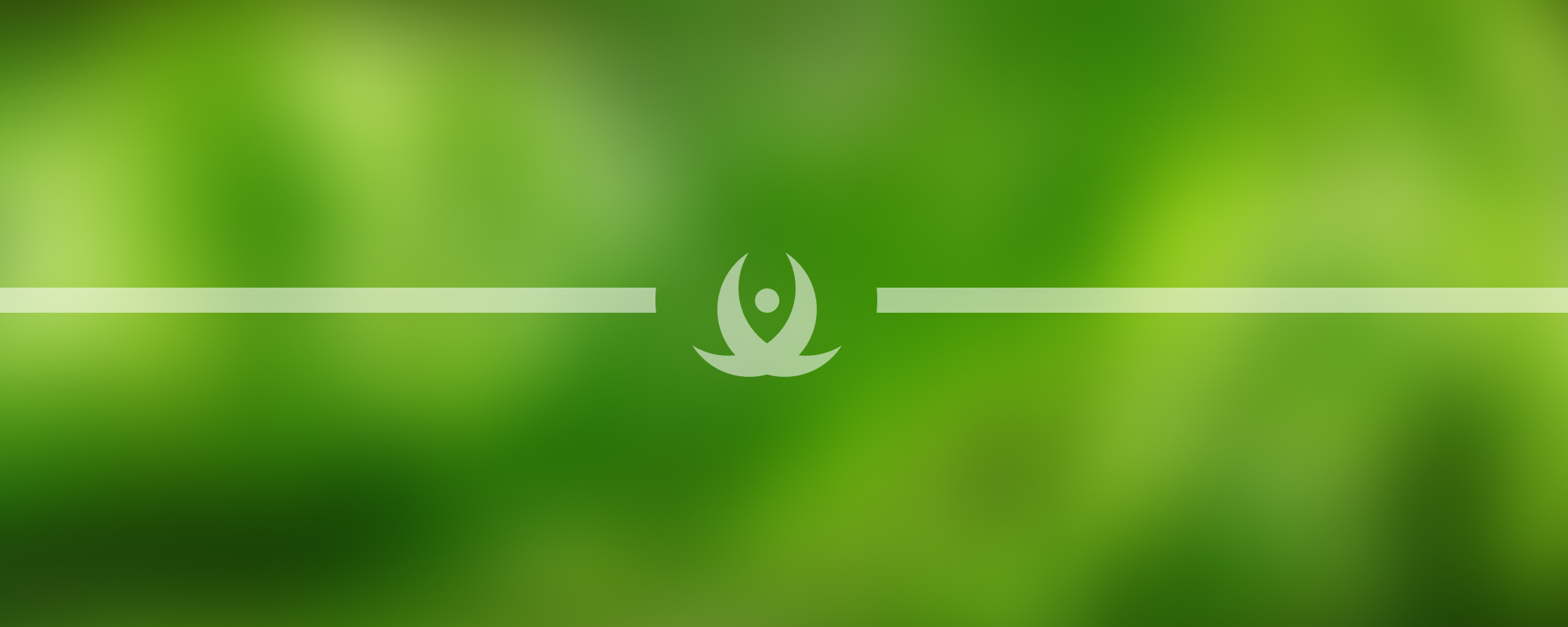
4. Struct, Classes
CultureInfo Class
CultureInfo is a class in the System.Globalization namespace that provides information about cultural conventions, such as currency formats, country codes, and other locale-specific details.
Struct vs. Class
A struct is a value type stored on the stack, while a class is a reference type stored on the heap.
- Struct
- Most built-in C# types like
int
,bool
, anddecimal
are structs.
- When you create an array of structs, each element automatically holds a default (often zero-based) value.
- Most built-in C# types like
- Class
- Arrays, strings, and complex objects are classes (reference types).
- When you create an array of a class type, each element initially contains
null
and must be instantiated withnew
before use.
Other differences include:
- No inheritance from one struct to another.
- A struct has no user-defined default constructor; a class can have one.
- Struct is the base concept for value types; class is the base concept for reference types.
Equals vs. ReferenceEquals
C# offers two main ways to compare objects:
- Equals
- Compares values for value types.
- For reference types, it defaults to reference comparison unless overridden. Developers can override
Equals
to compare internal fields rather than references.
- ReferenceEquals
- Checks if two references point to the same object in memory.
- It cannot be overridden.
In practice:
- For reference types,
Equals
andReferenceEquals
often do the same thing by default.
==
typically callsEquals
, but you can override==
in classes or structs for custom comparison logic.
Arrow Functions in C#
If a method or property contains only a single statement, you can use arrow syntax (=>
). For example:
public int GetAge() => age;
This syntax is concise and can be applied to getters in properties or short method definitions.
Properties in C#
Properties wrap fields with get
and set
accessors.
- Auto-properties
public int Salary { get; set; }
The compiler creates a backing field automatically.
- Full properties
private int myVar; public int MyProperty { get { return myVar; } set { myVar = value; } }
Here, you explicitly define a private backing field.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version