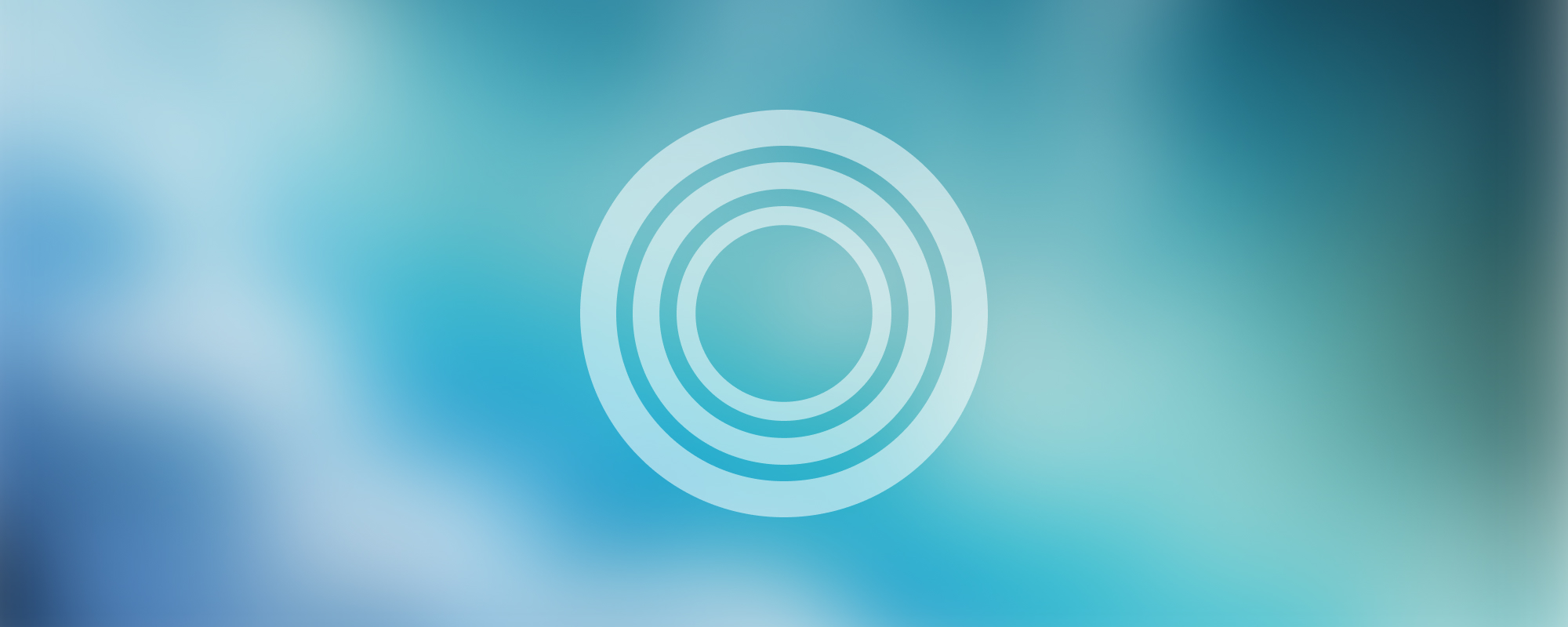
4. Proxy Pattern
Proxy Pattern in Software Design
Introduction to Proxy Pattern
The Proxy Pattern is a structural design pattern that provides a substitute or placeholder for another object. This pattern is used when you want to control access to an object, by providing a surrogate or placeholder for it. This proxy object can control the creation and access of the original object and can perform additional operations either before or after the request gets through to the original object.
Key Points of Proxy Pattern
- A proxy, in its most general form, is a class functioning as an interface to a particular object.
- Proxy objects are also called surrogates, handles, and wrappers.
- They are used for security reasons, as they can add a level of protection to the underlying object which they represent.
Proxy Pattern in Database Operations
In the context of a database operation, a proxy disguises itself as a database object. It has the ability to handle lazy initialization and result caching. The client or the real database object may not even be aware of this.
For example, when a proxy receives a number of similar queries, it stores (caches) the response. If it receives the same query again, it doesn't send a new request to the database. Instead, it reads the response from its cache, improving the efficiency and performance of database operations.
Code Example
A basic implementation of a Proxy Pattern in C# can be as follows:
public interface IDatabase
{
void Request();
}
public class RealDatabase : IDatabase
{
public void Request()
{
Console.WriteLine("Requesting data from the real database...");
}
}
public class DatabaseProxy : IDatabase
{
private RealDatabase _realDatabase;
public void Request()
{
if (_realDatabase == null)
{
_realDatabase = new RealDatabase();
}
_realDatabase.Request();
}
}
public class Client
{
public void ClientCode(IDatabase database)
{
database.Request();
}
}
Composite Pattern in Software Design
Introduction to Composite Pattern
The Composite Pattern is another structural design pattern that allows you to compose objects into tree structures and enables clients to treat individual objects and composed structures uniformly.
Key Points of Composite Pattern
- Composite Pattern is used when clients should ignore the difference between compositions of objects and individual objects.
- If you find that you are using multiple objects in the same way, and they often form a part hierarchy, a composite is a good choice.
Composite Pattern in Application
Composite Pattern is particularly useful when dealing with a hierarchical structure of objects. With the Composite Pattern, you can apply the same operations over both the individual objects and composed objects.
Code Example
A basic implementation of a Composite Pattern in C# can be as follows:
abstract class Component
{
public abstract void Operation();
}
class Leaf : Component
{
public override void Operation()
{
Console.WriteLine("Leaf operation");
}
}
class Composite : Component
{
List<Component> _children = new List<Component>();
public override void Operation()
{
foreach (var component in _children)
{
component.Operation();
}
}
public void Add(Component component)
{
_children.Add(component);
}
public void Remove(Component component)
{
_children.Remove(component);
}
}
In this example, both the Leaf
and Composite
classes inherit from the Component
class, which means they can be used interchangeably by the client. The Composite
class also contains a list of Component
objects, which can be of type Leaf
or Composite
. This allows for complex, tree-like structures to be built.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version