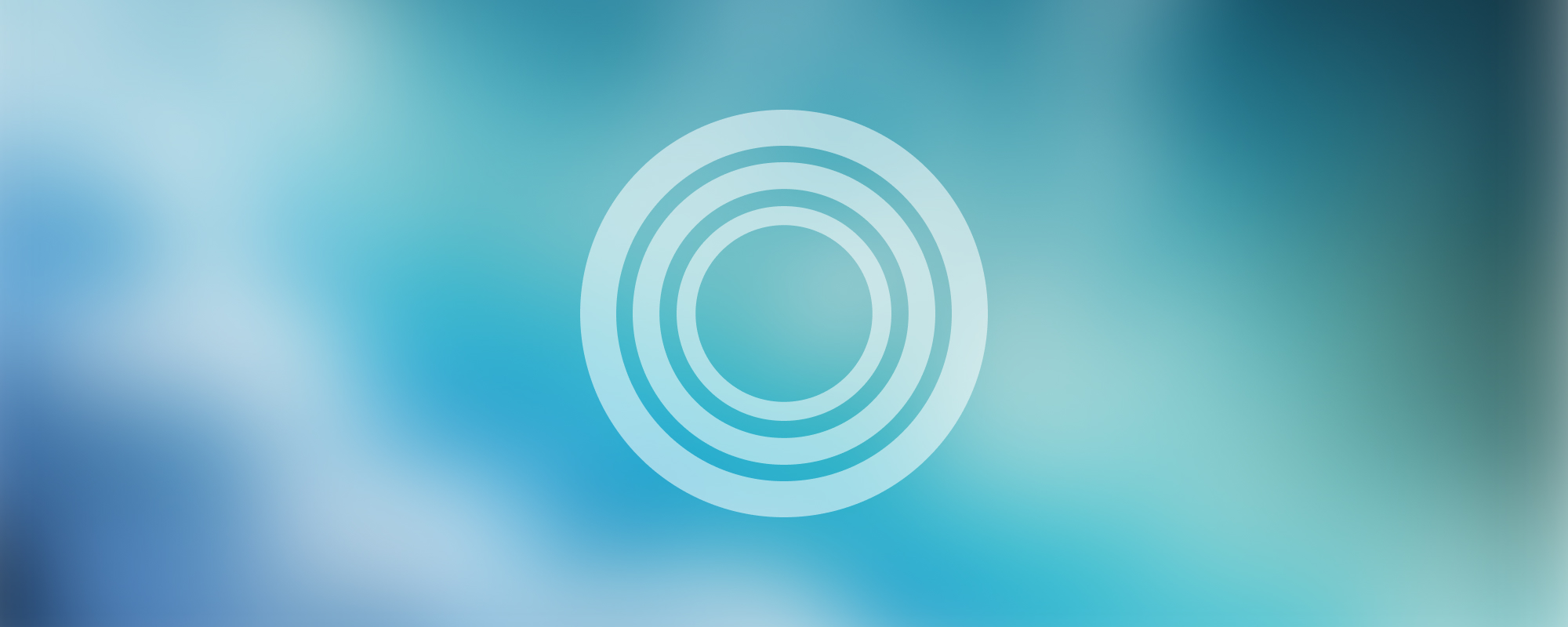
5. Flyweight, Decorator, Bridge Pattern
Design Patterns: Flyweight, Decorator, and Bridge Pattern
Flyweight Pattern
Definition
The Flyweight Pattern is a structural design pattern that optimizes memory usage by sharing common parts of state between multiple objects, rather than keeping all the data in each object. It is particularly useful when dealing with a large quantity of objects that require a significant amount of memory.
Key Points:
- It reduces the memory footprint by sharing data across objects.
- It is useful when dealing with a large number of high detail objects.
Example:
Here's an example using Python:
import json
from typing import Dict
class Flyweight():
_shared_state: Dict[str, str] = {}
def __init__(self, shared_state: str) -> None:
self._shared_state = json.loads(shared_state)
def operation(self, unique_state: str) -> None:
s = json.dumps(self._shared_state)
print(f"Flyweight: Displaying shared ({s}) and unique ({unique_state}) state.")
Bridge Pattern
Definition
The Bridge Pattern is a structural design pattern that decouples an abstraction from its implementation, so they can be developed independently. It allows splitting a large class or set of closely related classes into two separate hierarchies—abstraction and implementation.
Key Points:
- It promotes loose coupling between the abstraction and its implementation.
- It allows for the pieces to be developed independently.
Example:
Here's an example using Java:
interface Workshop {
void work();
}
class Produce implements Workshop {
public void work() {
System.out.print("Produced");
}
}
class Assemble implements Workshop {
public void work() {
System.out.print(" And");
System.out.println(" Assembled.");
}
}
abstract class Vehicle {
protected Workshop workShop1;
protected Workshop workShop2;
protected Vehicle(Workshop workShop1, Workshop workShop2) {
this.workShop1 = workShop1;
this.workShop2 = workShop2;
}
abstract public void manufacture();
}
Decorator Pattern
Definition
The Decorator Pattern is a structural design pattern that allows adding new behaviors to objects by placing these objects inside special wrapper objects that contain the behaviors. This pattern avoids modifying existing code and enhances the functionality of an object at runtime.
Key Points:
- It provides a flexible alternative to subclassing for extending functionality.
- It adds behavior to individual objects, not to the entire class.
Example:
Here's an example using JavaScript:
class Book {
constructor(title, author, price) {
this._title = title;
this._author = author;
this._price = price;
}
getDetails() {
return `${this._title} by ${this._author}`;
}
getPrice() {
return this._price;
}
}
class DiscountedBook {
constructor(book, discount) {
this._book = book;
this._discount = discount;
}
getDetails() {
return this._book.getDetails();
}
getPrice() {
return this._book.getPrice() * (1 - this._discount);
}
}
In this example, DiscountedBook
is a decorator class that adds a discount to the price of the book without modifying the original Book
class.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version