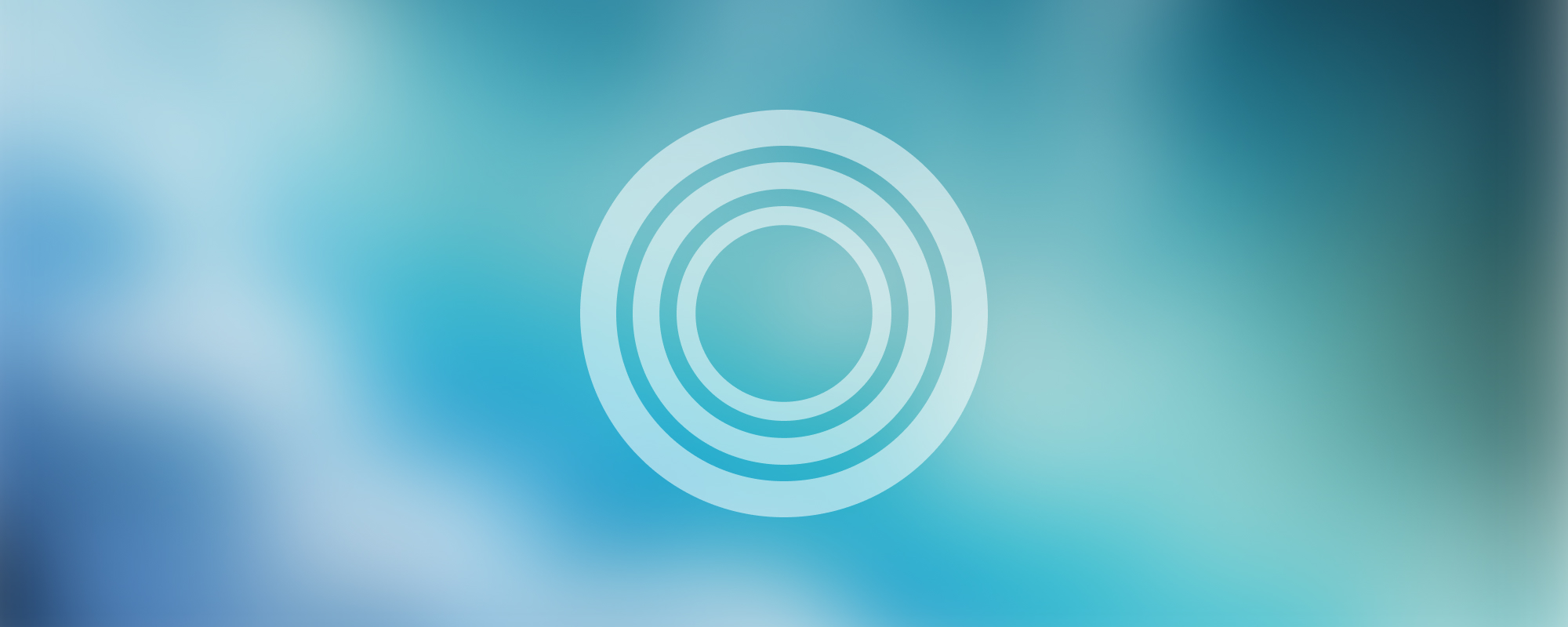
6. Facade Pattern
Facade Pattern in Software Design
Introduction to Facade Pattern
The Facade Pattern is a structural design pattern that provides a simplified interface to a complex subsystem. This pattern involves a single wrapper class that contains a set of members required by the client. These members access the system on behalf of the facade user, hiding the complexities of the larger system and providing a simpler interface to the client.
Detailed Explanation of Facade Pattern
The Facade Pattern is named aptly as it resembles a facade on a building, which hides the complexity of the building's structure and only presents a neat and organized interface to the outside world. Similarly, this pattern hides the complexities of a system and provides a simpler interface to the client.
Key Points:
- The Facade Pattern simplifies the interface of a complex system.
- It hides the complexities of a system behind a facade, making the system easier to use.
- A facade improves the efficiency and usability of a system by wrapping complex subsystem code into a single interface.
Example of Facade Pattern
Here's a simple code example in Java:
class ComplexSystem {
void operation1() { ... }
void operation2() { ... }
}
class Facade {
ComplexSystem cs = new ComplexSystem();
void simplifiedOperation() {
cs.operation1();
cs.operation2();
}
}
class Client {
public static void main(String[] args) {
Facade facade = new Facade();
facade.simplifiedOperation();
}
}
Behavioral Pattern in Software Design
Introduction to Behavioral Pattern
Behavioral Patterns are design patterns that identify common communication patterns between objects and realize these patterns. They increase flexibility in carrying out this communication.
Chain of Responsibility Pattern
The Chain of Responsibility Pattern is a behavioral design pattern that lets you pass requests along a chain of handlers. Upon receiving a request, each handler decides either to process the request or to pass it to the next handler in the chain.
Key Points:
- The Chain of Responsibility Pattern decouples sender and receiver of a request.
- It reduces the coupling degree by allowing an object in the chain to pass the request along the chain until an object handles it.
Example of Chain of Responsibility Pattern
Here's a simple code example in Java:
abstract class Handler {
protected Handler successor;
public void setSuccessor(Handler successor) {
this.successor = successor;
}
public abstract void handleRequest();
}
class ConcreteHandler1 extends Handler {
public void handleRequest() {
if (condition) {
// handle request
} else if (successor != null) {
successor.handleRequest();
}
}
}
class Client {
public static void main(String[] args) {
Handler h1 = new ConcreteHandler1();
Handler h2 = new ConcreteHandler2();
h1.setSuccessor(h2);
h1.handleRequest();
}
}
In this example, ConcreteHandler1
and ConcreteHandler2
are linked in a chain. When a request is passed to ConcreteHandler1
, it either handles the request or passes it to the next handler in the chain (ConcreteHandler2
), depending on a condition.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version