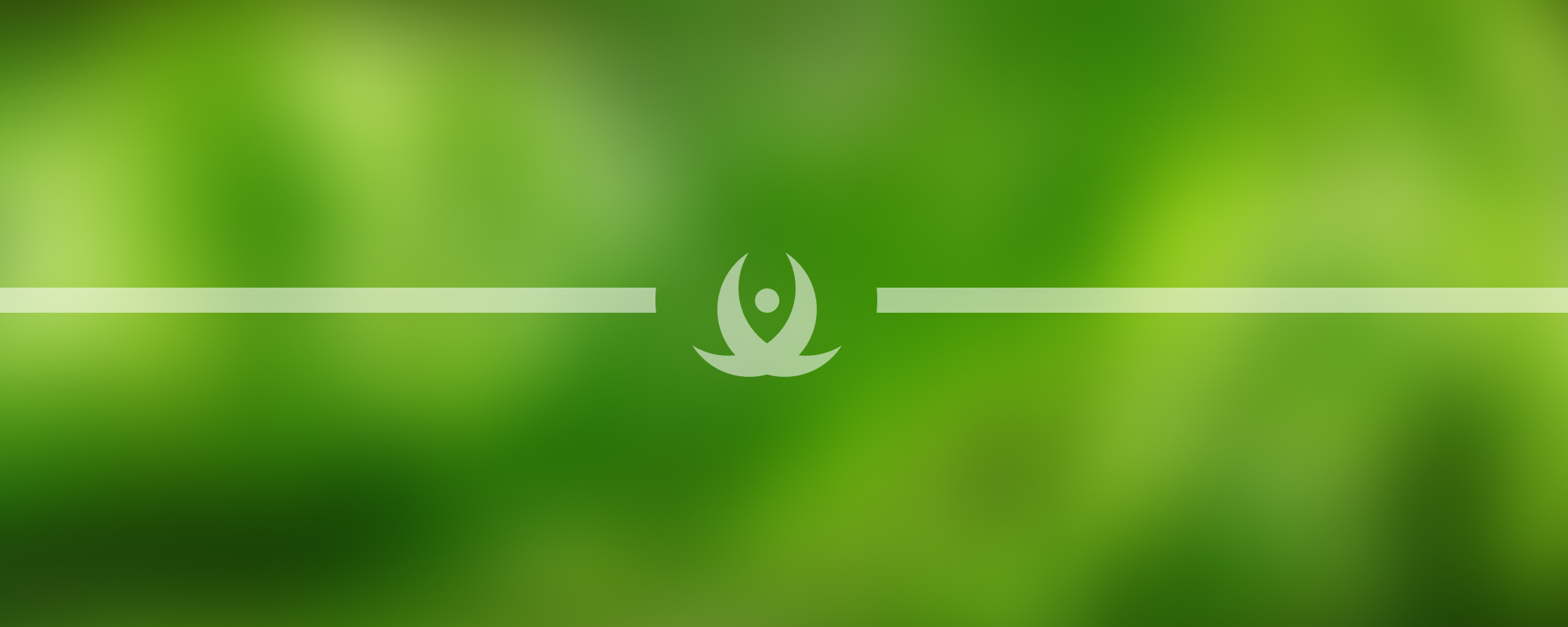
9. Abstract Method, Virtual Method
Abstract Classes and Methods in C#
What is an Abstract Class?
An abstract class is a class that cannot be instantiated directly. It serves as a blueprint for derived classes, possibly containing incomplete methods (i.e., abstract methods) that must be implemented by its subclasses. You typically mark a class abstract
when you want to enforce certain method signatures without providing a default implementation.
Key Points
- At least one method must be declared as
abstract
for a class to be abstract.
- An abstract class cannot be instantiated (e.g.,
new AbstractClass()
is not allowed).
- An abstract class can inherit from another abstract class (single inheritance in C#).
Abstract Methods
- An abstract method is a method without an implementation (no method body).
- It must be declared only inside an abstract class.
- Derived classes must override all abstract methods from the base class.
Example
public abstract class Shape
{
public abstract double GetArea(); // no body
public abstract double GetPerimeter();
}
public class Circle : Shape
{
private double _radius;
public Circle(double radius) => _radius = radius;
// Must override abstract methods
public override double GetArea() => Math.PI * _radius * _radius;
public override double GetPerimeter() => 2 * Math.PI * _radius;
}
Here, Shape
is an abstract class with abstract methods GetArea()
and GetPerimeter()
. Any derived class (e.g., Circle
) must override these methods with actual implementations.
Virtual vs. Abstract Methods
- Virtual methods have a default implementation (method body), but derived classes can opt to override them.
- Abstract methods have no default implementation. Derived classes must override them.
- Abstract methods are implicitly virtual (you do not need to mark them as virtual).
Polymorphism via Abstract Classes
When a derived class overrides abstract methods, you achieve polymorphism. You can refer to derived objects by their abstract base type and rely on the overridden methods at runtime:
Shape shape = new Circle(5.0);
Console.WriteLine($"Area: {shape.GetArea()}"); // Circle's implementation
Console.WriteLine($"Perimeter: {shape.GetPerimeter()}");
When to Use an Abstract Class
- You have a common base that should share code or default members, but also requires some methods to be customized in each subclass.
- You do not want direct instances of that base class (because it may not represent a fully realizable concept on its own).
Virtual Methods
- A virtual method has a body in the base class, but derived classes can override it if needed.
- If you want to allow but not force child classes to provide their own version, use
virtual
.
Example
public class BasePrinter
{
public virtual void Print()
{
Console.WriteLine("Base printer output");
}
}
public class FancyPrinter : BasePrinter
{
public override void Print()
{
Console.WriteLine("Fancy printer output with colors");
}
}
In this scenario, Print()
has a default implementation in BasePrinter
, but FancyPrinter
chooses to override it.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version