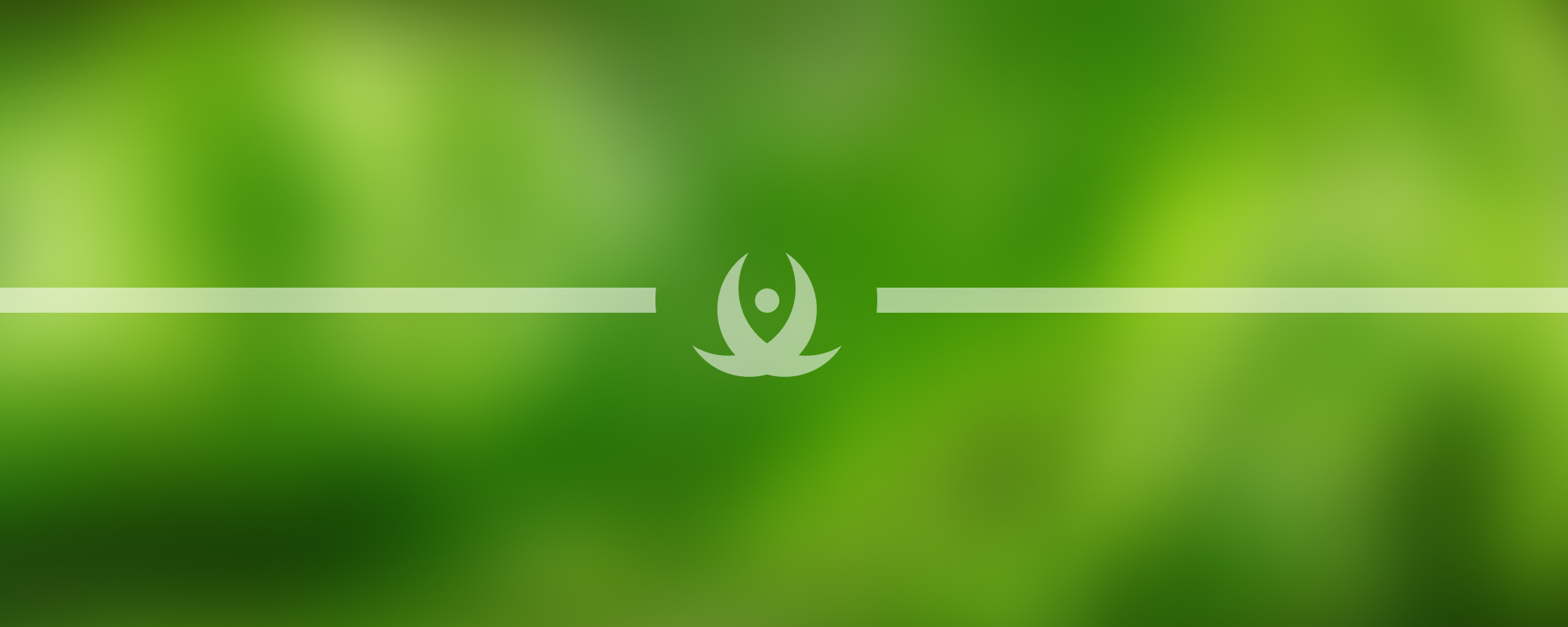
1. Introduction to C#
Overview of C# Basic Concepts
C# is a fully object-oriented programming language that supports various data types and programming paradigms. Below are key points concerning data types, anonymous types, string interpolation, and type conversion.
Data Types in C#
C# offers four major categories of data types:
- Value Types (stored on the stack)
- Examples:
bool
,char
,byte
,decimal
,double
,float
,int
,long
, etc.
- When you pass a value type to a method or assign it to another variable, its copy is passed or assigned.
decimal
is more precise thandouble
(e.g.,decimal d = 1.2m;
).
byte
can store values from 0 to 255.
- Examples:
- Reference Types (stored on the heap)
- When you pass or assign a reference type, the reference (not the copy) is passed.
- Example: Classes, arrays, etc.
- Generic Types
- Templates or type parameters (e.g.,
List<T>
,Dictionary<TKey, TValue>
).
- Templates or type parameters (e.g.,
- Pointer Types
- Less commonly used in high-level C#; often requires
unsafe
context for direct memory manipulation.
- Less commonly used in high-level C#; often requires
Note:
- Everything in a class is allocated on the heap.
- Everything in a struct is allocated on the stack.
Anonymous Types (and Related Concepts)
var
- Similar to
auto
in C++.
- The compiler infers the type at compile time.
- Because the compiler knows the type, methods and properties become available via IntelliSense when you type
.
(dot).
- Similar to
dynamic
- The compiler resolves the type at runtime.
- IntelliSense does not show methods or properties when you type
.
(dot), because the type isn’t determined until the program is running.
String Interpolation and Verbatim Strings
Instead of concatenating strings like:
// Console.WriteLine("First : " + number1 + "Second : " + number2);
You can use interpolation with the $
symbol:
Console.WriteLine($"First : {number1} Second : {number2}");
To include multiline text or preserve formatting, use the verbatim symbol @
in conjunction with $
:
int age = 12;
Console.WriteLine($@"
Hello
This program's age is {age}
Developer
C++
C#
");
This approach keeps the original formatting, including line breaks and whitespace.
Converting Data
Convert
- Methods like
Convert.ToInt32(string)
convert strings to numerical types.
- If conversion fails, an exception is thrown.
- Methods like
Parse
- Methods like
int.Parse(string)
also throw an exception on failure.
- Methods like
TryParse
- Methods like
int.TryParse(string, out int result)
return a boolean (true if successful, false otherwise).
- If the conversion is successful, the result is stored in the
result
variable; if not, you can handle it gracefully.
- Methods like
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version