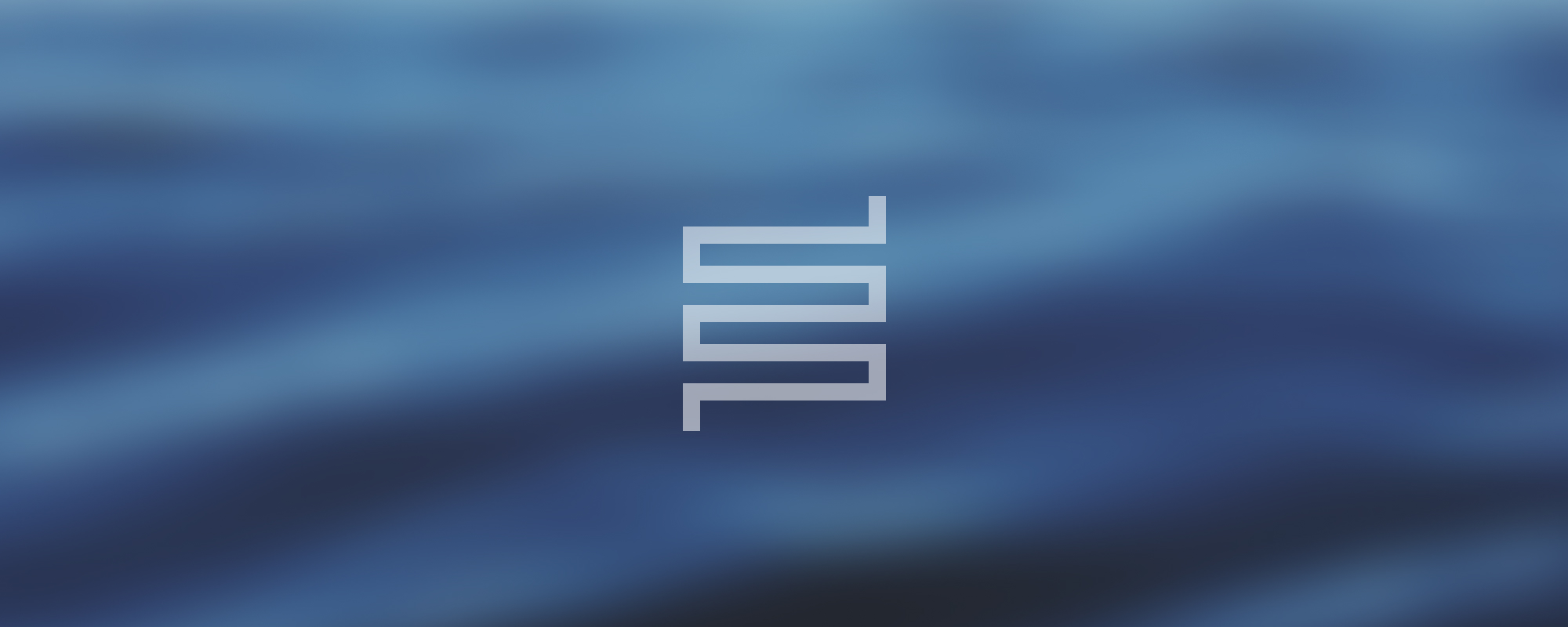
27. Understanding Namespaces
Introduction to Namespaces
A namespace is a declarative region that provides a scope to the identifiers (names of types, functions, variables, etc.
) inside it. Namespaces are used to organize code into logical groups and to prevent name collisions that can occur especially when your code base includes multiple libraries.
Key Points:
- Namespaces are used to group related code.
- They prevent naming conflicts or collisions.
- They enhance code readability.
Global Namespace
In C++, codes written without a namespace are said to be in the global namespace. This means every entity can see each other in this space.
// This function is in the global namespace
void func() {
// function code
}
Using the 'std' Namespace
The std
namespace contains all the classes, objects and functions of the C++ Standard Library. By declaring using namespace std
, you're telling the compiler to assume that you're using the std
namespace.
namespace std {
// The std namespace contains all the standard library code
}
If the std
namespace already exists, any code you write inside this scope supplements the existing std
namespace. If it doesn't exist, a new one is created.
Creating a Namespace
A namespace is created by using the namespace
keyword followed by the name of the namespace. Here's an example:
namespace Network
{
namespace HTTP
{
namespace HttpClient
{
class HttpClientRequest
{
};
}
}
}
In this example, we have created a nested namespace where Network
contains HTTP
which further contains HttpClient
.
Using a Namespace
To use the code in a namespace, we need to use the scope resolution operator ::
.
void main()
{
Network::HTTP::HttpClient::HttpClientRequest client;
}
Namespace Aliases
In C++, we can create an alias for a namespace using namespace alias = current::namespace::name
. Namespace aliases can make code more readable and easier to type, especially for lengthy nested namespaces.
// Creating Namespace Alias
namespace NHH = Network::HTTP::HttpClient;
void main()
{
// Using Namespace Alias
NHH::HttpClientRequest client2;
}
In this example, NHH
is an alias for Network::HTTP::HttpClient
, which can be used as a shortcut when declaring objects of the HttpClientRequest
class.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version