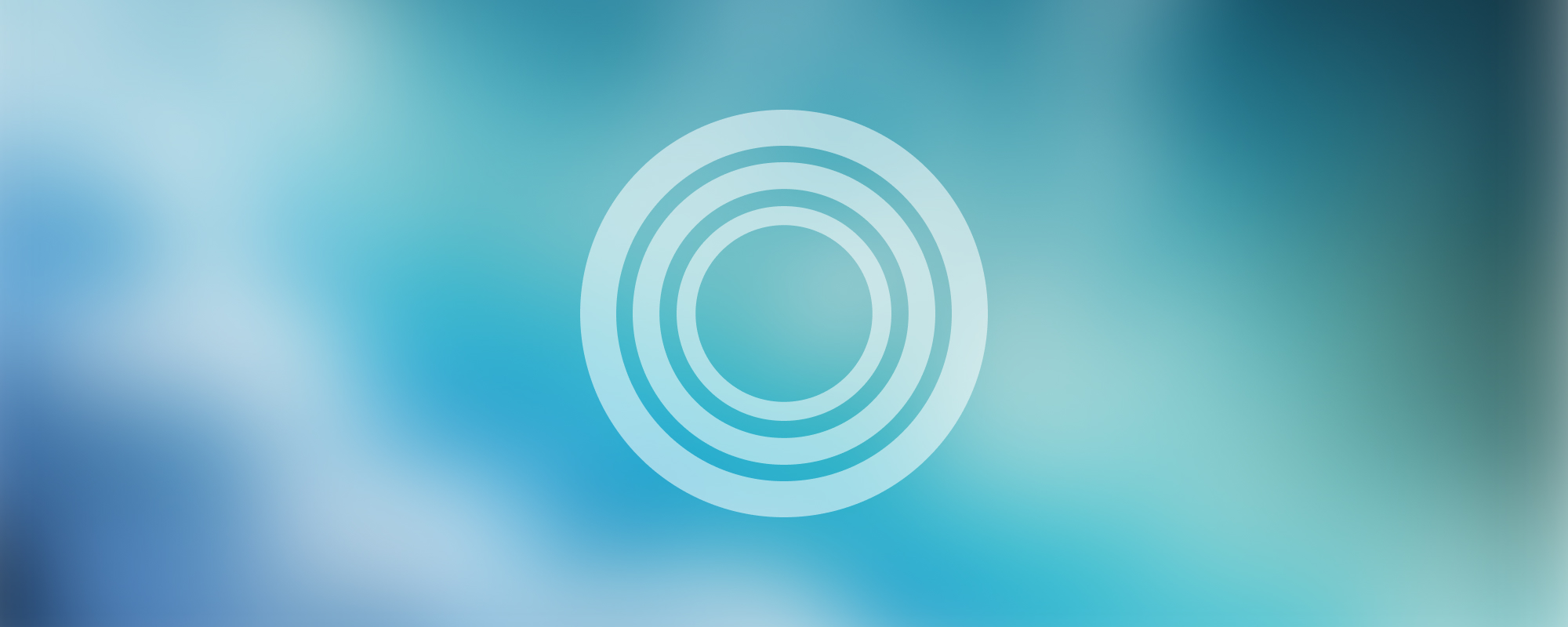
3. Adapter, Builder, Prototype Pattern
Design Patterns in Programming: Adapter, Builder, Prototype Pattern
Design patterns are general repeatable solutions to commonly occurring problems in software design. They can speed up the development process by providing tested, proven development paradigms. This note will cover three design patterns - the Adapter, Builder, and Prototype patterns.
Builder Pattern
Definition
The Builder Pattern is a creational design pattern that allows for the step-by-step construction of complex objects. This pattern enables the same construction process to create different representations and types of an object.
Explanation
In essence, the Builder Pattern separates the construction of an object from its representation. By doing so, the same construction process can be reused to build different types of objects. The Builder Pattern is a good choice when a class has too many fields, especially if many of them are optional.
Example
Below is a basic example of a Builder pattern in Java:
public class Car {
private String engine;
private int wheels;
public static class CarBuilder {
private String engine;
private int wheels;
public CarBuilder(String engine) {
this.engine = engine;
}
public CarBuilder setWheels(int wheels) {
this.wheels = wheels;
return this;
}
public Car build() {
return new Car(this);
}
}
private Car(CarBuilder builder) {
this.engine = builder.engine;
this.wheels = builder.wheels;
}
}
Prototype Pattern
Definition
The Prototype Pattern is another creational design pattern that permits the copying of existing objects without making the code dependent on their classes.
Explanation
This pattern is used when creating a duplicate instance of an existing object. Instead of creating an entirely new object from scratch and copying over each field, the Prototype pattern simply clones the existing object. It's especially useful when object creation is a costly affair.
Example
Here is an example of the Prototype pattern in Python:
import copy
class Prototype:
def __init__(self):
self._objects = {}
def register_object(self, name, obj):
self._objects[name] = obj
def unregister_object(self, name):
del self._objects[name]
def clone(self, name, **attr):
obj = copy.deepcopy(self._objects.get(name))
obj.__dict__.update(attr)
return obj
Adapter Pattern
Definition
The Adapter Pattern is a structural design pattern that allows objects with incompatible interfaces to work together.
Explanation
This pattern works as a bridge between two incompatible interfaces. It wraps itself around an object and exposes a standard interface to interact with that object. In this way, the Adapter translates requests from the format the client expects to the format the adaptee can understand.
Example
Here is a basic example of the Adapter pattern in C#:
public interface ITarget
{
string GetRequest();
}
public class Adaptee
{
public string GetSpecificRequest()
{
return "Specific request.";
}
}
public class Adapter : ITarget
{
private readonly Adaptee _adaptee;
public Adapter(Adaptee adaptee)
{
this._adaptee = adaptee;
}
public string GetRequest()
{
return $"This is '{this._adaptee.GetSpecificRequest()}'";
}
}
In this example, Adapter
makes the interface of Adaptee
compatible with the ITarget
interface.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version