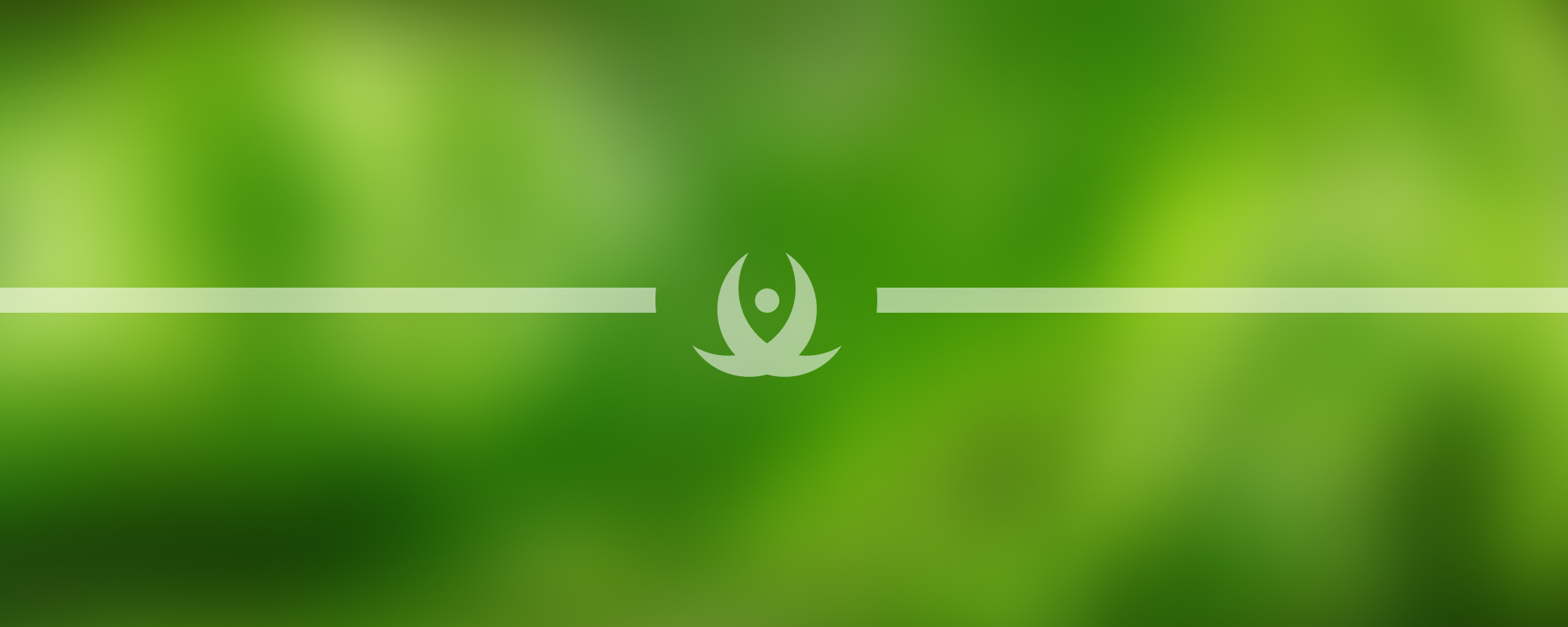
2. Functions and String
String and StringBuilder
C# provides both string
(lowercase s
) and String
(uppercase S
from System.String
) to represent text. These two names refer to the same type. However, string
in C# behaves like a value type in that it is immutable (once created, its content cannot be changed). If you need a mutable string, you can use StringBuilder
, which is a reference type and allows modifying the text without creating new objects.
Value vs. Reference Types
- Value Types (e.g.,
int
,bool
,struct
):Stored on the stack. When passed to a method or assigned to a new variable, a copy of the data is made. They are inherently immutable in that reassigning them creates a new copy.
- Reference Types (e.g.,
class
,array
,string
,StringBuilder
):Stored on the heap. When you pass or assign them, the reference is shared, meaning changes affect the original object. Most reference types are mutable, but
string
is a special reference type that is immutable by design.
Enums
Enums (enum
) are sets of named integer constants that improve code readability. For example:
enum Days { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday }
Nullability
Only reference types can be null
by default in C#. For value types (int
, bool
, etc.), you must explicitly use nullable notation to allow null
:
int? data = null;
ref, out, and in Keywords
- ref
Passes a parameter by reference, requiring that the variable has been assigned a value before it is passed. Changes in the called method affect the original variable.
- out
Similar to
ref
, but the variable is not required to have an initial value. The called method must assign a value before returning.
- in
Passes a parameter by reference but as read-only (a
const
reference). The called method cannot modify the original variable.
checked and unchecked
In C#, arithmetic operations can overflow, leading to unexpected results. The checked
keyword enforces overflow checking at runtime, throwing an exception if an operation exceeds the data type’s range. Conversely, unchecked
ignores overflow, potentially yielding truncated or incorrect values without an exception.
checked Example
checked
{
int val = int.MaxValue;
Console.WriteLine(val + 2); // Throws System.OverflowException
}
unchecked Example
unchecked
{
int val = int.MaxValue;
Console.WriteLine(val + 2); // Outputs a negative number (-2147483647)
}
When neither checked
nor unchecked
is specified, the default behavior depends on compiler settings (checked
for constants in some cases, unchecked
for non-constants by default).
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version