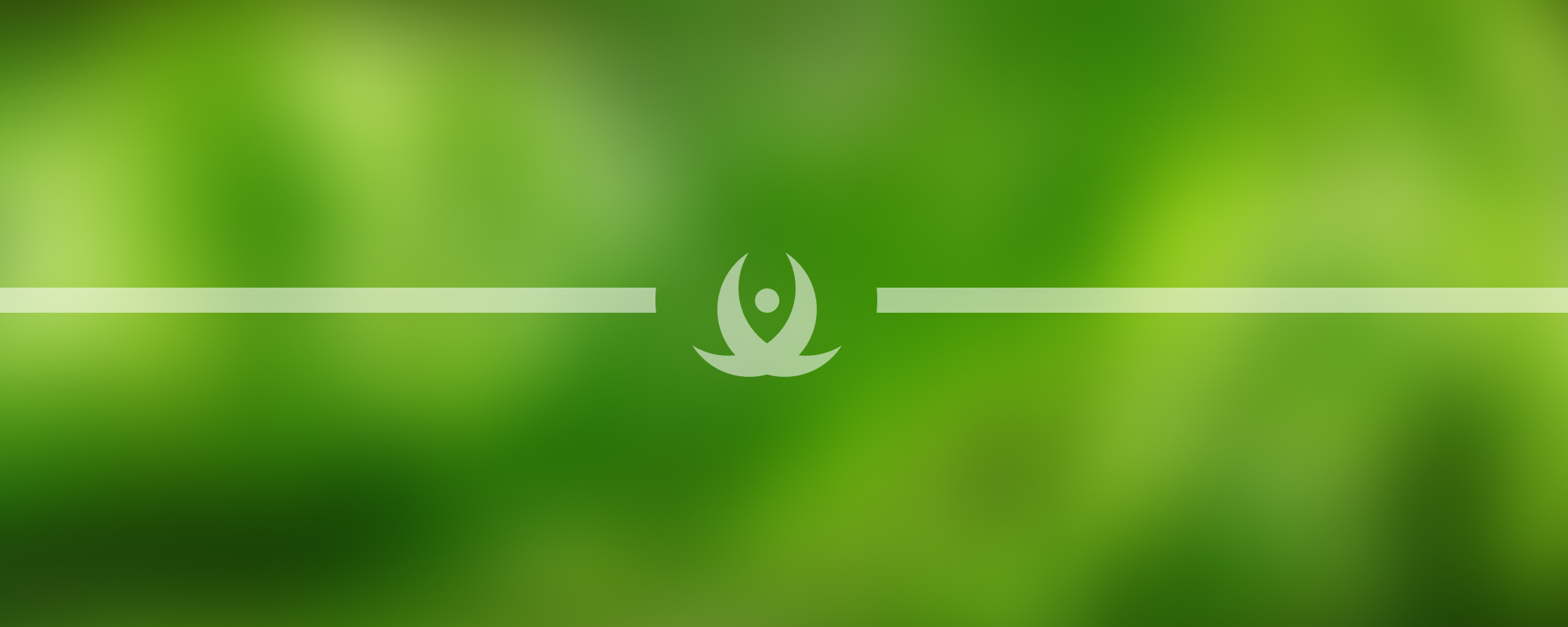
10. Interfaces
Custom Exception Classes
By deriving from ApplicationException
(or more commonly directly from Exception
), you can create custom exception classes that add contextual information specific to your application’s needs. This allows for clearer error reporting and handling.
Example:
public class MyCustomException : ApplicationException
{
public MyCustomException() { }
public MyCustomException(string message) : base(message) { }
public MyCustomException(string message, Exception inner) : base(message, inner) { }
}
You can then throw and catch MyCustomException
wherever a specialized error condition occurs:
if(someConditionFailed)
{
throw new MyCustomException("Something went wrong with my custom logic.");
}
Custom exceptions allow you to provide additional data or context for debugging or logging.
Interfaces
An interface in C# defines a contract that implementing classes must fulfill. All methods in an interface are implicitly abstract (no method bodies), and the interface cannot be instantiated directly.
Why Use Interfaces?
- Multiple Inheritance of Behavior:
A class can only inherit from one base class, but it can implement multiple interfaces. This allows you to combine various capabilities or contracts into a single class.
- Contract Enforcement:
Interfaces ensure that any implementing class provides certain methods (and optional properties/events). Clients can rely on those methods existing without caring about the underlying implementation.
- Loose Coupling:
Code that uses an interface is independent of specific class implementations, promoting flexibility and maintainability.
Basic Syntax
public interface ILogger
{
void Log(string message);
string LogFilePath { get; set; }
}
public class FileLogger : ILogger
{
public string LogFilePath { get; set; }
public FileLogger(string filePath)
{
LogFilePath = filePath;
}
public void Log(string message)
{
// Implementation that writes the log to a file
File.AppendAllText(LogFilePath, message + Environment.NewLine);
}
}
- A class must implement all members declared in the interface.
- Interface members are implicitly public and abstract; you cannot use access modifiers on interface members.
- An interface cannot have constructors or instance fields; you can only declare methods, properties, indexers, and events.
Implementing Multiple Interfaces
A class can implement multiple interfaces:
public class MultiTool : ITool, IDevice
{
// Must provide implementations for all methods/properties in ITool and IDevice
}
Interface as a Base Class?
While you can technically list an interface in a base-class-like position, interfaces are typically used to supplement or extend the functionality of an existing base class. A class can inherit from one base class and implement multiple interfaces.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version