. It must be converted to std::shared_ptr
in order to access the object.
Weak pointers are used to prevent strong reference cycles which could lead to memory leaks. They're not commonly used because they don't solve general problems, and behave somewhat like raw pointers.
Example:
std::shared_ptr<int> p1(new int(42));
std::weak_ptr<int> p2 = p1;
In this case, p2
is a weak pointer that shares memory with p1
. However, the destruction of p1
would result in the memory being deallocated, even if p2
was still in scope. To access the memory, p2
must first be converted to a shared pointer.
Smart pointers in C++ provide a level of memory management and safety not inherent with raw pointers, reducing potential bugs and memory leaks in C++ programs.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version