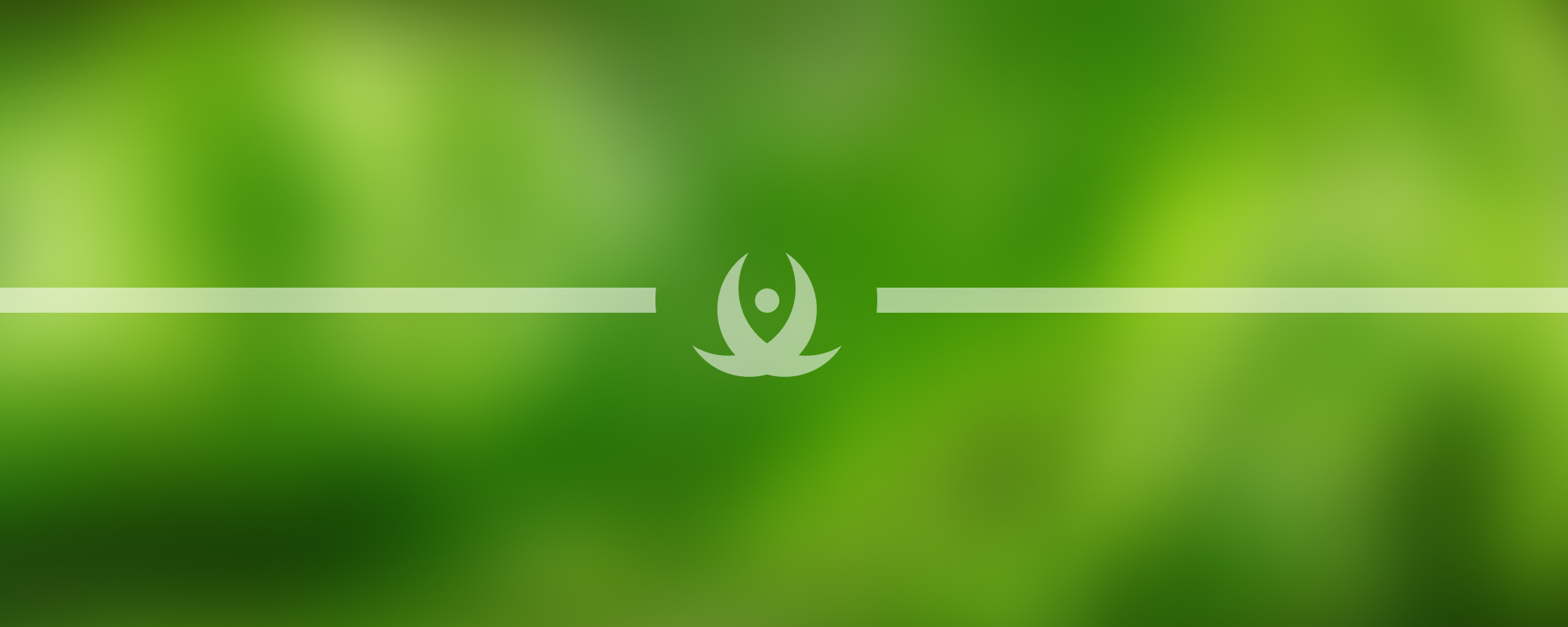
5. Static Constructor, Partial Classes
Static Constructors
A static constructor in C# is a special constructor that is called exactly once, before any object of the class is created or any static members are accessed. Even if you create 1000 objects, the static constructor runs only a single time.
- It can be useful for tasks like initializing static fields or logging when the type is first used.
- If a static constructor throws an exception, the type becomes unusable for the rest of the program execution, leading to potentially serious issues.
Partial Classes
A partial class allows you to split the definition of a class across multiple files or separate parts of the same file. Each part must declare the class as partial
, and they must reside in the same namespace (or use using
statements to reference it).
Why Use Partial Classes?
- They can help organize large classes by splitting functionality into different files.
- They can separate auto-generated code (for example, from a designer tool) from developer-written code.
Partial Methods
In C#, a partial method is a special kind of method that:
- Must be declared within a
partial
class.
- Has an implicit private accessibility and void return type (no other access modifiers or return types are allowed).
- Can be declared in one part of the partial class and optionally implemented in another part. If there is no implementation, all calls to that method are removed at compile time (no runtime overhead).
Example structure:
// File1.cs
public partial class Example
{
partial void OnSomethingHappened();
}
// File2.cs
public partial class Example
{
partial void OnSomethingHappened()
{
// Implementation
}
}
If OnSomethingHappened()
has no implementation, calls to it are compiled away.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version