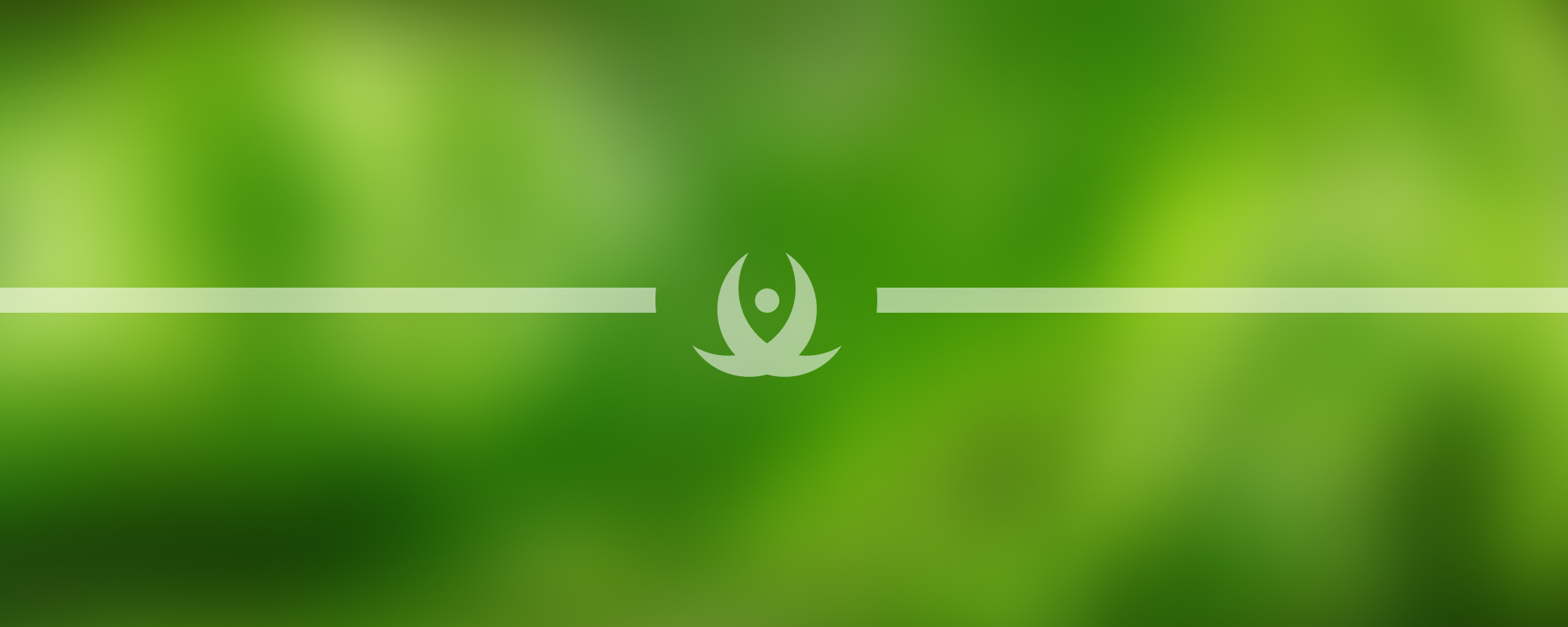
3. Arrays
Arrays in C#
Arrays are collections of elements that share the same data type. In C#, arrays are reference types stored in the heap. Passing an array to a method sends the reference, meaning any change made inside the method affects the original array.
Declaration and Initialization
// Shorthand
string[] students = { "Rocky", "Arif", "Leyla" };
// Long form
string[] students2 = new string[] { "Rocky", "Arif", "Leyla" };
Both forms create an array of string
elements. Since they are reference types, they are allocated on the heap.
Array Properties and Methods
array.Length
Provides the number of elements in the array. Internally, it’s accessed via a getter.
Cloning an Array
Clone()
returns a new object with the same contents at a different memory location.
string[] students = { "Rocky", "Arif", "Leyla" };
string[] stud_clone = students.Clone() as string[];
students[0] = "Salam";
if (stud_clone != null)
{
foreach (string student in stud_clone)
{
Console.WriteLine(student);
}
}
In the above example:
students.Clone()
returns a new object identical tostudents
.
as string[]
attempts to cast the clone to astring[]
, returning null if it cannot.
- Changes in
students
do not affectstud_clone
after the clone is made.
Alternatively, you can use the is pattern:
string[] students = { "Rocky", "Arif", "Leyla" };
var obj = students.Clone();
if (obj is string[] stud_clone)
{
foreach (var item in stud_clone)
{
Console.WriteLine(item);
}
}
else
{
Console.WriteLine("I cannot convert");
}
Copy Method
Copy()
is a string method that returns a new String
object with the same characters but a different object reference.
string str1 = "Hello";
string str2 = string.Copy(str1);
// str1 == "Hello", str2 == "Hello", but str1 and str2 refer to different objects
Multidimensional Arrays
Rectangular Arrays
All rows must have the same number of columns.
int[,] rectangleArray = new int[3, 2]
{
{1,2},
{3,4},
{5,6}
};
Jagged Arrays
An array of arrays, allowing each row to have a different length.
int[][] jaggedArray = new int[3][];
jaggedArray[0] = new int[2] { 1, 2 };
jaggedArray[1] = new int[3] { 3, 4, 5 };
jaggedArray[2] = new int[1] { 6 };
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version