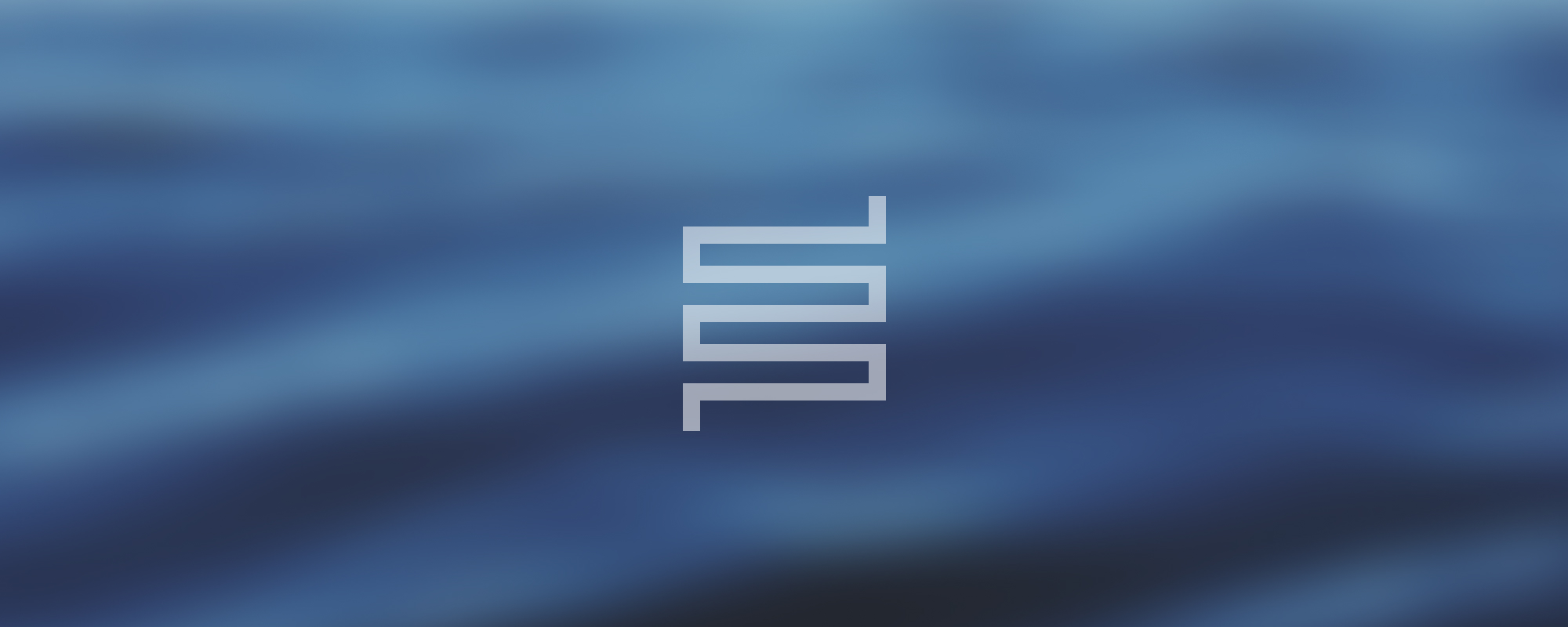
24. Standard Template Library (STL) in C++
Introduction to STL
The Standard Template Library (STL) is a powerful feature in C++ that provides predefined templates for a set of common data structures and algorithms. It includes various types of containers, algorithms, and iterators.
What is a Container?
A container is a data structure that stores data. In STL, containers are template classes, meaning they can store any data type. All containers have a begin
and end
function, which are common features. These functions are used to provide access to the start and end of the container respectively.
What is an Iterator?
An iterator is an object that enables a programmer to traverse a container, particularly lists. Different types of iterators are often provided through the container's interface. It's an intelligent class that knows how to navigate through each container.
Vector Container
A Vector is a dynamic array with the ability to resize itself automatically when an element is inserted or deleted. It works like an array and we can use the index to access elements.
Key Points:
- Vectors use dynamic memory allocation. If the size of the vector is increased, it creates a new array and copies the old values, then deletes the old array.
- It provides us with the ability to work with the size of the array according to our needs.
- To use vectors, include the vector library:
#include <vector>
.
Example of Vector in C++
#include <iostream>
#include <vector>
int main() {
// Declare a vector
std::vector<int> vec;
// Add elements to the vector
vec.push_back(1);
vec.push_back(2);
vec.push_back(3);
// Use iterator to access the values
for (std::vector<int>::iterator it = vec.begin(); it != vec.end(); ++it)
std::cout << ' ' << *it;
return 0;
}
List Container
A List is a container that supports constant time insertions and deletions from anywhere in the sequence. It is implemented as a doubly-linked list.
Example of List in C++
#include <iostream>
#include <list>
int main() {
// Declare a list
std::list<int> lst;
// Add elements to the list
lst.push_back(1);
lst.push_back(2);
lst.push_back(3);
// Use iterator to access the values
for (std::list<int>::iterator it = lst.begin(); it != lst.end(); ++it)
std::cout << ' ' << *it;
return 0;
}
In conclusion, STL in C++ is a powerful library that provides a set of common data structures (like vectors and lists) and algorithms with template classes, allowing for more efficient, rapid, and safer code development.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version