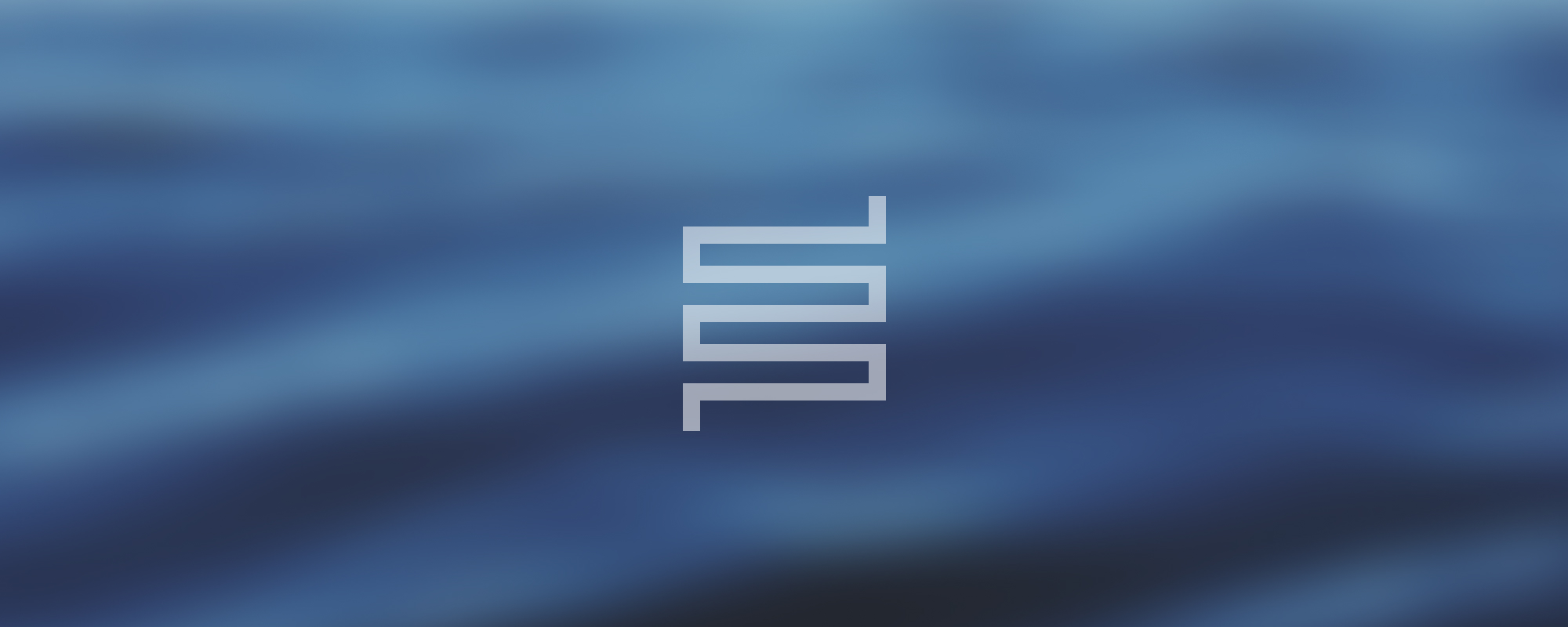
26. Functors and Algorithms
A functor (function object) in C++ is a class or struct that can act like a function. This is achieved by defining the operator()
of a class.
Functors can be used to encapsulate a function that takes one or more arguments. They can be passed as arguments to other functions or used as objects of a class.
Defining a Functor
To define a functor, we overload the operator()
in a class. Here is an example of a functor that compares the ages of different Kitty
objects:
class byAge
{
bool order;
public:
byAge(bool order) : order(order)
{
}
bool operator()(const Kitty& cat1, const Kitty& cat2)
{
if (order)
{
return cat1.GetAge() < cat2.GetAge();
}
return cat1.GetAge() > cat2.GetAge();
}
};
void main()
{
kitties.sort(byAge(false));
}
In this example, byAge
is a functor that can be used to sort Kitty
objects by age.
Predicates in C++
Predicates are functions that return a boolean value. They are often used with functors and algorithms to perform operations based on certain conditions.
Functors can be used in place of multiple predicates, leading to cleaner and more organized code.
Algorithms in C++
C++ provides several algorithms in the <algorithm>
and <numeric>
libraries that can be used with containers and functors to perform various operations.
transform()
The transform()
function traverses a container and performs an operation based on a given functor or predicate.
accumulate()
The accumulate()
function, included in the <numeric>
library, performs a specified operation at the end (default operation is addition).
count()
The count()
function returns the number of occurrences of a specified data in a vector.
find()
The find()
function returns the address of a specified data if found.
binary_search()
The binary_search()
function returns true
if a specified data is found, or false
otherwise. The vector must be sorted before using this function.
for_each()
The for_each()
function traverses a vector and performs a specified operation:
for_each(v.begin(), v.end(), print);
find_if()
The find_if()
function returns the element in a container that satisfies a specific condition:
// Returns element that is less than 10
auto p = find_if(v.begin(), v.end(), [](int data)->bool {
return data < 10;
});
count_if()
The count_if()
function returns the count of elements in a container that satisfy a specific condition:
int c = count_if(s.begin(), s.end(), [](Student s) {
return s.GetScore() > 90;
});
sort()
The sort()
function sorts a container based on a specified condition:
sort(v.begin(), v.end(), [](int a, int b)->bool {
return a > b;
});
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version