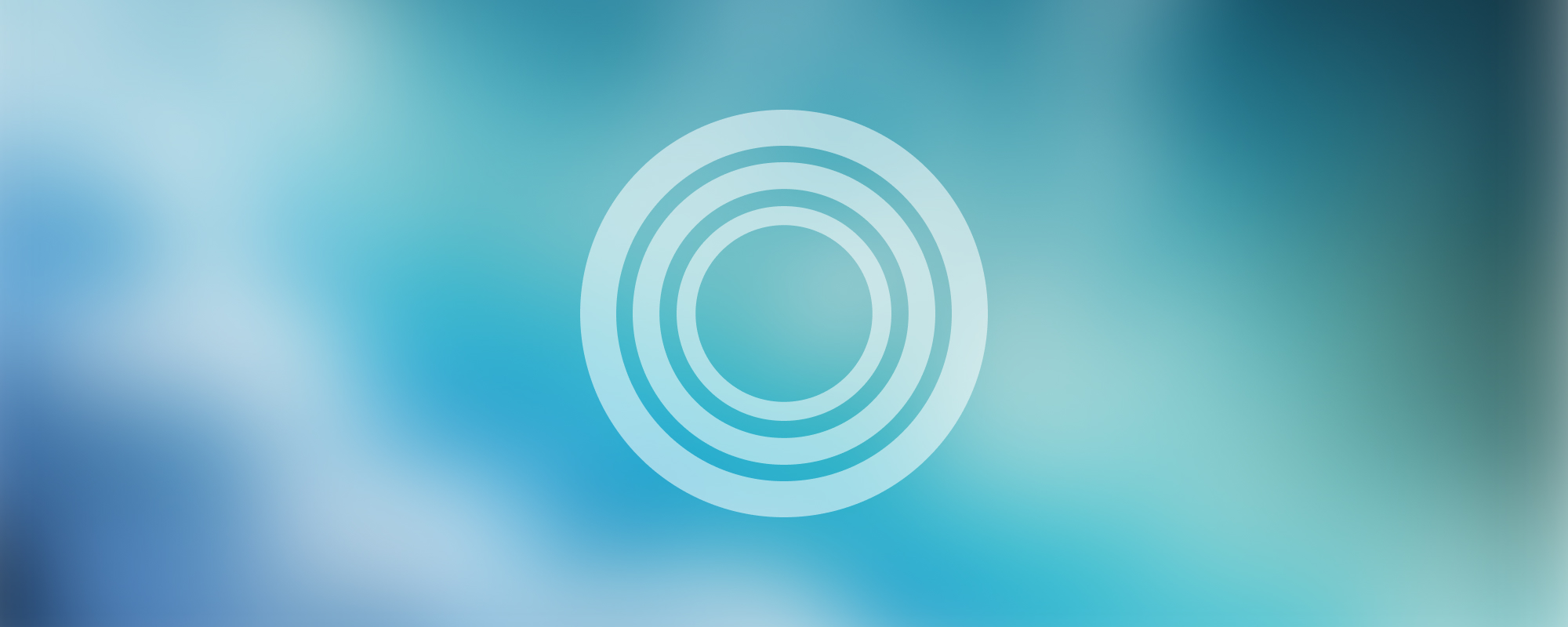
8. State, Iterator, Observer, Template Method Pattern
State Pattern in Software Design
Introduction to State Pattern
The State Pattern is a behavioral design pattern that allows an object to alter its behavior when its internal state changes. This change gives the illusion that the object has changed its class.
Understanding the State Pattern
In a practical example, consider a football game. The team could be in different states such as 'defense' or 'attack'. The behavior of each player changes depending on the state of the entire team.
Example code in Python:
class TeamState:
...
class DefenseState(TeamState):
...
class AttackState(TeamState):
...
This example demonstrates how state changes can alter the behavior of an object (a football team in this case).
Strategy Pattern in Software Design
Introduction to Strategy Pattern
The Strategy Pattern is a behavioral design pattern that allows you to define a family of algorithms, encapsulate each one in a separate class, and make their objects interchangeable.
Understanding the Strategy Pattern
Consider a navigation system that can navigate via car, bus, or on foot. Each mode of transport represents a different algorithm (strategy) for navigation.
Example code in Python:
class NavigationStrategy:
...
class CarStrategy(NavigationStrategy):
...
class BusStrategy(NavigationStrategy):
...
The Strategy Pattern and the State Pattern may seem similar, but they differ in that the State Pattern is about changing states while the Strategy Pattern revolves around changing algorithms (strategies).
Iterator Pattern in Software Design
Introduction to Iterator Pattern
The Iterator Pattern is a behavioral design pattern that allows you to traverse elements of a collection without exposing its underlying representation (like a list, stack, or tree).
Understanding the Iterator Pattern
The Iterator pattern can be used to traverse a collection in different orders. For instance, a depth-first search goes as deep as possible before backtracking, while a breadth-first search goes from left to right, then down, and so on.
Example code in Python:
class Iterator:
...
class DepthFirstIterator(Iterator):
...
class BreadthFirstIterator(Iterator):
...
Observer Pattern in Software Design
Introduction to Observer Pattern
The Observer Pattern is a behavioral design pattern that lets you define a subscription mechanism to notify multiple objects about any events that happen to the object they're observing.
Understanding the Observer Pattern
Think of a publisher and subscriber relationship, like a notification system. When an event happens (e.g., a new post is published), all subscribers are notified.
Example code in Python:
class Publisher:
...
class Subscriber:
...
Template Method Pattern in Software Design
Introduction to Template Method Pattern
The Template Method Pattern is a behavioral design pattern that defines the skeleton of an algorithm in a superclass but lets subclasses override specific steps of the algorithm without changing its structure.
Understanding the Template Method Pattern
Imagine you have a method that is templated. Different versions of this method can be derived from the template, altering the algorithm without changing the overall flow.
Example code in Python:
class SuperClass:
def template_method(self):
...
class SubClass(SuperClass):
def template_method(self):
...
In this example, the template_method
in SubClass
might have a different implementation but it would still follow the same sequence of operations defined in SuperClass
.
Reference
The content in this document is based on the original notes provided in Azerbaijani. For further details, you can refer to the original document using the following link:
Original Note - Azerbaijani Version