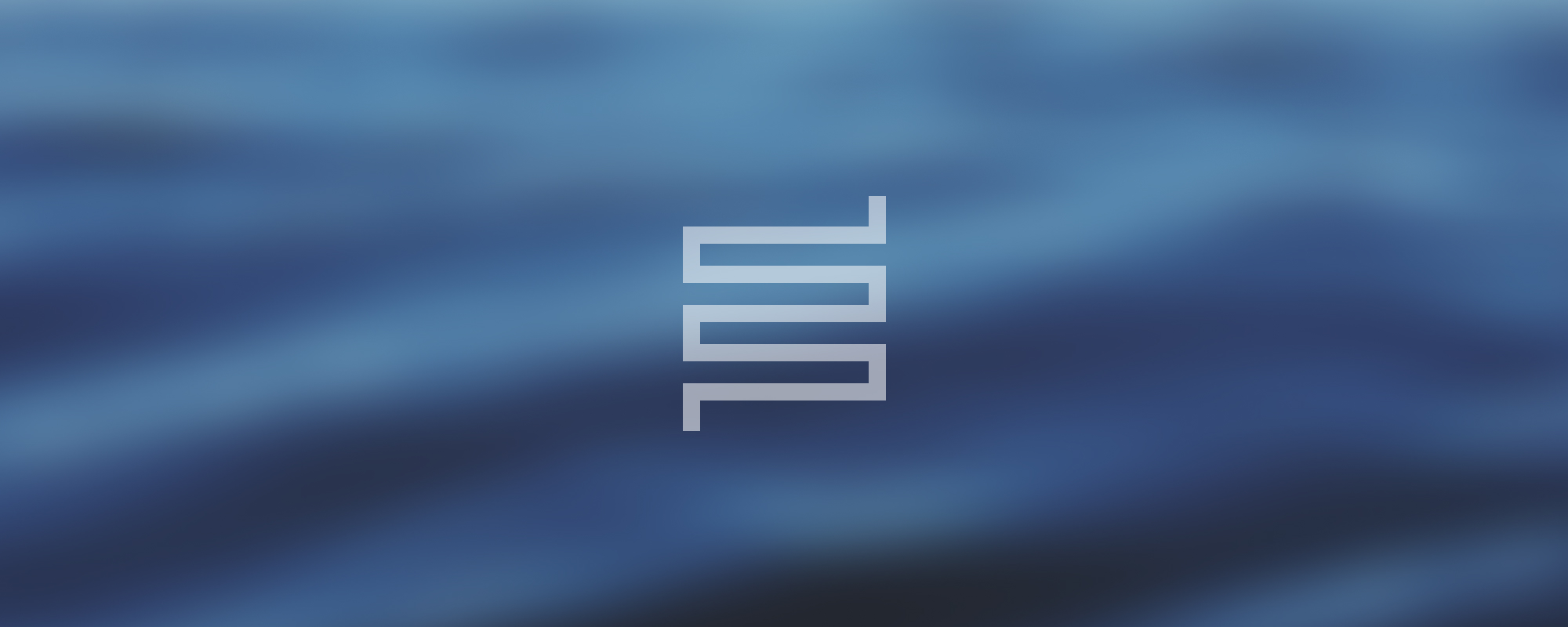
25. The Standard Template Library (STL)
The Standard Template Library (STL) provides a set of common classes for C++, such as containers and associative arrays, to handle common programming data structures like vectors and lists.
STL Containers: Overview
In Lesson 24, we discussed vectors and lists. Now, we will cover four more types of STL containers:
- set
- map
- multiset
- multimap
To use these containers, you need to include the relevant headers:
#include <set>
#include <map>
Set Containers
A set is a type of associative container that stores unique elements following a specific order. In C++, sets are typically implemented as binary search trees.
Key Points:
- Elements in a set are always sorted following a specific order.
- Each element in a set is unique.
Map Containers
A map is another type of associative container that stores elements formed by a combination of a key value and a mapped value, following a specific order. Here, the key values are unique and it serves as the index to access the corresponding mapped value. Like sets, maps are usually implemented as binary search trees.
Example:
map<string, string> ypx;
ypx["10-KY-248"] = "Range Rover";
In this example, if the key "10-KY-248" exists in the map, the value "Range Rover" will replace the existing value. If the key does not exist, a new element with this key value and mapped value will be added to the map.
Multiset Containers
A multiset is similar to a set. However, it allows multiple instances of equivalent elements. It means that multiset unlike set can have duplicate elements.
Multimap Containers
A multimap is similar to a map, but it can have duplicate keys. This means that multimap can have multiple pairs with equivalent keys.
Note:
- You cannot access multimap elements with an index. For example,
cout << multimap["AUDI"] << endl;
would result in an error.
STL List and Forward List
#include "list" // For a doubly-linked list that supports navigation in both directions.
#include <forward_list> // For a singly-linked list that supports navigation in only one direction.
Predicates
A predicate is a function that returns a boolean value. Predicates can be used in sorting a list.
Example:
bool byNameAsc(const Kitty& k1, const Kitty& k2) // - Predicate
{
return k1.GetName() < k2.GetName();
}
bool byAgeAsc(const Kitty& k1, const Kitty& k2) // - Predicate
{
return k1.GetAge() < k2.GetAge();
}
void main()
{
list<Kitty> kitties;
kitties.push_back(Kitty("Toplan", "Toplan123", 35));
kitties.push_back(Kitty("Mestan", "Qara", 12));
kitties.push_back(Kitty("Black", "Jav Jav", 67));
kitties.push_back(Kitty("Garfield", "Hungry Cat", 16));
PrintKitties(kitties);
kitties.sort(byNameAsc);
cout << endl;
PrintKitties(kitties);
kitties.sort(byAgeAsc);
cout << endl;
PrintKitties(kitties);
}
In this example, we first sort the list of kitties by name ascendingly. Then, we sort the list by age ascendingly.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version