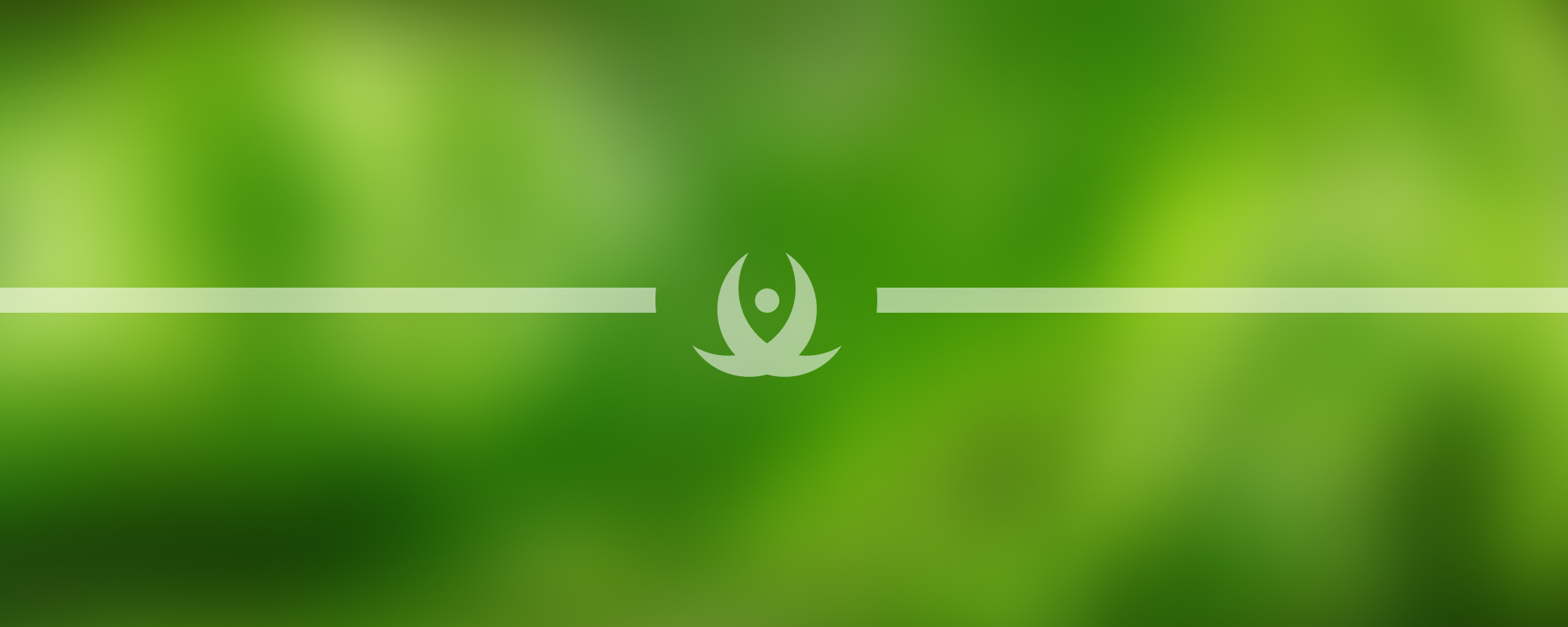
16. Serialization
XML Files and Serialization in C#
XML Files
XML (Extensible Markup Language) is a text-based data format often used for configuration, data exchange, or storage. It organizes data into nested tags, making it both human-readable and machine-readable.
<Person>
<Name>John Doe</Name>
<Age>30</Age>
</Person>
You can parse or generate XML in C# using classes such as:
XmlDocument
(older, DOM-based model)
XDocument
andXElement
in LINQ to XML (more modern and convenient)
XmlSerializer
for object serialization
Serialization
Serialization is the process of converting an object’s in-memory state into a format (e.g., binary, XML, JSON) that can be stored or transmitted. The reverse process is called deserialization, converting the format back into an object instance.
Why Serialize?
- Persisting Data: Storing an object’s state to disk or database.
- Communication: Sending objects over a network, such as via web services.
- Caching: Quickly saving object states for later retrieval.
[Serializable]
Attribute
In .NET, applying [Serializable]
to a class indicates that instances of that class can be serialized. This is often used for binary or SOAP serialization. For XML or JSON serialization, a class typically doesn’t require [Serializable]
; however, it might need other attributes (e.g., [XmlElement]
, [JsonProperty]
, etc.) depending on the serializer used.
[Serializable]
public class Person
{
// Only properties are serialized by default
public string Name { get; set; }
public int Age { get; set; }
}
Serialization Target
- Memory: Serialize to a
MemoryStream
for in-memory data transfer.
- File: Serialize to a
FileStream
for persistent storage.
- Network: Send serialized data over TCP/HTTP.
XML Serialization in C#
Using XmlSerializer
from the System.Xml.Serialization
namespace, you can serialize an object to XML and back. By default, public properties are included.
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
// Serializing
XmlSerializer xmlSerializer = new XmlSerializer(typeof(Person));
using (FileStream fs = new FileStream("person.xml", FileMode.Create))
{
var john = new Person { Name = "John Doe", Age = 30 };
xmlSerializer.Serialize(fs, john);
}
// Deserializing
using (FileStream fs = new FileStream("person.xml", FileMode.Open))
{
Person loadedPerson = (Person)xmlSerializer.Deserialize(fs);
Console.WriteLine($"{loadedPerson.Name}, {loadedPerson.Age}");
}
JSON Files
JSON (JavaScript Object Notation) is a lightweight, text-based format. It’s frequently used in modern applications for data transfer, especially with web APIs.
{
"Name": "John Doe",
"Age": 30
}
JSON Serialization in C#
In .NET, you can use System.Text.Json or Newtonsoft.Json for JSON serialization:
System.Text.Json example:
using System.Text.Json;
var person = new Person { Name = "John Doe", Age = 30 };
// Serialize to string
string jsonString = JsonSerializer.Serialize(person);
Console.WriteLine(jsonString);
// Deserialize
Person deserializedPerson = JsonSerializer.Deserialize<Person>(jsonString);
Console.WriteLine($"{deserializedPerson.Name}, {deserializedPerson.Age}");
Key Points
- Properties Only: By default, serialization frameworks focus on serializing public properties, not private fields.
- Format Choice: XML, JSON, and binary each have trade-offs in readability, size, and interoperability.
- Backwards Compatibility: When changing class definitions, ensure version compatibility or specify custom rules so that previously serialized data can still be deserialized.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version